How to deal with concurrent data access issues in Go language?
In the Go language, dealing with concurrent data access issues is a very important task. Due to the concurrent programming model characteristics of the Go language, we can easily implement concurrent read and write operations. The following will introduce some common methods of dealing with concurrent data access problems and give specific code examples.
- Mutex lock (Mutex)
Mutex lock is one of the most commonly used methods to deal with concurrent access problems in the Go language. It can ensure that only one goroutine can access the protected object at the same time. resource. The following is a sample code that uses a mutex lock to solve concurrent access problems:
package main import ( "fmt" "sync" ) var ( count int mutex sync.Mutex ) func main() { // 创建一个WaitGroup,用于等待所有goroutine完成 var wg sync.WaitGroup // 启动10个goroutine并发地对count进行自增操作 for i := 0; i < 10; i++ { wg.Add(1) go increment(&wg) } // 等待所有goroutine完成 wg.Wait() fmt.Println("Final count:", count) } func increment(wg *sync.WaitGroup) { // 在函数退出时释放锁 defer wg.Done() // 获取互斥锁 mutex.Lock() defer mutex.Unlock() // 修改被保护的资源 count++ }
In this example, we use sync.Mutex
to create a mutex lock and Add mutex locking and unlocking operations where access to protected resources is required. This ensures that only one goroutine can access the count global variable at the same time.
- Read-Write Lock (RWMutex)
Mutex locks can cause performance problems when facing concurrent read operations and a small number of write operations. The read-write lock (RWMutex) is a more efficient solution, which allows multiple goroutines to read protected resources at the same time, but only one goroutine can perform a write operation.
The following is a sample code that uses read-write locks to solve concurrent access problems:
package main import ( "fmt" "sync" ) var ( count int lock sync.RWMutex wg sync.WaitGroup ) func main() { // 启动100个goroutine并发地读取count值 for i := 0; i < 100; i++ { wg.Add(1) go read(&wg) } // 启动10个goroutine并发地对count进行自增操作 for i := 0; i < 10; i++ { wg.Add(1) go increment(&wg) } wg.Wait() fmt.Println("Final count:", count) } func read(wg *sync.WaitGroup) { defer wg.Done() // 获取读锁 lock.RLock() defer lock.RUnlock() // 读取被保护的资源 fmt.Println("Read:", count) } func increment(wg *sync.WaitGroup) { defer wg.Done() // 获取写锁 lock.Lock() defer lock.Unlock() // 修改被保护的资源 count++ }
In this example, we use sync.RWMutex
to create a Read and write locks, and use the RLock
method to acquire the read lock, the Lock
method to acquire the write lock, and use the RUnlock
and Unlock
methods to release the lock . This ensures that for read operations, multiple goroutines can be performed concurrently, but for write operations, only one goroutine can be performed.
In actual applications, other methods can also be used to deal with concurrent data access issues according to specific needs, such as channels, atomic operations, etc. The above are just some of the common methods, I hope they will be helpful to you.
The above is the detailed content of How to deal with concurrent data access issues in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
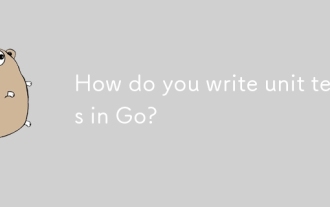
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
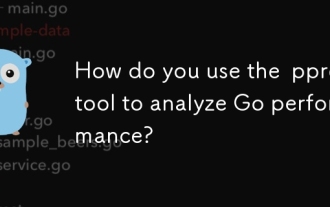
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
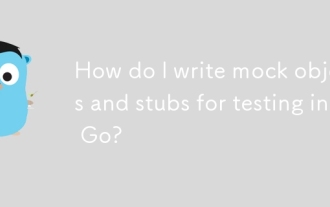
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
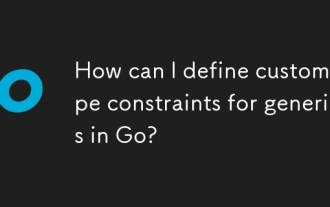
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
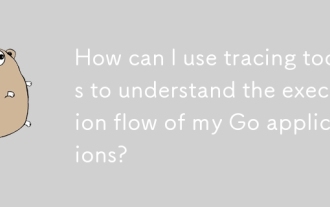
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
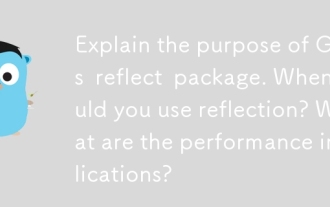
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
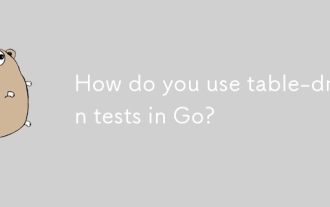
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
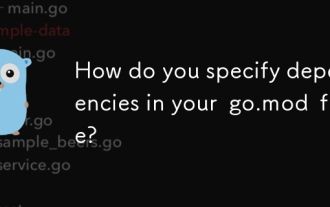
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
