


How to solve high-concurrency task scheduling problems in PHP development
How to solve the high-concurrency task scheduling problem in PHP development
Overview:
In the PHP development process, high-concurrency task scheduling is a common challenge. Especially in large websites or applications, task scheduling may involve issues such as simultaneous execution, competition for resources, and performance. This article will introduce some methods to solve the problem of high concurrent task scheduling and provide specific code examples.
- Use message queue:
Message queue is an efficient task scheduling method, which can process tasks asynchronously and achieve concurrent execution of tasks. In PHP, you can use third-party libraries such as Redis to implement message queues. The sample code is as follows:
// 生产者,将任务添加到消息队列中 $redis = new Redis(); $redis->pconnect('localhost', 6379); $task = array( 'task_id' => 1, 'task_data' => 'This is a task', ); $redis->lpush('task_queue', json_encode($task)); // 消费者,从消息队列中获取任务并执行 while (true) { $redis = new Redis(); $redis->pconnect('localhost', 6379); $taskStr = $redis->rpop('task_queue'); if ($taskStr) { $task = json_decode($taskStr, true); // 执行任务 $result = executeTask($task); } usleep(1000); // 休眠一毫秒,避免空转浪费CPU资源 } function executeTask($task) { // 执行具体任务逻辑 // ... }
- Use process pool:
The process pool is a pre-generated batch of processes. And a method to obtain idle processes from the process pool to perform tasks when needed. In PHP, you can use the pcntl extension to implement a process pool. The sample code is as follows:
$workerNum = 10; // 定义进程池大小 $workers = array(); // 进程数组 // 创建子进程 for ($i = 0; $i < $workerNum; $i++) { $pid = pcntl_fork(); if ($pid < 0) { exit("Fork failed"); } elseif ($pid === 0) { // 子进程执行任务 while (true) { // 从消息队列中获取任务并执行 $redis = new Redis(); $redis->pconnect('localhost', 6379); $taskStr = $redis->rpop('task_queue'); if ($taskStr) { $task = json_decode($taskStr, true); // 执行任务 $result = executeTask($task); } usleep(1000); // 休眠一毫秒,避免空转浪费CPU资源 } exit(0); } else { $workers[] = $pid; } } // 主进程等待所有子进程退出 foreach ($workers as $pid) { pcntl_waitpid($pid, $status); } function executeTask($task) { // 执行具体任务逻辑 // ... }
- Use distributed task scheduling:
When the task volume is extremely large and cannot be processed by a single machine, Distributed task scheduling can be used to achieve efficient scheduling and execution of tasks. Distributed task scheduling frameworks such as Beanstalkd can be used in PHP. The sample code is as follows:
// 生产者,将任务添加到Beanstalkd队列中 $pheanstalk = new Pheanstalk('127.0.0.1'); $taskData = array( 'task_id' => 1, 'task_data' => 'This is a task', ); $pheanstalk->useTube('task_queue')->put(json_encode($taskData)); // 消费者,从Beanstalkd队列中获取任务并执行 $pheanstalk = new Pheanstalk('127.0.0.1'); while (true) { $job = $pheanstalk->reserve(); if ($job) { $task = json_decode($job->getData(), true); // 执行任务 $result = executeTask($task); $pheanstalk->delete($job); // 任务执行完成后删除任务 } usleep(1000); // 休眠一毫秒,避免空转浪费CPU资源 } function executeTask($task) { // 执行具体任务逻辑 // ... }
Summary:
The above are several methods to solve the problem of high concurrent task scheduling in PHP development. By using technical means such as message queues, process pools, and distributed task scheduling, you can optimize the concurrency, resource competition, and system performance of task scheduling, and improve application stability and user experience.
The above code examples can be used as a reference, and the specific implementation may vary depending on application requirements. In the actual development process, factors such as business logic, performance tuning, and system resources also need to be comprehensively considered to choose an appropriate method to solve the problem.
The above is the detailed content of How to solve high-concurrency task scheduling problems in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


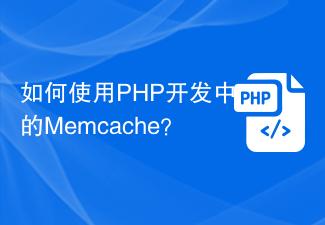
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
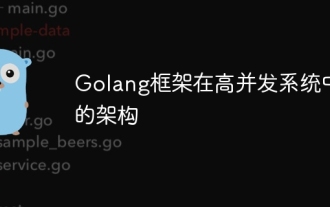
For high-concurrency systems, the Go framework provides architectural modes such as pipeline mode, Goroutine pool mode, and message queue mode. In practical cases, high-concurrency websites use Nginx proxy, Golang gateway, Goroutine pool and database to handle a large number of concurrent requests. The code example shows the implementation of a Goroutine pool for handling incoming requests. By choosing appropriate architectural patterns and implementations, the Go framework can build scalable and highly concurrent systems.
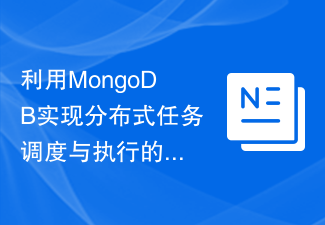
MongoDB is an open source NoSQL database with high performance, scalability and flexibility. In distributed systems, task scheduling and execution are a key issue. By utilizing the characteristics of MongoDB, distributed task scheduling and execution solutions can be realized. 1. Requirements Analysis for Distributed Task Scheduling In a distributed system, task scheduling is the process of allocating tasks to different nodes for execution. Common task scheduling requirements include: 1. Task request distribution: Send task requests to available execution nodes.
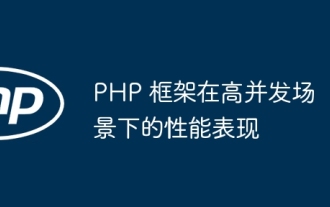
In high-concurrency scenarios, according to benchmark tests, the performance of the PHP framework is: Phalcon (RPS2200), Laravel (RPS1800), CodeIgniter (RPS2000), and Symfony (RPS1500). Actual cases show that the Phalcon framework achieved 3,000 orders per second during the Double Eleven event on the e-commerce website.
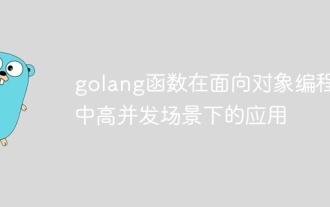
In high-concurrency scenarios of object-oriented programming, functions are widely used in the Go language: Functions as methods: Functions can be attached to structures to implement object-oriented programming, conveniently operating structure data and providing specific functions. Functions as concurrent execution bodies: Functions can be used as goroutine execution bodies to implement concurrent task execution and improve program efficiency. Function as callback: Functions can be passed as parameters to other functions and be called when specific events or operations occur, providing a flexible callback mechanism.
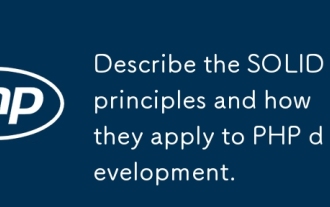
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
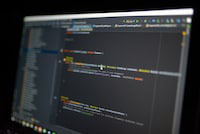
Asynchronous programming, English Asynchronous Programming, means that certain tasks in the program can be executed concurrently without waiting for other tasks to complete, thereby improving the overall operating efficiency of the program. In Python, the asyncio module is the main tool for implementing asynchronous programming. It provides coroutines, event loops, and other components required for asynchronous programming. Coroutine: Coroutine is a special function that can be suspended and then resumed execution, just like a thread, but a coroutine is more lightweight and consumes less memory than a thread. The coroutine is declared with the async keyword and execution is suspended at the await keyword. Event loop: Event loop (EventLoop) is the key to asynchronous programming
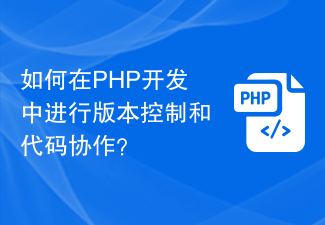
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
