Solutions to common array out-of-bounds problems in C++
Solutions to common array out-of-bounds problems in C require specific code examples
In C programming, array out-of-bounds is a common error. When we access an element in an array beyond the index range of the array, it will cause undefined behavior in the program. To avoid such errors we need to adopt some solutions.
Solution 1: Use array index correctly
First, we need to make it clear that the index of the array starts from 0. For example, an array with 5 elements has an index ranging from 0 to 4. Therefore, when accessing array elements, make sure that the index used is within the legal range.
int arr[5] = {1, 2, 3, 4, 5}; int index = 3; if(index >= 0 && index < 5) { int element = arr[index]; // 使用元素... } else { // 处理越界情况... }
In the above code, we first determine whether the index is within the legal range. If so, the array elements can be accessed safely. Otherwise, we need to handle out-of-bounds situations.
Solution 2: Avoid hardcoding array lengths
Another common mistake is using hardcoded array lengths. When we modify the size of the array, if we forget to modify the length of the array at the same time, it can easily lead to out-of-bounds problems. To avoid this error, we can use a variable to represent the length of the array.
int arr[] = {1, 2, 3, 4, 5}; int length = sizeof(arr) / sizeof(arr[0]); // 动态获取数组长度 int index = 5; // 越界索引 if(index >= 0 && index < length) { int element = arr[index]; // 使用元素... } else { // 处理越界情况... }
By using a dynamically calculated array length, we can avoid the error of hardcoding the length and ensure that the corrected length is not missed when modifying the array.
Solution 3: Use standard library containers
In addition to traditional arrays, C also provides a series of standard library containers, such as vector, list, etc. These containers provide more advanced functionality and enhanced security, making it easier to deal with out-of-bounds issues.
#include <vector> std::vector<int> vec = {1, 2, 3, 4, 5}; int index = 5; // 越界索引 if(index >= 0 && index < vec.size()) { int element = vec[index]; // 使用元素... } else { // 处理越界情况... }
Using vector containers, we can get the size of the container by calling the size() function without worrying about out-of-bounds issues.
Summary:
In C, avoiding array out-of-bounds problems is an important programming principle. To solve this problem, we can use array indexing correctly, avoid hardcoding array lengths, use standard library containers, etc. On the one hand, these methods can prevent out-of-bounds errors, and on the other hand, they can also improve the readability and maintainability of the program. When writing C code, we should always pay attention to the boundaries of the array to ensure the correct operation of the program.
The above is the detailed content of Solutions to common array out-of-bounds problems in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


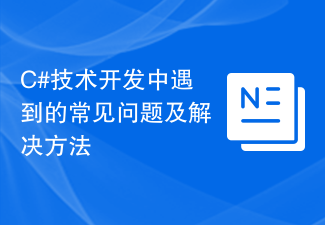
Common problems and solutions encountered in C# technology development Introduction: C# is an object-oriented high-level programming language that is widely used in the development of Windows applications. However, during the development process of C# technology, you may encounter some common problems. This article will introduce some common problems, provide corresponding solutions, and attach specific code examples to help readers better understand and solve these problems. 1. NullReferenceException (null reference exception) in the C# development process,
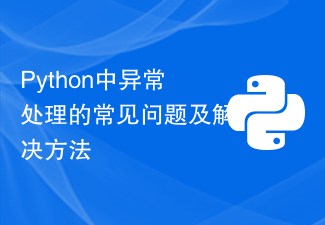
Common problems and solutions to exception handling in Python Introduction: When writing programs, it is difficult to avoid various errors and exceptions. Exception handling is a mechanism that can catch and handle these exceptions while the program is running, thereby ensuring the stability and reliability of the program. In Python, exception handling is a very important skill. This article will introduce common problems and solutions to exception handling in Python, and provide specific code examples. 1. Exception classification and common problems Grammar errors (SyntaxErr
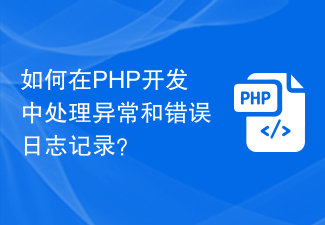
How to handle exceptions and error logging in PHP development? As a very popular back-end programming language, PHP is widely used in the field of web development. During the development process, we often need to handle exceptions and record error logs in order to discover and solve problems in time. This article will introduce best practices for handling exceptions and error logging in PHP development. 1. Exception handling In PHP, an exception is a special object used to handle error situations. When the code encounters an error that it cannot handle, we can throw an exception and
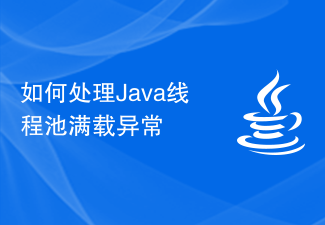
In Java development, thread pool is a very commonly used multi-threading mechanism. It can effectively manage, control and reuse threads, improving program performance and efficiency. However, in actual development, the thread pool may be fully loaded, causing tasks to fail to execute normally. This article will discuss how to handle thread pool full exceptions to improve program stability and reliability. First, we need to understand the cause of the thread pool full exception. The main reason why the thread pool is full is that task submission exceeds the maximum number of threads set by the thread pool. When a task is submitted to a thread
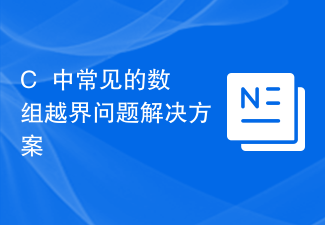
Solutions to common array out-of-bounds problems in C++ require specific code examples In C++ programming, array out-of-bounds is a common error. When we access an element in an array beyond the index range of the array, it will cause undefined behavior in the program. To avoid such errors we need to adopt some solutions. Solution 1: Use array index correctly First, we need to make sure that the index of the array starts from 0. For example, an array with 5 elements has an index ranging from 0 to 4. Therefore, when accessing array elements, make sure
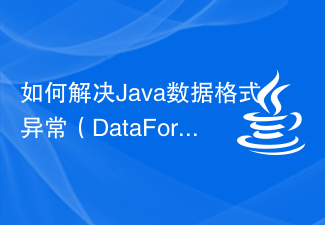
How to solve Java data format exception (DataFormatException) In Java programming, we often encounter various abnormal situations. Among them, data format exception (DataFormatException) is a common but also very challenging problem. This exception will be thrown when the input data cannot meet the specified format requirements. Solving this anomaly requires certain skills and experience. This article will detail how to resolve Java data format exceptions and provide some code examples
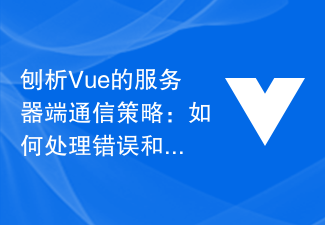
Analyzing Vue's server-side communication strategy: How to handle errors and exceptions When developing web applications, communicating with the server is an essential task. As a popular front-end framework, Vue.js provides a powerful set of tools and methods to handle communication with the server. In this article, we will focus on Vue’s server-side communication strategies and focus on how to effectively utilize these strategies when handling errors and exceptions. In Vue, we usually use axios to handle server-side communication. Ax

How to handle database errors in PHP? When developing PHP applications, interacting with the database is a very common and important part. However, when it comes to database operations, mistakes are inevitable. In order to deal with these errors and ensure the robustness and stability of the application, we need to handle database errors correctly. In this article, I will introduce you to some ways to handle database errors in PHP and provide specific code examples. Catching exceptions using try-catch block In PHP we can use try-ca
