Detailed explanation of common coding standard issues in C++
Detailed explanation of common coding standard issues in C, specific code examples are required
Introduction:
In the software development process, good coding standards are to ensure code quality one of the important factors. A standardized coding style can improve code readability, maintainability, and team collaboration efficiency. This article will analyze in detail common coding standard issues in C and provide specific code examples to help readers better understand and apply these standards.
1. Naming specifications
- Class names, structure names and enumeration names use big camel case naming, such as MyClass, MyStruct, MyEnum.
- Function names, variable names and member variables use camel case naming, such as myFunction, myVariable, myMemberVariable.
- Use all capital letters for constant names and separate words with underscores, such as MY_CONSTANT.
- Naming should be descriptive, avoid using meaningless names, and try to follow domain-specific naming conventions.
Sample code:
class MyClass { public: enum MyEnum { ENUM_VALUE_1, ENUM_VALUE_2 }; void myFunction() { int myVariable = 0; const int MY_CONSTANT = 10; } private: int myMemberVariable; };
2. Indentation and alignment
- Use spaces instead of tabs for indentation, usually 4 spaces .
- For the curly braces of functions, use newlines and open symbol alignment, as shown in the following example.
Sample code:
void myFunction() { if (condition) { // do something } else { // do something else } }
3. Code comments
- For complex logic or key algorithms, detailed comments should be written to explain the code Intent and implementation details.
- In the header of each file, a brief description, author information, and modification history of the file should be provided.
Sample code:
/* * MyClass.h * * Description: This file contains the definition of MyClass. * Author: John Smith * Date: 2020-01-01 * * Modification history: * 2020-01-01: Initial version * ... */ class MyClass { public: void myFunction(); // This function does something };
4. The order of function and class definition
- Declare the function prototype first, and then define the function implementation.
- The constructor and destructor of the class should be placed first and last to facilitate the calling and finding of other member functions.
Sample code:
class MyClass { public: MyClass(); ~MyClass(); void myFunction(); void myOtherFunction(); private: int myVariable; };
5. Logic and maintainability of code
- Use good code structure and modular programming to compile the code Split into multiple functions, each function should be responsible for completing a clear task.
- Avoid using long functions, long files, and too many global variables to improve code readability and maintainability.
- Repetitive code that needs to be used multiple times should be abstracted into functions or macros to avoid code redundancy.
Sample code:
// Bad example void myFunction() { // a long piece of code... // ... // another long piece of code... // ... // more code... } // Good example void doSomething() { // a piece of code... } void doSomethingElse() { // another piece of code... } void myFunction() { doSomething(); doSomethingElse(); }
Conclusion:
This article analyzes common coding standard issues in C in detail and provides specific code examples. Good coding standards can improve code readability, maintainability and team collaboration efficiency. By following these conventions, we can write high-quality C code.
The above is the detailed content of Detailed explanation of common coding standard issues in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


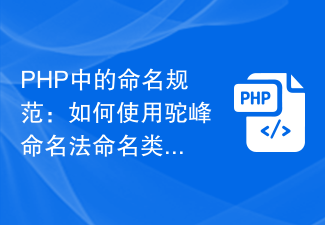
Naming conventions in PHP: How to use camelCase notation to name classes, methods, and variables In PHP programming, good naming conventions are an important coding practice. It improves code readability and maintainability, and makes teamwork smoother. In this article, we will explore a common naming convention: camelCase and provide some examples of how to use it in PHP to name classes, methods, and variables. 1. What is camel case nomenclature? CamelCase is a common naming convention in which the first letter of each word is capitalized,
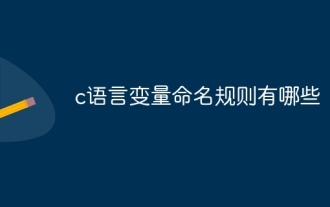
When writing a program, it is often necessary to store data in memory in order to use the data or modify the value of the data. We usually use variables to store data, and using variables can reference the data stored in memory and process the data at any time as needed.
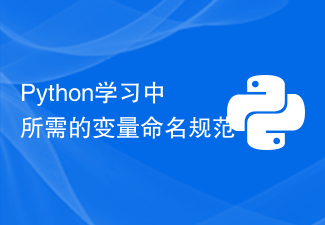
Variable naming conventions you need to know when learning Python An important aspect when learning the Python programming language is learning how to name and use variables correctly. Variables are identifiers used to store and represent data. Good variable naming conventions not only improve the readability of your code, but also reduce the possibility of errors. This article will introduce some commonly used variable naming conventions and give corresponding code examples. Use Meaningful Names Variable names should have a clear meaning and be able to describe the data stored in the variable. Using meaningful names allows it to
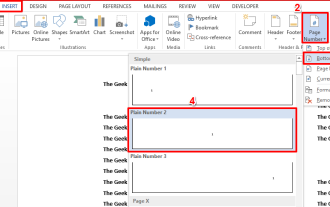
So, you have a huge table on one of the pages of your Word document, and you don't want the page numbers to be displayed on that page. At the same time, if the hidden page number is 7, you want to count the pages and the next page number should be 8. Well, you may have wasted a lot of time looking for a solution. Even if you find a solution, you may feel lost because you think it's too complex to implement. Well, GeekPage simplifies the complicated stuff for you. In this article, we have explained how to easily omit a certain page number from a Word document in very simple steps that you can easily understand. Hope you found this article helpful. How to Omit a Single Page Number Step 1: First, let’s insert in the footer
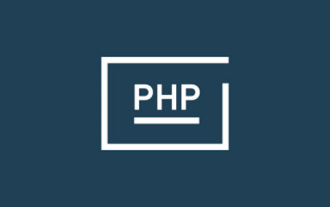
PHP's square brackets are a symbol, generally used to obtain the corresponding value of an array through the key name of the array. In fact, the syntax is such as "$array = array('a','b');echo $array[0] ;$user = $_POST['user'];$user = $_GET['user'];$user = $_SESSION['user'];".

With the increasingly perfect network environment and web page construction, PHP, as an excellent server-side scripting language, is widely used in the development of websites and applications. In order to better improve PHP programming skills, today we will share some common PHP programming skills. The following is a detailed introduction: Try to avoid using global variables. In PHP programming, global variables are a ubiquitous variable type. However, using global variables will increase the complexity and unreadability of the code, which can easily lead to unnecessary errors and problems. Therefore, when programming PHP
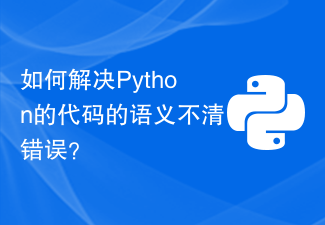
Python is a simple and easy-to-learn scripting language, but when writing code, unclear semantic errors often occur. These errors can seriously affect the correctness and maintainability of the program. This article will introduce how to solve unclear semantic errors in Python code. 1. Understand the language features of Python. The Python language has its own unique syntax and semantics. To avoid unclear semantic errors, you must first understand the language features of Python. Python is an object-oriented language that supports important modules, functions, variables, etc.
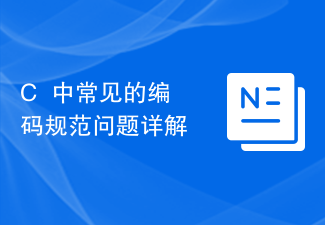
Detailed explanation of common coding standard issues in C++, specific code examples are required Introduction: In the software development process, good coding standards are one of the important factors to ensure code quality. A standardized coding style can improve code readability, maintainability, and team collaboration efficiency. This article will analyze in detail common coding standard issues in C++ and provide specific code examples to help readers better understand and apply these standards. 1. Naming standards: Class names, structure names and enumeration names use big camel case naming method, such as MyClass, MyStruc
