How to implement distributed cache consistency in Java
How to achieve distributed cache consistency in Java
Introduction:
In distributed systems, caching is one of the important ways to improve system performance. However, the implementation of distributed cache is not simple due to issues related to data consistency. This article will introduce how to implement distributed cache consistency in Java and provide specific code examples.
1. The concept of distributed cache consistency
Distributed cache consistency means that in a distributed cache system, the data between all cache nodes is consistent. In other words, no matter which cache node the user performs read and write operations, they can obtain the same results.
2. Ways to achieve distributed cache consistency
There are many ways to achieve distributed cache consistency. Two common ways are introduced below.
- Cache consistency protocol
The cache consistency protocol is mainly divided into two types: strong consistency and weak consistency. Strong consistency means that data updates between cache nodes are performed synchronously, ensuring that the data of all nodes is consistent; weak consistency means that data updates between cache nodes are performed asynchronously, and data inconsistency may exist for a period of time.
In Java, ZooKeeper can be used to implement the cache consistency protocol. ZooKeeper is a distributed coordination service that can be used to achieve consistency in distributed applications.
The following is a sample code that uses ZooKeeper to achieve cache consistency:
public class DistributedCache { private ZooKeeper zooKeeper; public DistributedCache(String address) throws IOException { zooKeeper = new ZooKeeper(address, 5000, null); } public void put(String key, String value) throws KeeperException, InterruptedException { byte[] data = value.getBytes(); zooKeeper.setData("/cache/" + key, data, -1); } public String get(String key) throws KeeperException, InterruptedException { byte[] data = zooKeeper.getData("/cache/" + key, null, null); return new String(data); } }
- Cache update notification mechanism
The cache update notification mechanism refers to when the data of the cache node changes , automatically notify other nodes to update data.
In Java, message queue can be used to implement the cache update notification mechanism. The message queue can push messages to subscribers. After the subscribers receive the messages, they update the corresponding cached data.
The following is a sample code that uses RabbitMQ to implement cache update notification:
public class CacheUpdater { private Connection connection; private Channel channel; public CacheUpdater(String host, int port, String username, String password) throws IOException, TimeoutException { ConnectionFactory factory = new ConnectionFactory(); factory.setHost(host); factory.setPort(port); factory.setUsername(username); factory.setPassword(password); connection = factory.newConnection(); channel = connection.createChannel(); channel.queueDeclare("cache.update", false, false, false, null); } public void updateCache(String key, String value) throws IOException { String message = key + ":" + value; channel.basicPublish("", "cache.update", null, message.getBytes()); } }
3. Summary
This article introduces two ways to achieve distributed cache consistency in Java: caching Coherence protocol and cache update notification mechanism. Both methods can achieve distributed cache consistency, and the specific choice should be judged based on the actual situation. In actual development, you can choose a suitable solution according to your needs to achieve distributed cache consistency.
The above is the detailed content of How to implement distributed cache consistency in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
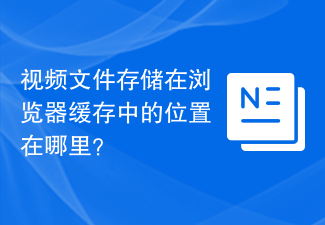
Which folder does the browser cache the video in? When we use the Internet browser every day, we often watch various online videos, such as watching music videos on YouTube or watching movies on Netflix. These videos will be cached by the browser during the loading process so that they can be loaded quickly when played again in the future. So the question is, in which folder are these cached videos actually stored? Different browsers store cached video folders in different locations. Below we will introduce several common browsers and their
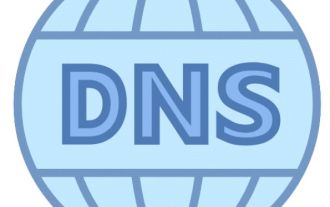
DNS (DomainNameSystem) is a system used on the Internet to convert domain names into corresponding IP addresses. In Linux systems, DNS caching is a mechanism that stores the mapping relationship between domain names and IP addresses locally, which can increase the speed of domain name resolution and reduce the burden on the DNS server. DNS caching allows the system to quickly retrieve the IP address when subsequently accessing the same domain name without having to issue a query request to the DNS server each time, thereby improving network performance and efficiency. This article will discuss with you how to view and refresh the DNS cache on Linux, as well as related details and sample code. Importance of DNS Caching In Linux systems, DNS caching plays a key role. its existence
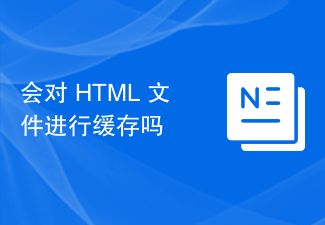
Title: Caching mechanism and code examples of HTML files Introduction: When writing web pages, we often encounter browser cache problems. This article will introduce the caching mechanism of HTML files in detail and provide some specific code examples to help readers better understand and apply this mechanism. 1. Browser caching principle In the browser, whenever a web page is accessed, the browser will first check whether there is a copy of the web page in the cache. If there is, the web page content is obtained directly from the cache. This is the basic principle of browser caching. Benefits of browser caching mechanism
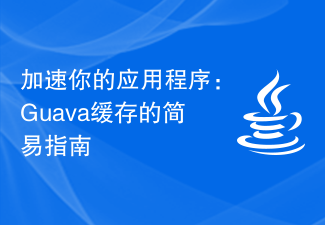
A Beginner's Guide to Guava Cache: Speed Up Your Applications Guava Cache is a high-performance in-memory caching library that can significantly improve application performance. It provides a variety of caching strategies, including LRU (least recently used), LFU (least recently used), and TTL (time to live). 1. Install Guava cache and add the dependency of Guava cache library to your project. com.goog
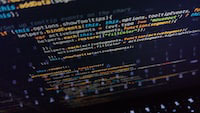
Optimizing Cache Size and Cleanup Strategies It is critical to allocate appropriate cache size to APCu. A cache that is too small cannot cache data effectively, while a cache that is too large wastes memory. Generally speaking, setting the cache size to 1/4 to 1/2 of the available memory is a reasonable range. Additionally, having an effective cleanup strategy ensures that stale or invalid data is not kept in the cache. You can use APCu's automatic cleaning feature or implement a custom cleaning mechanism. Sample code: //Set the cache size to 256MB apcu_add("cache_size",268435456); //Clear the cache every 60 minutes apcu_add("cache_ttl",60*60); Enable compression
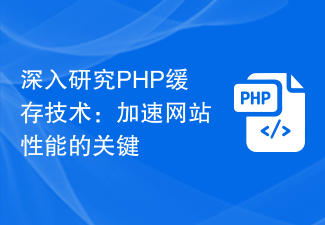
Exploration of PHP caching technology: a powerful tool to improve website performance, specific code examples are required Introduction: With the rapid development of today's Internet, website performance is crucial to user experience and search engine rankings. As a commonly used programming language, PHP is widely used in website development. How to improve the performance of PHP websites has become an urgent issue for developers. One of the very important solutions is to use PHP caching technology. This article will explore the concept and specific technology of PHP caching, and come with code examples to help readers understand
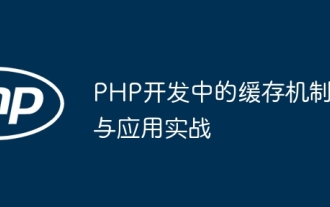
In PHP development, the caching mechanism improves performance by temporarily storing frequently accessed data in memory or disk, thereby reducing the number of database accesses. Cache types mainly include memory, file and database cache. Caching can be implemented in PHP using built-in functions or third-party libraries, such as cache_get() and Memcache. Common practical applications include caching database query results to optimize query performance and caching page output to speed up rendering. The caching mechanism effectively improves website response speed, enhances user experience and reduces server load.
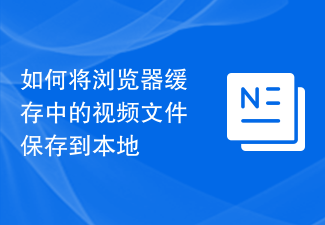
How to Export Browser Cache Videos With the rapid development of the Internet, videos have become an indispensable part of people's daily lives. When browsing the web, we often encounter video content that we want to save or share, but sometimes we cannot find the source of the video files because they may only exist in the browser's cache. So, how do you export videos from your browser cache? This article will introduce you to several common methods. First, we need to clarify a concept, namely browser cache. The browser cache is used by the browser to improve user experience.
