


How to use php functions to optimize form processing and submission functionality?
How to use PHP functions to optimize form processing and submission functions?
As web applications develop, form processing and submission are a very important part of development. In PHP, using appropriate functions for processing and submitting forms can improve the readability and maintainability of your code. This article will introduce some commonly used PHP functions and their application in form processing and submission.
-
htmlspecialchars() function
When users submit form data, in order to prevent XSS attacks, special characters usually need to be converted into HTML entities. The htmlspecialchars() function can achieve this function.// 原始表单数据 $data = $_POST['input']; // 转换为HTML实体 $data = htmlspecialchars($data);
Copy after login trim() function
Before the form is submitted, it is usually necessary to remove the leading and trailing spaces in the user input. The trim() function can achieve this function.// 原始表单数据 $data = $_POST['input']; // 移除首尾空格 $data = trim($data);
Copy after loginfilter_var() function
Before the form is submitted, the data entered by the user needs to be verified and filtered. The filter_var() function can verify and filter data according to the specified filter.// 原始表单数据 $data = $_POST['input']; // 验证和过滤数据 $data = filter_var($data, FILTER_SANITIZE_STRING); // 过滤HTML标签和特殊字符 $data = filter_var($data, FILTER_VALIDATE_EMAIL); // 验证是否为有效的电子邮件地址
Copy after loginisset() function
Before the form is submitted, it is usually necessary to check whether all required fields have been filled in. The isset() function can check whether the variable is set and not null.// 检查必填字段是否存在 if(isset($_POST['input1']) && isset($_POST['input2'])) { // 执行表单提交操作 } else { // 显示错误消息 }
Copy after loginfile_exists() function
When the form is submitted, if you want to upload a file, you usually need to check whether the file already exists. The file_exists() function can check whether the file exists.// 获取上传文件路径 $file = $_FILES['input']['tmp_name']; // 检查文件是否存在 if(file_exists($file)) { // 执行文件上传操作 } else { // 显示错误消息 }
Copy after loginmove_uploaded_file() function
When uploading a file, you usually need to move the file to the specified directory. The move_uploaded_file() function can achieve this function.// 获取上传文件路径 $file = $_FILES['input']['tmp_name']; // 移动文件到指定目录 $destination = 'uploads/' . $_FILES['input']['name']; if(move_uploaded_file($file, $destination)) { // 文件移动成功 } else { // 文件移动失败 }
Copy after loginpassword_hash() function
When a user registers, it is usually necessary to hash the password. The password_hash() function can generate the hash value of the password.// 获取用户输入的密码 $password = $_POST['input']; // 生成密码的哈希值 $hashedPassword = password_hash($password, PASSWORD_DEFAULT);
Copy after loginpassword_verify() function
When a user logs in, it is usually necessary to verify whether the password matches the stored hash value. The password_verify() function can verify passwords.// 获取用户输入的密码 $password = $_POST['input']; // 获取存储的哈希值 $hashedPassword = '...'; // 验证密码 if(password_verify($password, $hashedPassword)) { // 密码匹配 } else { // 密码不匹配 }
Copy after login
By using these PHP functions, we can process and submit form data more efficiently. At the same time, these functions also improve the security and scalability of the code. In actual development, according to specific needs, different functions can be used as needed to optimize the form processing and submission functions.
The above is the detailed content of How to use php functions to optimize form processing and submission functionality?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


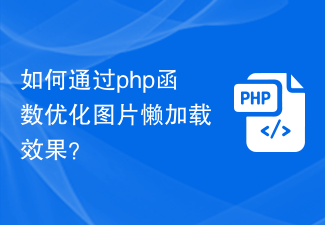
How to optimize the lazy loading effect of images through PHP functions? With the development of the Internet, the number of images in web pages is increasing, which puts pressure on page loading speed. In order to improve user experience and reduce loading time, we can use image lazy loading technology. Lazy loading of images can delay the loading of images. Images are only loaded when the user scrolls to the visible area, which can reduce the loading time of the page and improve the user experience. When writing PHP web pages, we can optimize the lazy loading effect of images by writing some functions. Details below
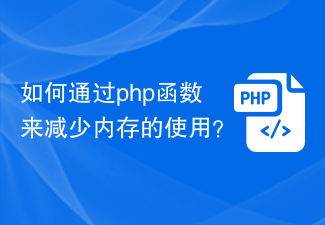
How to reduce memory usage through PHP functions. In development, memory usage is a very important consideration. If a large amount of memory is used in a program, it may cause slowdowns or even program crashes. Therefore, reasonably managing and reducing memory usage is an issue that every PHP developer should pay attention to. This article will introduce some methods to reduce memory usage through PHP functions, and provide specific code examples for readers' reference. Use the unset() function to release variables in PHP. When a variable is no longer needed, use
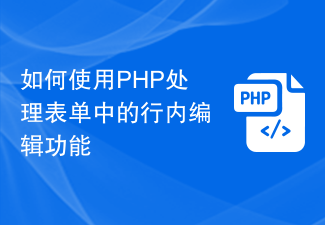
How to use PHP to handle inline editing functions in forms Introduction: Forms are one of the commonly used elements in web development and are used to collect data entered by users. The inline editing function allows users to instantly edit and save data directly within the form, improving user experience and operational efficiency. This article will introduce how to use PHP to handle inline editing functions in forms, and attach corresponding code examples. 1. HTML part First, we need to create a form that contains inline editing functionality. In HTML, we can use content
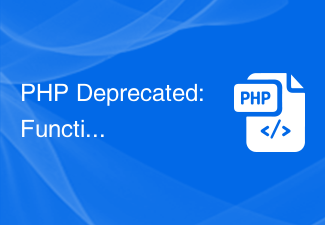
PHPDeprecated: Functionereg_replace()isdeprecated-Solution When developing in PHP, we often encounter the problem of some functions being declared deprecated. This means that in the latest PHP versions, these functions may be removed or replaced. One common example is the ereg_replace() function. ereg_replace
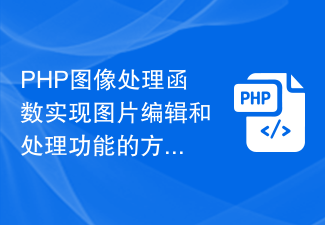
PHP image processing functions are a set of functions specifically used to process and edit images. They provide developers with rich image processing functions. Through these functions, developers can implement operations such as cropping, scaling, rotating, and adding watermarks to images to meet different image processing needs. First, I will introduce how to use PHP image processing functions to achieve image cropping function. PHP provides the imagecrop() function, which can be used to crop images. By passing the coordinates and size of the cropping area, we can crop the image

PHP function introduction: strtr() function In PHP programming, the strtr() function is a very useful string replacement function. It is used to replace specified characters or strings in a string with other characters or strings. This article will introduce the usage of strtr() function and give some specific code examples. The basic syntax of the strtr() function is as follows: strtr(string$str, array$replace) where $str is the original word to be replaced.
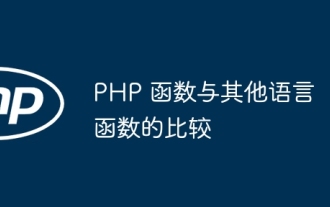
PHP functions have similarities with functions in other languages, but also have some unique features. Syntactically, PHP functions are declared with function, JavaScript is declared with function, and Python is declared with def. In terms of parameters and return values, PHP functions accept parameters and return a value. JavaScript and Python also have similar functions, but the syntax is different. In terms of scope, functions in PHP, JavaScript and Python all have global or local scope. Global functions can be accessed from anywhere, and local functions can only be accessed within their declaration scope.
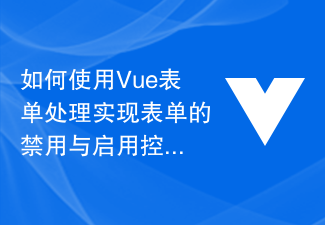
How to use Vue form processing to implement disabling and enabling control of forms. In web development, forms are one of the indispensable components. Sometimes, we need to control the disabled and enabled status of a form based on specific conditions. Vue provides a concise and effective way to handle this situation. This article will introduce in detail how to use Vue to implement disabling and enabling control of the form. First, we need to create a basic Vue instance and a form. Here is a basic HTML and Vue code example: <divid=&
