Common performance tuning methods and techniques in Java development
Common performance tuning methods and techniques in Java development
Abstract: In Java development, performance tuning is an important topic. Good performance can improve the stability and user experience of the software system. This article will introduce some common performance tuning methods and techniques and provide specific code examples.
- Choose appropriate data structures and algorithms
When writing code, choosing appropriate data structures and algorithms is the key to improving performance. For example, in situations where frequent insertion and deletion of elements is required, using a linked list may be more efficient than using an array. In situations where fast searching and sorting is required, using a binary search tree or a red-black tree may be faster than using an ordinary array. In addition, reasonable use of cache and index can greatly improve the efficiency of the program.
Sample code:
LinkedList
linkedList.add(1); // Insert elements
linkedList.remove(0); // Delete elements
- Avoid frequent object creation and destruction
The garbage collection mechanism in Java will automatically recycle objects that are frequently created and destroyed, but this The process will bring certain performance overhead. Therefore, in scenarios with high performance requirements, we can avoid frequent object creation and destruction through object pools or caches, thereby improving performance.
Sample code:
//Use object pool to manage the creation and destruction of objects
ObjectPool pool = new ObjectPool();
Object obj = pool.getObject(); // Get the object from the object pool
// After using the object, put the object back into the object pool
pool.releaseObject(obj);
- Use multi-threading appropriately
Multithreading is a common way to improve program performance. Reasonable use of multi-threading can make full use of system resources and improve the concurrent execution capability of code. However, too many threads may cause frequent switching between threads, thereby reducing performance. Therefore, when using multi-threading, you need to reasonably adjust the number of threads according to the actual situation, and pay attention to synchronization and mutual exclusion between threads to avoid thread safety issues.
Sample code:
// Use thread pool to manage threads
ExecutorService executorService = Executors.newFixedThreadPool(5); // Create a fixed size thread pool
Runnable task = new MyTask(); // Define a task
executorService.execute(task); // Submit the task to the thread pool for execution
- Reduce IO operations
IO operations are usually time-consuming Operations, in scenarios with high performance requirements, it is necessary to minimize the number of IO operations. For example, caching can be used to avoid frequent IO operations when reading and writing files or network communications. In addition, when performing a large number of IO operations, using NIO (non-blocking IO) method can improve the efficiency of IO.
Sample code:
// Use caching to reduce the number of IO operations
InputStream input = new BufferedInputStream(new FileInputStream("file.txt"));
OutputStream output = new BufferedOutputStream(new FileOutputStream("copy.txt"));
byte[] buffer = new byte[1024];
int length;
while ((length = input.read(buffer)) ! = -1) {
output.write(buffer, 0, length);
}
- Performance testing and monitoring
Performance tuning is not only done when writing code, but also requires performance testing and monitoring. In order to promptly discover performance bottlenecks and optimize them. You can use some performance testing tools to evaluate the performance of the code, such as JMH, Apache JMeter, etc. At the same time, you can also use some performance monitoring tools to monitor the running status of the code, such as JConsole, VisualVM, etc.
Summary:
This article introduces common performance tuning methods and techniques in Java development, and provides specific code examples. I hope that by studying this article, readers can better understand and master how to perform Java performance tuning, thereby improving their programming abilities. In actual development, we should choose appropriate tuning methods based on specific needs, combined with code writing and performance testing, to improve program performance.
The above is the detailed content of Common performance tuning methods and techniques in Java development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


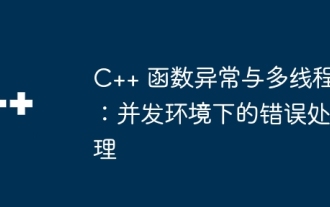
Function exception handling in C++ is particularly important for multi-threaded environments to ensure thread safety and data integrity. The try-catch statement allows you to catch and handle specific types of exceptions when they occur to prevent program crashes or data corruption.
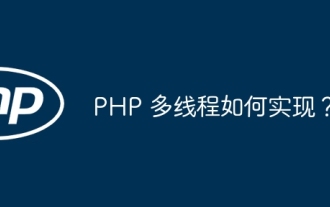
PHP multithreading refers to running multiple tasks simultaneously in one process, which is achieved by creating independently running threads. You can use the Pthreads extension in PHP to simulate multi-threading behavior. After installation, you can use the Thread class to create and start threads. For example, when processing a large amount of data, the data can be divided into multiple blocks and a corresponding number of threads can be created for simultaneous processing to improve efficiency.
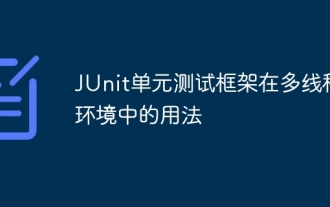
There are two common approaches when using JUnit in a multi-threaded environment: single-threaded testing and multi-threaded testing. Single-threaded tests run on the main thread to avoid concurrency issues, while multi-threaded tests run on worker threads and require a synchronized testing approach to ensure shared resources are not disturbed. Common use cases include testing multi-thread-safe methods, such as using ConcurrentHashMap to store key-value pairs, and concurrent threads to operate on the key-value pairs and verify their correctness, reflecting the application of JUnit in a multi-threaded environment.
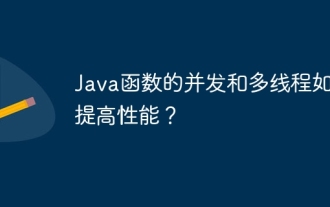
Concurrency and multithreading techniques using Java functions can improve application performance, including the following steps: Understand concurrency and multithreading concepts. Leverage Java's concurrency and multi-threading libraries such as ExecutorService and Callable. Practice cases such as multi-threaded matrix multiplication to greatly shorten execution time. Enjoy the advantages of increased application response speed and optimized processing efficiency brought by concurrency and multi-threading.
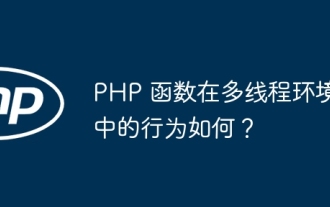
In a multi-threaded environment, the behavior of PHP functions depends on their type: Normal functions: thread-safe, can be executed concurrently. Functions that modify global variables: unsafe, need to use synchronization mechanism. File operation function: unsafe, need to use synchronization mechanism to coordinate access. Database operation function: Unsafe, database system mechanism needs to be used to prevent conflicts.
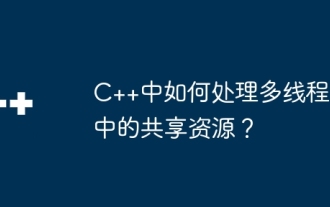
Mutexes are used in C++ to handle multi-threaded shared resources: create mutexes through std::mutex. Use mtx.lock() to obtain a mutex and provide exclusive access to shared resources. Use mtx.unlock() to release the mutex.
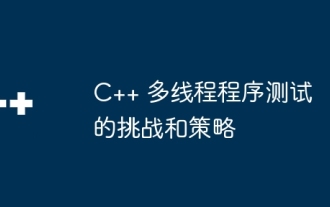
Multi-threaded program testing faces challenges such as non-repeatability, concurrency errors, deadlocks, and lack of visibility. Strategies include: Unit testing: Write unit tests for each thread to verify thread behavior. Multi-threaded simulation: Use a simulation framework to test your program with control over thread scheduling. Data race detection: Use tools to find potential data races, such as valgrind. Debugging: Use a debugger (such as gdb) to examine the runtime program status and find the source of the data race.
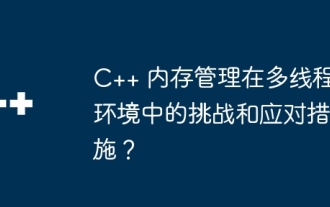
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.
