Target drift problem in object tracking
The target drift problem in object tracking requires specific code examples
In the field of computer vision, object tracking is a very important task, and it can be applied to many Fields such as intelligent monitoring, autonomous driving, etc. However, with the complexity of target motion and uncertainty of environmental conditions, the target drift problem becomes a challenge in object tracking.
Target drift means that after a period of tracking, the target position tracked by the object tracking algorithm will deviate from the true position. This problem occurs mainly for two reasons: the target's own movement and changes in the environment.
In order to solve the problem of target drift, we can use different algorithms and techniques. A specific code example is given below to show a common method to solve the target drift problem-Kalman filter.
import numpy as np class KalmanFilter: def __init__(self, dt, u, std_acc, std_meas): self.dt = dt self.u = u self.std_acc = std_acc self.std_meas = std_meas self.A = np.array([[1, dt], [0, 1]]) self.B = np.array([0.5 * dt**2, dt]) self.H = np.array([[1, 0]]) self.Q = np.array([[0.25 * dt**4, 0.5 * dt**3], [0.5 * dt**3, dt**2]]) * std_acc**2 self.R = std_meas**2 self.state = np.zeros((2, 1)) self.P = np.zeros((2, 2)) def update(self, z): prediction = self.A @ self.state + self.B * self.u predict_cov = self.A @ self.P @ self.A.T + self.Q K = predict_cov @ self.H.T @ np.linalg.inv(self.H @ predict_cov @ self.H.T + self.R) self.state = prediction + K @ (z - self.H @ prediction) self.P = (np.eye(2) - K @ self.H) @ predict_cov # 使用示例 dt = 0.1 u = 0 std_acc = 0.1 std_meas = 0.1 kf = KalmanFilter(dt, u, std_acc, std_meas) # 假设在第0时刻目标位置为[0, 0] true_position = np.array([[0, 0]]).T # 生成时间序列 T = 10 time = np.arange(0, T, dt) # 生成测量序列 meas = true_position + np.random.randn(len(time), 1) * std_meas # 进行物体跟踪 for i, z in enumerate(meas): kf.update(z) print("Time: {:.1f}, Measured Position: [{:.1f}, {:.1f}], Estimated Position: [{:.1f}, {:.1f}]".format( time[i], z[0], z[1], kf.state[0], kf.state[1]))
In the above code, we first define a Kalman filter class KalmanFilter
, which includes initialization, update and other methods. In the example, we assume that the target motion is a uniform linear motion, and use the Kalman filter to estimate the target's position by given the true position and the measured value with Gaussian noise added.
In actual applications, we can set and adjust parameters according to specific scenarios and needs. It should be noted that the solution to the target drift problem does not only rely on algorithms and technologies, but also needs to take into account changes in the environment and the movement characteristics of the target itself. Therefore, in practical applications, we need to select algorithms and adjust parameters according to specific situations so that the object tracking algorithm can better resist the target drift problem.
The above is the detailed content of Target drift problem in object tracking. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




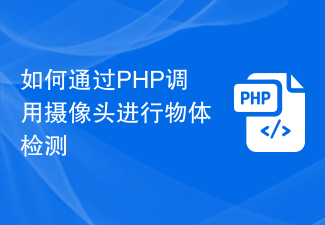
How to call the camera for object detection through PHP Cameras have become very common in modern life. We can use cameras to perform various operations, one of which is object detection. This article will introduce how to use PHP language to call the camera and perform object detection. Before we begin, we need to make sure that PHP is installed and the camera is available. Following are the steps to use PHP for object detection: Install relevant libraries To use PHP for object detection, we first need to install some necessary libraries. Here we will make
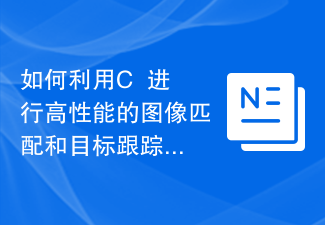
How to use C++ for high-performance image matching and target tracking? Overview: Image matching and target tracking are important research directions in the field of computer vision and have a wide range of applications, including object recognition, detection, tracking, etc. In this article, we will introduce how to implement high-performance image matching and target tracking algorithms using the C++ programming language and explain in detail with code examples. 1. Image matching: Image matching refers to finding similar feature points or corresponding feature areas between different images, thereby achieving registration or alignment between images. C++
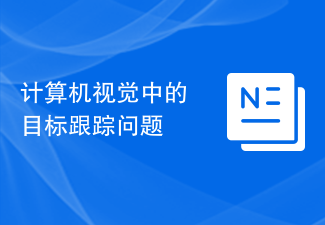
The target tracking problem in computer vision requires specific code examples Introduction: With the development of artificial intelligence, computer vision has been widely used in various fields, among which the target tracking problem is an important research direction in computer vision. Target tracking aims to use computer algorithms to continuously, accurately and real-time track targets in videos. It is widely used in video surveillance, driverless driving, virtual reality and other fields, bringing great convenience to applications in various scenarios. This article will introduce the basic concepts and common algorithms of target tracking, and give a specific
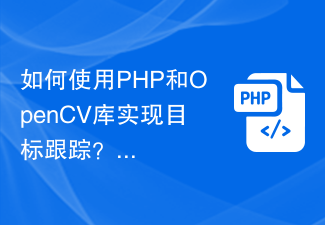
How to implement target tracking using PHP and OpenCV library? Object tracking is an important problem in computer vision, which involves identifying and tracking objects of interest in continuous image sequences. In this article, we will discuss how to implement object tracking using PHP and the OpenCV library and provide some code examples. Install PHP and OpenCV libraries First, we need to install PHP and the corresponding OpenCV extension library. The relevant libraries can be installed by executing the following command in the terminal or command line: sudoap
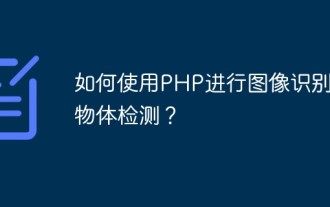
With the continuous development of artificial intelligence technology, image recognition and object detection have become popular research directions. In practice, PHP, as a popular scripting language, can also be used for image recognition and object detection. This article will introduce how to use PHP for image recognition and object detection. 1. PHP image processing library Before using PHP for image recognition and object detection, you need to prepare some basic tools. Among them, the PHP image processing library is an indispensable tool. The main function of the PHP image processing library is to provide some basic graphics
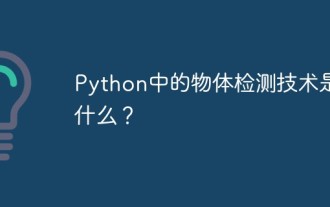
With the continuous development of artificial intelligence technology, object detection technology is becoming more and more important in the field of computer vision. Among them, the application of Python language is becoming more and more widespread. This article will introduce object detection technology in Python. 1. What is object detection technology? Object detection technology, as the name suggests, is a technology that automatically identifies specific objects in images or videos. This technique usually consists of two stages: first, object localization. That is to find the location of the object in the image. The second is object recognition. That is, determine the type of object.
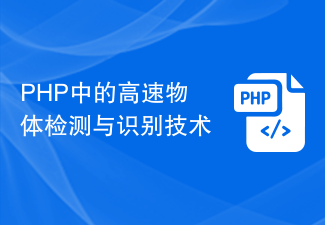
When we talk about PHP, we often think of rapid Web development and data processing, but rarely talk about its application in the field of high-speed object detection and recognition technology. However, PHP plays a very important role in this field. Its simple and easy-to-use syntax structure and rich extensible modules provide a powerful platform for high-speed object detection and recognition technology. First, we need to understand what high-speed object detection and recognition technology is. In short, it is a technology that automatically tracks and identifies high-speed moving objects through computers. in some
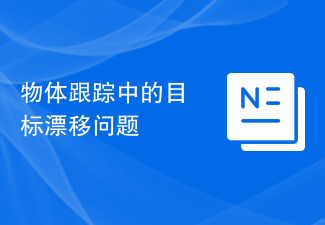
The target drift problem in object tracking requires specific code examples. In the field of computer vision, object tracking is a very important task, and it can be applied to many fields, such as intelligent monitoring, autonomous driving, etc. However, with the complexity of target motion and uncertainty of environmental conditions, the target drift problem becomes a challenge in object tracking. Target drift means that after a period of tracking, the target position tracked by the object tracking algorithm deviates from the true position. There are two main reasons why this problem occurs: the target's own movement and changes in the environment.
