


How to use iterators and recursive algorithms to process data in C#
How to use iterators and recursive algorithms to process data in C# requires specific code examples
In C#, iterators and recursive algorithms are two commonly used data processing method. Iterators can help us traverse the elements in a collection, and recursive algorithms can handle complex problems efficiently. This article details how to use iterators and recursive algorithms to process data, and provides specific code examples.
- Use iterators to process data
In C#, we can use iterators to traverse the elements in a collection without knowing the size of the collection in advance. Through iterators, we can access the elements in the collection one by one and operate on them.
First, we need to define a class that implements the IEnumerable
The following is a sample code that uses an iterator to traverse the elements of a collection:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
|
Running the above code will output elements 1, 2, and 3 in the collection.
- Use recursive algorithms to process data
The recursive algorithm is a method that solves problems by calling itself. When dealing with complex problems, recursive algorithms often provide concise and efficient solutions.
The following is a sample code that uses recursive algorithm to calculate the Fibonacci sequence:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
|
Running the above code will output the first 10 numbers of the Fibonacci sequence.
The above is an introduction to how to use iterators and recursive algorithms to process data, as well as specific code examples. Iterators and recursive algorithms are commonly used data processing methods in C#, through which we can handle various data structures and requirements more flexibly. Hope this article helps you!
The above is the detailed content of How to use iterators and recursive algorithms to process data in C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
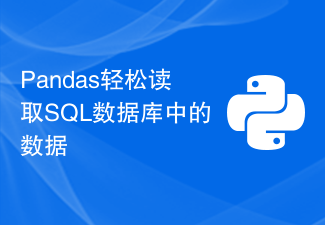
Data processing tool: Pandas reads data in SQL databases and requires specific code examples. As the amount of data continues to grow and its complexity increases, data processing has become an important part of modern society. In the data processing process, Pandas has become one of the preferred tools for many data analysts and scientists. This article will introduce how to use the Pandas library to read data from a SQL database and provide some specific code examples. Pandas is a powerful data processing and analysis tool based on Python
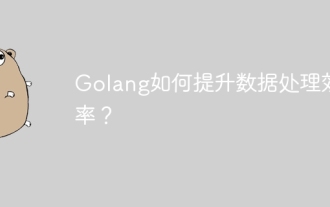
Golang improves data processing efficiency through concurrency, efficient memory management, native data structures and rich third-party libraries. Specific advantages include: Parallel processing: Coroutines support the execution of multiple tasks at the same time. Efficient memory management: The garbage collection mechanism automatically manages memory. Efficient data structures: Data structures such as slices, maps, and channels quickly access and process data. Third-party libraries: covering various data processing libraries such as fasthttp and x/text.
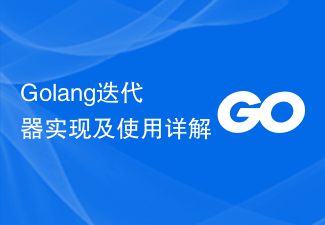
Golang is a fast and efficient statically compiled language. Its concise syntax and powerful performance make it very popular in the field of software development. In Golang, iterator (Iterator) is a commonly used design pattern for traversing elements in a collection without exposing the internal structure of the collection. This article will introduce in detail how to implement and use iterators in Golang, and help readers better understand through specific code examples. 1. Definition of iterator In Golang, iterator usually consists of an interface and implementation
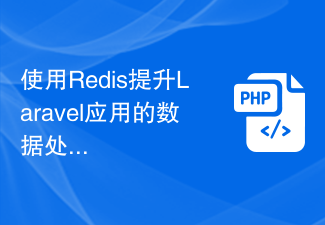
Use Redis to improve the data processing efficiency of Laravel applications. With the continuous development of Internet applications, data processing efficiency has become one of the focuses of developers. When developing applications based on the Laravel framework, we can use Redis to improve data processing efficiency and achieve fast access and caching of data. This article will introduce how to use Redis for data processing in Laravel applications and provide specific code examples. 1. Introduction to Redis Redis is a high-performance memory data
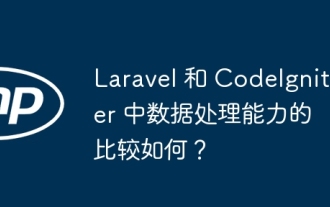
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
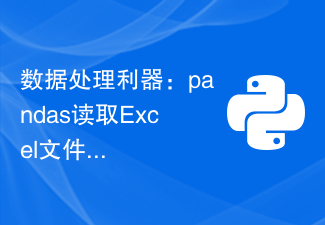
With the increasing popularity of data processing, more and more people are paying attention to how to use data efficiently and make the data work for themselves. In daily data processing, Excel tables are undoubtedly the most common data format. However, when a large amount of data needs to be processed, manually operating Excel will obviously become very time-consuming and laborious. Therefore, this article will introduce an efficient data processing tool - pandas, and how to use this tool to quickly read Excel files and perform data processing. 1. Introduction to pandas pandas

Efficient data processing: Using Pandas to modify column names requires specific code examples. Data processing is a very important part of data analysis, and during the data processing process, it is often necessary to modify the column names of the data. Pandas is a powerful data processing library that provides a wealth of methods and functions to help us process data quickly and efficiently. This article will introduce how to use Pandas to modify column names and provide specific code examples. In actual data analysis, the column names of the original data may have inconsistent naming standards and are difficult to understand.
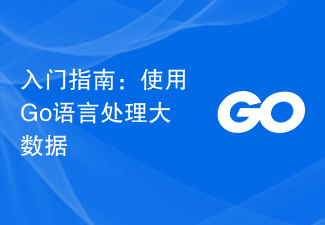
As an open source programming language, Go language has gradually received widespread attention and use in recent years. It is favored by programmers for its simplicity, efficiency, and powerful concurrent processing capabilities. In the field of big data processing, the Go language also has strong potential. It can be used to process massive data, optimize performance, and can be well integrated with various big data processing tools and frameworks. In this article, we will introduce some basic concepts and techniques of big data processing in Go language, and show how to use Go language through specific code examples.
