


How to handle interface concurrent requests and concurrent processing in PHP development
How to handle interface concurrent requests and concurrent processing in PHP development
In actual Web development, we often encounter concurrent requests. Concurrent requests refer to multiple requests being sent to the server for processing at the same time. If our application cannot handle concurrent requests correctly, it may lead to data inconsistency, performance degradation and other problems. This article will introduce how to handle concurrent requests and concurrent processing of interfaces in PHP development, and provide specific code examples.
1. The problem of concurrent requests
In traditional Web development, each request is processed in sequence. However, with the development of the Internet, the increase in the number of users, and the increase in user needs, we need to handle a large number of concurrent requests more efficiently. If we simply submit concurrent requests to the server, the following problems may occur:
- Data inconsistency: If multiple requests read or modify the same data at the same time, it may cause data inconsistency. . For example, if two requests read a certain value in the database at the same time and operate based on that value, one of the requests may operate based on an expired value.
- Performance degradation: If the server does not handle concurrent requests correctly, but instead processes each request in turn, the waiting time for the request will increase, thereby reducing the overall performance.
In order to solve the problem of concurrent requests, we need to introduce some mechanisms to ensure data consistency and improve performance.
2. Solution for handling concurrent requests
- Transaction processing: In database operations, transactions can be used to ensure data consistency. Transactions provide ACID (atomicity, consistency, isolation, and durability) characteristics and can commit or rollback a set of related database operations as a whole. By placing concurrent requests within a transaction, data consistency is ensured.
In PHP, you can use PDO to perform database operations, and use methods such as beginTransaction, commit, and rollback to implement transaction processing. The following is a simple sample code:
try { $pdo = new PDO('mysql:host=localhost;dbname=test', 'username', 'password'); $pdo->beginTransaction(); // 执行数据库操作 $pdo->commit(); } catch (PDOException $e) { $pdo->rollback(); echo "Error: " . $e->getMessage(); }
- Lock mechanism: In addition to database transactions, we can also use locks to handle concurrent requests. A lock is a synchronization mechanism that prevents multiple processes from accessing a resource at the same time. In PHP, you can use file locks, database locks, etc. to achieve this.
The following is a sample code that uses file locks to handle concurrent requests:
$fp = fopen("lock.txt", "w+"); if (flock($fp, LOCK_EX)) { // 执行操作 flock($fp, LOCK_UN); // 释放锁 } else { echo "无法获得锁"; } fclose($fp);
- Queue processing: Queue is a commonly used concurrent processing mechanism. into the queue, and then process the requests in the queue in sequence, which can avoid the impact of concurrent requests on the system.
In PHP, you can use message queue, Redis queue, etc. to implement queue processing of concurrent requests.
The following is a sample code that uses Redis queue to process concurrent requests:
$redis = new Redis(); $redis->connect('127.0.0.1', 6379); $redis->lpush('queue', 'request1'); $redis->lpush('queue', 'request2'); while ($request = $redis->lpop('queue')) { // 处理请求 }
Through the above processing mechanism, we can effectively handle concurrent requests, ensure data consistency and improve performance.
Summary
In PHP development, handling concurrent requests for interfaces is a common problem. In order to avoid problems such as data inconsistency and performance degradation, we can use transaction processing, locking mechanisms, queue processing, etc. to handle concurrent requests. This article gives specific code examples, I hope it will be helpful to everyone. Of course, depending on specific business scenarios and needs, other solutions may be needed to handle concurrent requests.
The above is the detailed content of How to handle interface concurrent requests and concurrent processing in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


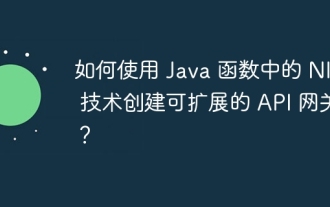
Answer: Using NIO technology you can create a scalable API gateway in Java functions to handle a large number of concurrent requests. Steps: Create NIOChannel, register event handler, accept connection, register data, read and write handler, process request, send response
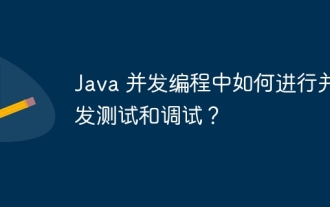
Concurrency testing and debugging Concurrency testing and debugging in Java concurrent programming are crucial and the following techniques are available: Concurrency testing: Unit testing: Isolate and test a single concurrent task. Integration testing: testing the interaction between multiple concurrent tasks. Load testing: Evaluate an application's performance and scalability under heavy load. Concurrency Debugging: Breakpoints: Pause thread execution and inspect variables or execute code. Logging: Record thread events and status. Stack trace: Identify the source of the exception. Visualization tools: Monitor thread activity and resource usage.
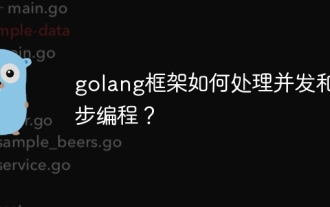
The Go framework uses Go's concurrency and asynchronous features to provide a mechanism for efficiently handling concurrent and asynchronous tasks: 1. Concurrency is achieved through Goroutine, allowing multiple tasks to be executed at the same time; 2. Asynchronous programming is implemented through channels, which can be executed without blocking the main thread. Task; 3. Suitable for practical scenarios, such as concurrent processing of HTTP requests, asynchronous acquisition of database data, etc.
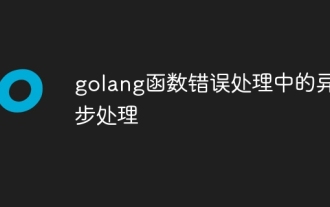
In Go functions, asynchronous error handling uses error channels to asynchronously pass errors from goroutines. The specific steps are as follows: Create an error channel. Start a goroutine to perform operations and send errors asynchronously. Use a select statement to receive errors from the channel. Handle errors asynchronously, such as printing or logging error messages. This approach improves the performance and scalability of concurrent code because error handling does not block the calling thread and execution can be canceled.
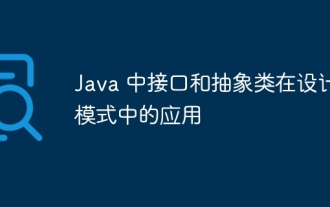
Interfaces and abstract classes are used in design patterns for decoupling and extensibility. Interfaces define method signatures, abstract classes provide partial implementation, and subclasses must implement unimplemented methods. In the strategy pattern, the interface is used to define the algorithm, and the abstract class or concrete class provides the implementation, allowing dynamic switching of algorithms. In the observer pattern, interfaces are used to define observer behavior, and abstract or concrete classes are used to subscribe and publish notifications. In the adapter pattern, interfaces are used to adapt existing classes. Abstract classes or concrete classes can implement compatible interfaces, allowing interaction with original code.
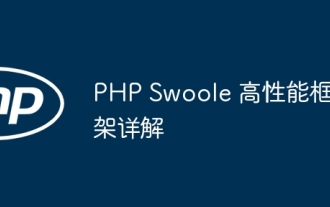
Swoole is a concurrency framework based on PHP coroutines, which has the advantages of high concurrency processing capabilities, low resource consumption, and simplified code development. Its main features include: coroutine concurrency, event-driven networks and concurrent data structures. By using the Swoole framework, developers can greatly improve the performance and throughput of web applications to meet the needs of high-concurrency scenarios.
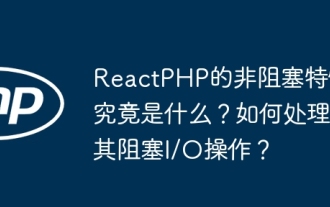
An official introduction to the non-blocking feature of ReactPHP in-depth interpretation of ReactPHP's non-blocking feature has aroused many developers' questions: "ReactPHPisnon-blockingbydefault...

Java allows inner classes to be defined within interfaces and abstract classes, providing flexibility for code reuse and modularization. Inner classes in interfaces can implement specific functions, while inner classes in abstract classes can define general functions, and subclasses provide concrete implementations.
