Analysis and solutions to file operation problems in C++
Analysis and Solutions of File Operation Problems in C
In C programming, file operation is a very common requirement. However, some problems may arise due to various reasons. This article will analyze several common file operation problems and provide corresponding solutions, along with specific code examples.
Problem 1: File opening failure
When we try to open a file, sometimes we encounter file opening failure. This may be caused by the file not existing, wrong file path, permission issues, etc. In order to solve this problem, we can use the fail() function of the file stream object to determine whether the file is successfully opened, and use the error handling mechanism to handle exceptions.
#include <iostream> #include <fstream> int main() { std::ifstream file("file.txt"); // 尝试打开文件 if (file.fail()) { std::cerr << "文件打开失败!" << std::endl; return 1; } // 文件打开成功后的操作代码... file.close(); // 关闭文件 return 0; }
Question 2: File reading failure
When we read data from a file, sometimes the file reading fails. This may be due to reasons such as incorrect file format, empty file content, or incorrect reading position. In order to solve this problem, we can use the eof() function of the file stream object to determine whether the end of the file is reached, and use a loop to continuously read the file content.
#include <iostream> #include <fstream> #include <string> int main() { std::ifstream file("file.txt"); // 打开文件 if (!file) { std::cerr << "文件打开失败!" << std::endl; return 1; } std::string line; while (std::getline(file, line)) { std::cout << line << std::endl; } if (file.eof()) { std::cout << "文件读取完毕。" << std::endl; } else { std::cerr << "文件读取失败!" << std::endl; } file.close(); // 关闭文件 return 0; }
Question 3: File writing failure
When we write data to the file, sometimes the file writing fails. This may be caused by reasons such as the file being read-only, insufficient disk space, or writing to the wrong location. In order to solve this problem, we can use the good() function of the file stream object to determine whether the write operation is successful, and use the error handling mechanism to handle exceptions.
#include <iostream> #include <fstream> int main() { std::ofstream file("file.txt", std::ios::app); // 打开文件,追加内容 if (!file.good()) { std::cerr << "文件打开失败!" << std::endl; return 1; } file << "写入的内容" << std::endl; if (!file.good()) { std::cerr << "文件写入失败!" << std::endl; } else { std::cout << "文件写入成功。" << std::endl; } file.close(); // 关闭文件 return 0; }
Question 4: File deletion failure
When trying to delete a file, file deletion failure may occur. This may be caused by the file being occupied by other programs, insufficient permissions, or the file not existing. To solve this problem, we can use the standard library function remove() to delete the file and use the error handling mechanism to handle exceptions.
#include <iostream> #include <cstdio> int main() { const char* filename = "file.txt"; int result = std::remove(filename); if (result != 0) { std::cerr << "文件删除失败!" << std::endl; return 1; } std::cout << "文件删除成功。" << std::endl; return 0; }
Through the above code examples, we can implement solutions to file operation problems in C. Of course, in actual applications, more complex problems may be encountered, but by learning and understanding these basic principles and methods, we can better handle various abnormal situations in file operations. I hope this article can be helpful to readers.
The above is the detailed content of Analysis and solutions to file operation problems in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


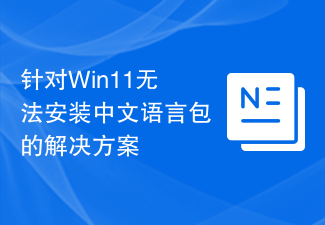
Win11 is the latest operating system launched by Microsoft. Compared with previous versions, Win11 has greatly improved the interface design and user experience. However, some users reported that they encountered the problem of being unable to install the Chinese language pack after installing Win11, which caused trouble for them to use Chinese in the system. This article will provide some solutions to the problem that Win11 cannot install the Chinese language pack to help users use Chinese smoothly. First, we need to understand why the Chinese language pack cannot be installed. Generally speaking, Win11
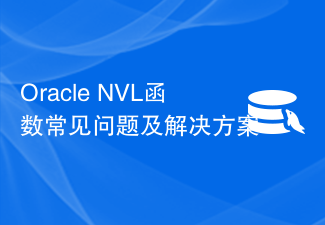
Common problems and solutions for OracleNVL function Oracle database is a widely used relational database system, and it is often necessary to deal with null values during data processing. In order to deal with the problems caused by null values, Oracle provides the NVL function to handle null values. This article will introduce common problems and solutions of NVL functions, and provide specific code examples. Question 1: Improper usage of NVL function. The basic syntax of NVL function is: NVL(expr1,default_value).
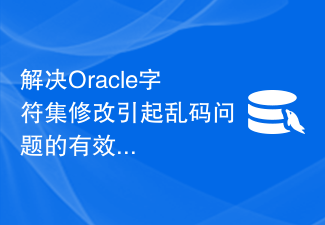
Title: An effective solution to solve the problem of garbled characters caused by Oracle character set modification. In Oracle database, when the character set is modified, the problem of garbled characters often occurs due to the presence of incompatible characters in the data. In order to solve this problem, we need to adopt some effective solutions. This article will introduce some specific solutions and code examples to solve the problem of garbled characters caused by Oracle character set modification. 1. Export data and reset the character set. First, we can export the data in the database by using the expdp command.
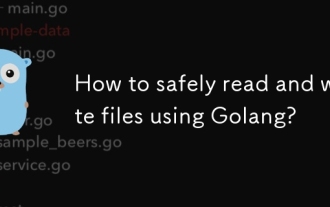
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
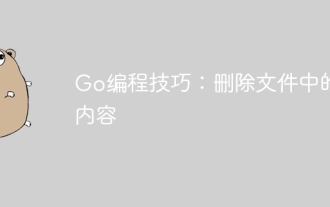
The Go language provides two methods to clear file contents: using io.Seek and io.Truncate, or using ioutil.WriteFile. Method 1 involves moving the cursor to the end of the file and then truncating the file, method 2 involves writing an empty byte array to the file. The practical case demonstrates how to use these two methods to clear content in Markdown files.
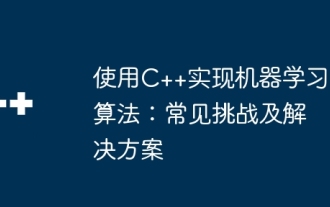
Common challenges faced by machine learning algorithms in C++ include memory management, multi-threading, performance optimization, and maintainability. Solutions include using smart pointers, modern threading libraries, SIMD instructions and third-party libraries, as well as following coding style guidelines and using automation tools. Practical cases show how to use the Eigen library to implement linear regression algorithms, effectively manage memory and use high-performance matrix operations.
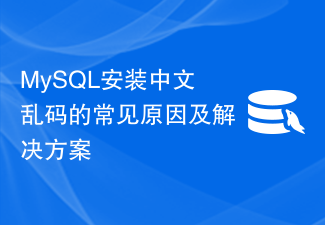
Common reasons and solutions for Chinese garbled characters in MySQL installation MySQL is a commonly used relational database management system, but you may encounter the problem of Chinese garbled characters during use, which brings trouble to developers and system administrators. The problem of Chinese garbled characters is mainly caused by incorrect character set settings, inconsistent character sets between the database server and the client, etc. This article will introduce in detail the common causes and solutions of Chinese garbled characters in MySQL installation to help everyone better solve this problem. 1. Common reasons: character set setting
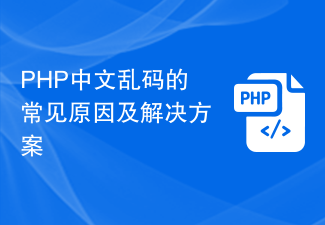
Common causes and solutions for PHP Chinese garbled characters. With the development of the Internet, Chinese websites play an increasingly important role in our lives. However, in PHP development, the problem of Chinese garbled characters is still a common problem that troubles developers. This article will introduce the common causes of Chinese garbled characters in PHP and provide solutions. It also attaches specific code examples for readers' reference. 1. Common reasons: Inconsistent character encoding: Inconsistencies in PHP file encoding, database encoding, HTML page encoding, etc. may lead to Chinese garbled characters. database
