Java and Linux Script Operations: How to Achieve Efficient File Management
Java and Linux script operations: How to achieve efficient file management
In software development and system management, file management is a very important task. Whether we are dealing with file input and output in development, or file backup and migration in system management, we all need an efficient way to manage and operate files. This article will introduce how to use Java and Linux scripts to achieve efficient file management and provide specific code examples.
1. Java file operations
Java is a powerful programming language that provides a wealth of classes and methods to process files. The following are some common Java file operation examples:
- Creating Folders
Using Java's File class, we can easily create folders:
File folder = new File("path/to/folder"); boolean success = folder.mkdirs(); if (success) { System.out.println("文件夹创建成功"); } else { System.out.println("文件夹创建失败"); }
- Copy files
Java's file copy mainly relies on the reading and writing of byte streams. The following is a simple file copy example:
File sourceFile = new File("path/to/source/file"); File destFile = new File("path/to/destination/file"); try ( FileInputStream fis = new FileInputStream(sourceFile); FileOutputStream fos = new FileOutputStream(destFile) ) { byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = fis.read(buffer)) > 0) { fos.write(buffer, 0, bytesRead); } System.out.println("文件复制成功"); } catch (IOException e) { e.printStackTrace(); System.out.println("文件复制失败"); }
- Deleting files
Use the delete() method of Java's File class to delete files:
File file = new File("path/to/file"); boolean deleted = file.delete(); if (deleted) { System.out.println("文件删除成功"); } else { System.out.println("文件删除失败"); }
The above are just simple examples of Java file operations. Java also provides more file operation methods, such as renaming, traversing folders, etc., which can be selected and used according to specific needs.
2. Linux script file operation
Linux is one of the most commonly used operating systems and provides a wealth of command line tools for file management. The following are some common examples of Linux script file operations:
- Create Folder
Using the mkdir command can easily create a folder in Linux:
mkdir path/to/folder
- Copy files
Linux provides the cp command to copy files:
cp path/to/source/file path/to/destination/file
- Delete files
Use rm Commands can delete files:
rm path/to/file
The above are just simple examples of file operations in Linux scripts. Linux also provides more file operation commands, such as the mv command for renaming and moving files, and the find command for Find files and more. Can be selected and used according to specific needs.
3. Combined application of Java and Linux scripts
Java and Linux scripts can be well combined to provide more efficient file management. The following is an example that demonstrates how to use Java's file operations and Linux scripts to implement batch file backup:
import java.io.File; import java.io.IOException; public class FileBackup { public static void main(String[] args) { String sourceFolder = "path/to/source/folder"; String destFolder = "path/to/destination/folder"; // 创建备份文件夹 File destDir = new File(destFolder); destDir.mkdirs(); // 获取源文件夹下的所有文件 File sourceDir = new File(sourceFolder); File[] files = sourceDir.listFiles(); // 使用Linux脚本进行文件复制 for (File file : files) { String filename = file.getName(); String command = "cp " + sourceFolder + "/" + filename + " " + destFolder + "/" + filename; try { Process process = Runtime.getRuntime().exec(command); process.waitFor(); System.out.println(filename + " 备份成功"); } catch (IOException | InterruptedException e) { e.printStackTrace(); System.out.println(filename + " 备份失败"); } } } }
The above example demonstrates how to create a backup folder through Java and use Linux scripts for file copying. It can be modified and extended according to specific needs, such as selective backup of files by adding file filters.
Summary:
This article introduces how to use Java and Linux scripts to achieve efficient file management. Through Java file operations and Linux command line tools, we can easily create folders, copy files, and delete files. The combined application of Java and Linux scripts can provide more flexible and powerful file management capabilities. I hope this article is helpful to your file management efforts.
The above is the detailed content of Java and Linux Script Operations: How to Achieve Efficient File Management. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Windows Subsystem for Linux The first option is to use Windows Subsystem for Linux or WSL, which is a compatibility layer for running Linux binary executables natively on Windows systems. It works for most scenarios and allows you to run shell scripts in Windows 11/10. WSL is not automatically available, so you must enable it through your Windows device's developer settings. You can do this by going to Settings > Update & Security > For Developers. Switch to developer mode and confirm the prompt by selecting Yes. Next, look for W
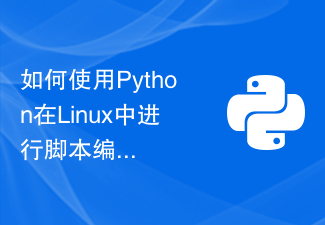
How to use Python to write and execute scripts in Linux In the Linux operating system, we can use Python to write and execute various scripts. Python is a concise and powerful programming language that provides a wealth of libraries and tools to make scripting easier and more efficient. Below we will introduce the basic steps of how to use Python for script writing and execution in Linux, and provide some specific code examples to help you better understand and use it. Install Python
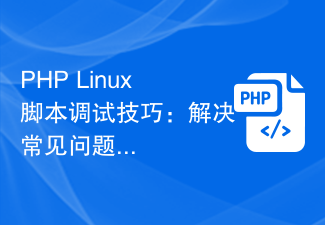
PHPLinux script debugging skills: methods to solve common problems, specific code examples are required Introduction: When developing and maintaining PHP scripts, we often encounter various problems. Debugging is one of the key steps in resolving these issues. This article will introduce some common problems and solutions for debugging PHP scripts in a Linux environment, and provide specific code examples. 1. Use echo and var_dump to output variable values. When debugging PHP scripts, we often need to view the values of variables to determine the execution of the code.
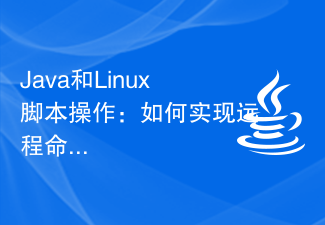
Java and Linux Script Operation: Implementing Remote Command Execution Overview: In actual application development, we often encounter the need to execute commands on a remote server. This article will introduce how to implement remote command execution through Java and Linux scripts, and provide specific code examples. Java implements remote command execution In Java, you can use the JSch library to implement remote command execution. JSch is an SSH2 protocol library implemented in pure Java, which can be used to establish SSH connections, execute commands, and transfer files.
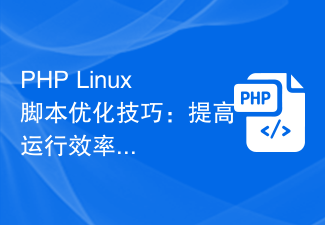
PHPLinux script optimization tips: Overview of methods to improve operating efficiency: When developing and deploying PHP applications, how to optimize the performance of scripts is an important issue. Especially on Linux operating systems, there are many optimization techniques that can improve the efficiency of scripts. This article will introduce some commonly used PHPLinux script optimization techniques and provide specific code examples. Using Buffered Output When a PHP script outputs a large amount of data, you can use buffered output to reduce the number of I/O operations and thereby improve performance. exist

Java and Linux script operations: How to achieve efficient file management In software development and system management, file management is a very important task. Whether we are dealing with file input and output in development, or file backup and migration in system management, we all need an efficient way to manage and operate files. This article will introduce how to use Java and Linux scripts to achieve efficient file management and provide specific code examples. 1. Java file operations Java is a powerful programming language that provides
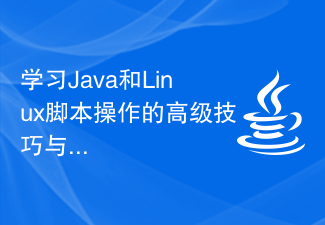
Learning advanced techniques and techniques for Java and Linux script operations requires specific code examples. In the contemporary field of software development, mastering advanced techniques and techniques for Java and Linux script operations is very critical. Whether in enterprise-level application development, or in system management and automated scripting, the flexible use of these skills can greatly improve development efficiency and application performance. Java is a widely used object-oriented programming language. Its powerful features and rich libraries make it one of the preferred languages for many developers. Down
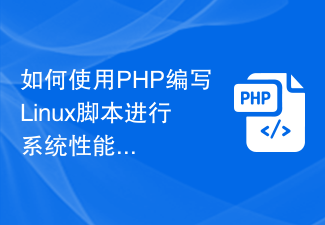
How to use PHP to write Linux scripts for system performance monitoring In Linux systems, system performance monitoring is very important for administrators and developers. By monitoring the performance of the system, we can understand the load of the system, discover potential problems in time, and take corresponding measures to ensure the stable operation of the system. This article will introduce how to use PHP to write Linux scripts for system performance monitoring and provide specific code examples. 1. Preparation First, make sure that PHP is installed on your Linux system. you can
