


How to debug and optimize database connections in PHP development
How to debug and optimize database connections in PHP development requires specific code examples
Introduction:
In PHP development, the database is a very critical component , good database connection debugging and optimization can effectively improve the performance of the website. This article explains how to debug and optimize database connections and provides some concrete code examples.
1. Debugging database connection:
- Use PDO to connect to the database:
Connect to the database through PDO, you can easily obtain error information in the database connection. First, ensure that the relevant configuration information of the database is correct, and then add error handling logic to the code to capture database connection errors and output error information.
<?php try { $dbh = new PDO('mysql:host=localhost;dbname=test', 'username', 'password'); } catch (PDOException $e) { echo '数据库连接失败: ' . $e->getMessage(); } ?>
- Use mysqli to connect to the database:
For the case of using mysqli to connect to the database, you can use the mysqli_connect_errno() and mysqli_connect_error() functions to obtain information about database connection errors.
<?php $con = mysqli_connect("localhost","username","password","test"); if (mysqli_connect_errno()) { echo "数据库连接错误: " . mysqli_connect_error(); } ?>
- Record database connection error log:
In addition to outputting error information directly, you can also record database connection error information into a log file to facilitate subsequent review and analysis. Error information can be written to the specified log file by using the error_log() function.
<?php try { $dbh = new PDO('mysql:host=localhost;dbname=test', 'username', 'password'); } catch (PDOException $e) { error_log('数据库连接失败: ' . $e->getMessage(), 3, '/path/to/error.log'); } ?>
2. Optimize database connection:
- Use long connection:
Using long connection can avoid the overhead of frequent connection/disconnection of the database and improve database connection. s efficiency. For MySQL database, you can add"pconnect=true"
to the connection string to enable long connections. However, it should be noted that long connections will also occupy the resources of the database server. If the connections are not used for a long time, the number of connections to the server may reach the upper limit. - Optimize the database connection pool:
In the case of high concurrency, the use of connection pools can effectively manage and reuse database connections and improve the efficiency of database connections. Using a connection pool allows the connection creation and closing process to be managed by the connection pool, reducing the overhead of connection creation.
<?php class ConnectionPool { private static $pool; public static function getInstance() { if (!self::$pool) { self::$pool = new self(); } return self::$pool; } private function __construct() { // 初始化连接池 } public function getConnection() { // 从连接池中获取数据库连接 } public function releaseConnection($connection) { // 将连接释放到连接池中 } } $pool = ConnectionPool::getInstance(); $connection = $pool->getConnection(); // 执行数据库操作 $pool->releaseConnection($connection); ?>
- Optimize database query:
Optimizing database query can reduce the consumption of database connections and improve the efficiency of database query. Common optimization methods include using indexes, merging queries, optimizing complex query statements, etc.
<?php $pdo = new PDO('mysql:host=localhost;dbname=test', 'username', 'password'); $stmt = $pdo->prepare("SELECT * FROM users WHERE age > :age"); $stmt->bindParam(':age', $age, PDO::PARAM_INT); $stmt->execute(); $result = $stmt->fetchAll(PDO::FETCH_ASSOC); ?>
Conclusion:
Debugging and optimizing database connections are very important in PHP development. By using error handling mechanisms, recording error logs, and optimizing connection methods and query statements, the stability and performance of database connections can be improved. It should be noted that during the process of optimizing database connections and queries, adjustments should be made according to the actual situation to achieve the best performance.
Reference materials:
- PHP official documentation: https://www.php.net/manual/zh/book.pdo.php
- PHP official documentation :https://www.php.net/manual/zh/book.mysqli.php
- PHP connection pool implementation: https://github.com/swoole/library/blob/master/ConnectionPool/README .md
The above is the detailed content of How to debug and optimize database connections in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


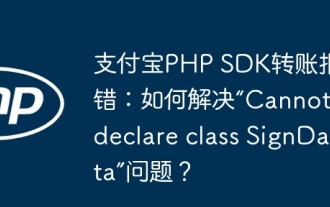
Alipay PHP...
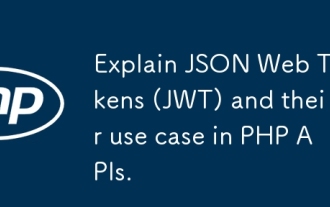
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
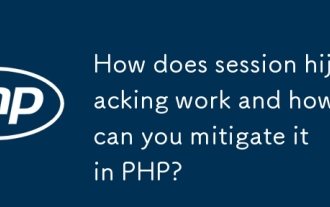
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
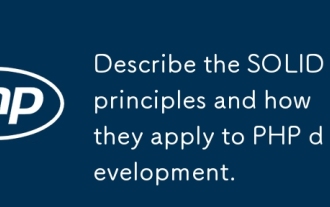
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
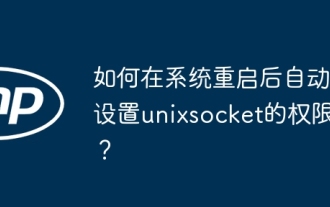
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
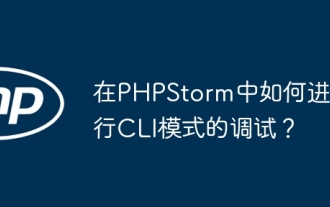
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
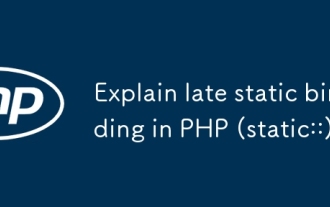
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
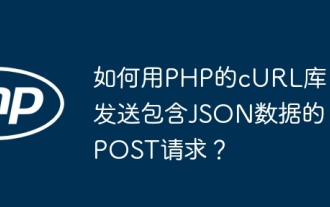
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
