


How to optimize logging and performance monitoring in PHP development
How to optimize logging and performance monitoring in PHP development
In the PHP development process, logging and performance monitoring are very important tasks. Recording logs and monitoring performance reasonably and effectively can help us quickly locate and solve problems and improve system stability and performance. This article will introduce some methods to optimize logging and performance monitoring, and provide specific code examples.
1. Optimize logging
- Use appropriate log levels
In PHP, we can use different log levels to record different severities event. Choosing the appropriate log level based on actual needs can avoid excessive useless log records and reduce the impact on system performance. The following are several common log levels:
- DEBUG: debugging information, recording detailed debugging logs, generally only used during the development process.
- INFO: General information, records some important running information, such as user operation logs.
- WARNING: Warning information, recording some problems that do not affect the normal operation of the system but require attention.
- ERROR: Error information, recording errors and exceptions in the system, which need to be processed in a timely manner.
- CRITICAL: Critical error message, recording serious system errors and crashes.
Using appropriate log levels can ensure the accuracy of logging and avoid generating too many useless log files.
- Reasonable selection of log storage methods
In PHP, we can choose different storage methods to save log information, such as files, databases, message queues, etc. Choosing an appropriate storage method based on the actual situation can improve the efficiency and scalability of logging. The following are several common log storage methods:
- File storage: Writing log information to files makes it easy to view and analyze logs. Use file locking or cutting strategies to avoid problems with large log files or concurrent writes.
- Database storage: Save log information to the database for easy query and statistical analysis. Using technologies such as indexing and sharding can improve query efficiency and system performance.
- Message queue storage: Send log information to the message queue, which can process log information asynchronously and reduce the impact on system performance. Message queues can be used to achieve batch processing and reliable transmission of logs.
Choose an appropriate log storage method based on project requirements and actual conditions, which can not only meet the needs of logging, but also improve system performance and scalability.
- Use caching technology
During the logging process, frequent disk IO operations may affect system performance. In order to reduce the number of disk IOs, caching technology can be used to temporarily store log information in memory, and then write it to disk regularly or asynchronously. This can greatly reduce disk access and improve system performance.
The following is a logging example using caching technology:
<?php class Logger { private $logCache = []; public function log($level, $message) { $logCache[] = ['level' => $level, 'message' => $message]; } public function flush() { foreach ($logCache as $log) { // 将日志信息写入磁盘 file_put_contents('log.txt', $log['message'], FILE_APPEND); } $logCache = []; } } // 使用示例 $logger = new Logger(); $logger->log('INFO', 'This is a test log message'); $logger->flush(); ?>
In the above example, the log method in the Logger class adds log information to the $logCache array, and the flush method periodically adds $logCache The log information in the array is written to disk. By using caching technology, you can reduce the number of disk accesses and improve logging efficiency and system performance.
2. Performance monitoring
- Use performance analysis tools
During the PHP development process, we can use performance analysis tools to monitor the performance of the system. Performance analysis tools can help us identify performance bottlenecks and potential problems in the system, so as to carry out targeted optimization. The following are several common performance analysis tools:
- Xdebug: Xdebug is a debugging and analysis extension for PHP that can provide real-time performance data (such as function calls, memory usage, etc.) and call graphs.
- Blackfire: Blackfire is a powerful PHP performance analysis tool that can deeply analyze performance issues in applications.
- New Relic: New Relic is an application performance monitoring tool that can monitor application performance indicators (such as response time, database queries, etc.) in real time.
By using performance analysis tools, you can fully understand the operation of the system, identify performance bottlenecks and potential problems, and perform corresponding performance optimization.
- Using logging to record time consumption
In addition to using performance analysis tools, we can also monitor system performance by recording time consumption information in the code. By recording the execution time of important functions or processes, we can understand which parts of the system take a long time and make targeted optimizations.
The following is an example of recording function execution time:
<?php function testFunction() { // 记录函数开始时间 $start = microtime(true); // 函数主体代码 // ... // 计算函数执行时间 $executionTime = microtime(true) - $start; // 将执行时间写入日志 file_put_contents('log.txt', 'Function execution time: ' . $executionTime . ' seconds', FILE_APPEND); } // 使用示例 testFunction(); ?>
In the above example, we use the microtime function to record the function start time and end time respectively, and calculate the execution time. The execution time is then written to the log file. In this way, we can understand which functions in the system take a long time to execute and optimize them.
Summarize
Optimizing logging and performance monitoring are very important tasks in PHP development. By choosing appropriate log levels and storage methods, using caching technology and performance analysis tools, and reasonably recording time-consuming information, we can improve system stability and performance. I hope the methods and examples provided in this article are helpful to you.
The above is the detailed content of How to optimize logging and performance monitoring in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
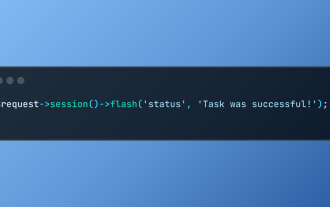
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
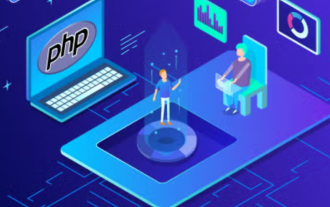
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
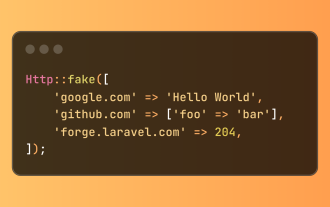
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
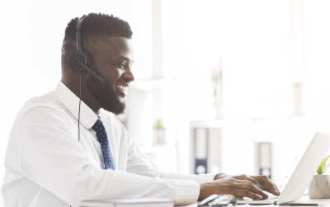
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
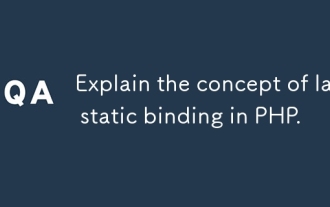
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
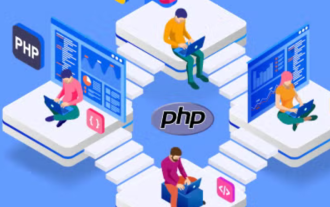
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
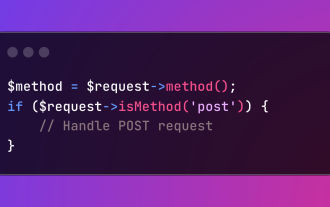
Laravel simplifies HTTP verb handling in incoming requests, streamlining diverse operation management within your applications. The method() and isMethod() methods efficiently identify and validate request types. This feature is crucial for building
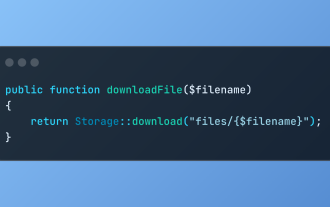
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
