


Tips for using Python for script debugging on Linux platform
Tips of using Python for script debugging on the Linux platform
Using Python for script debugging on the Linux platform is one of the common tasks in the development process. Script debugging can help us quickly locate and fix errors in the code and improve development efficiency. This article will introduce some techniques for script debugging using Python on the Linux platform and provide specific code examples.
- Use the pdb module for interactive debugging
Python provides the pdb module, which can insert breakpoints in the code and enter interactive debugging mode during running. The following is a simple example:
import pdb def divide(x, y): result = x / y return result pdb.set_trace() # 插入断点 print(divide(10, 0))
After inserting the pdb.set_trace()
statement in the code, the running code will pause at this location and enter interactive debugging mode. We can use the commands provided by the pdb module for debugging, such as step
to execute code step by step, print
to print variable values, etc. This way you can quickly locate the problem.
- Use the logging module for log debugging
The logging module is Python's built-in logging tool, which can easily insert log statements into the code to help us track the execution process of the code. Here is an example:
import logging logging.basicConfig(level=logging.DEBUG) # 设置日志级别为DEBUG def divide(x, y): logging.debug("start divide function") try: result = x / y except ZeroDivisionError: logging.error("division by zero") return None return result print(divide(10, 0))
By inserting logging.debug()
and logging.error()
statements in the code, we can run the process record relevant information. Use the basicConfig()
function to configure the log, including setting the log level, log output location, etc. Log levels include DEBUG
, INFO
, WARNING
, ERROR
and CRITICAL
. We can set different levels as needed. level.
- Use assertions for code checking
Assertion is a statement in Python that is used to check the code. If the condition of the assertion is not met, the program will interrupt and throw anAssertionError
exception. Here is an example:
def divide(x, y): assert y != 0, "division by zero" result = x / y return result print(divide(10, 0))
In the above example, we use the assert
statement to check if y
is 0 and throw an exception if it is 0, and output an error message. By using assertions, we can pre-check various conditions in the code and reduce the occurrence of errors.
In addition to the above tips, there are some other debugging tools that can help us debug Python scripts on the Linux platform, such as using IDE integrated debuggers, using third-party tools such as pdb, etc. Choosing a debugging method that suits you can improve development efficiency and reduce debugging time.
To summarize, using Python for script debugging on the Linux platform requires mastering the use of the pdb module, the configuration of the logging module, and the use of assertions. By using these techniques appropriately, we can locate and fix errors in the code more quickly and improve development efficiency.
(564 words in total)
The above is the detailed content of Tips for using Python for script debugging on Linux platform. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


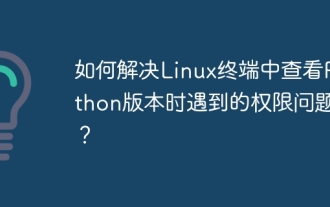
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
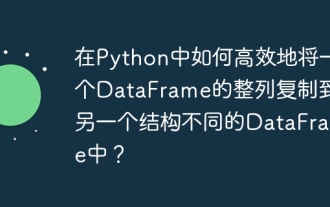
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
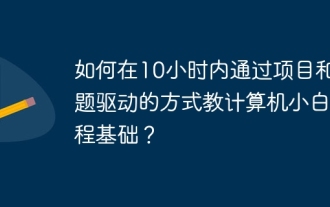
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
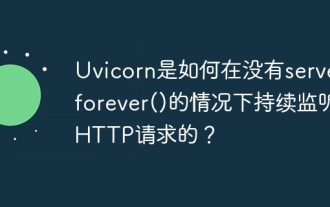
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
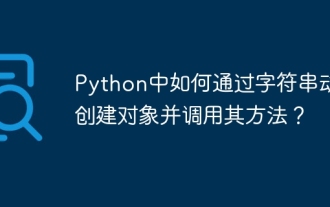
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
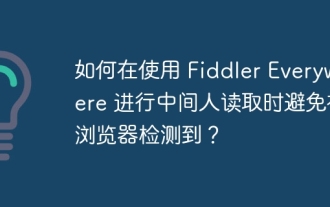
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
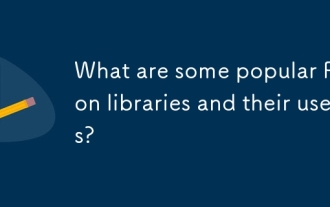
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
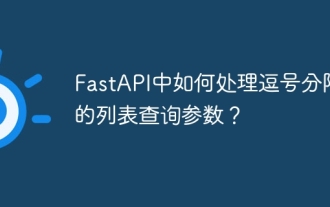
Fastapi ...
