


How to deal with database transaction issues in C# development
How to handle database transactions in C# development requires specific code examples
Introduction:
In C# development, the processing of database transactions is very important technology. Through transaction processing, we can ensure the consistency and integrity of database operations and improve the stability and security of the system. This article will introduce how to handle database transactions in C# and give specific code examples.
1. Introduction to database transactions
A database transaction is a logical unit of database operations, which can be composed of one or more operations. Transactions have four basic properties, namely atomicity, consistency, isolation and durability, which are often referred to as ACID properties.
Atomicity: Either all operations in a transaction are executed, or none are executed.
Consistency: The database remains in a consistent state before and after transaction execution.
Isolation: The execution of transactions will not interfere with each other, and each transaction considers itself to be the only one executing.
Durability: Once a transaction is submitted, its results will be permanently stored in the database.
Database transaction processing in C# is mainly implemented through the Transaction class in ADO.NET. The following will introduce how to use the Transaction class in C# to handle database transaction issues.
2. Examples of database transaction processing in C
#Example 1: Open a transaction and submit
using (SqlConnection connection = new SqlConnection(connectionString)) { connection.Open(); // 开启事务 using (SqlTransaction transaction = connection.BeginTransaction()) { try { // 执行一系列数据库操作 // 提交事务 transaction.Commit(); } catch (Exception ex) { // 发生异常,回滚事务 transaction.Rollback(); // 处理异常 Console.WriteLine("发生异常:" + ex.Message); } } }
Example 2: Set the isolation level of the transaction
using (SqlConnection connection = new SqlConnection(connectionString)) { connection.Open(); // 开启事务,并设置隔离级别为Serializable using (SqlTransaction transaction = connection.BeginTransaction(IsolationLevel.Serializable)) { try { // 执行一系列数据库操作 // 提交事务 transaction.Commit(); } catch (Exception ex) { // 发生异常,回滚事务 transaction.Rollback(); // 处理异常 Console.WriteLine("发生异常:" + ex.Message); } } }
Example 3: Transaction processing across multiple databases
using (SqlConnection connection1 = new SqlConnection(connectionString1)) using (SqlConnection connection2 = new SqlConnection(connectionString2)) { connection1.Open(); connection2.Open(); // 开启事务 using (SqlTransaction transaction1 = connection1.BeginTransaction()) using (SqlTransaction transaction2 = connection2.BeginTransaction()) { try { // 在connection1上执行一系列数据库操作 // 在connection2上执行一系列数据库操作 // 提交事务 transaction1.Commit(); transaction2.Commit(); } catch (Exception ex) { // 发生异常,回滚事务 transaction1.Rollback(); transaction2.Rollback(); // 处理异常 Console.WriteLine("发生异常:" + ex.Message); } } }
3. Summary
By using the Transaction class in C#, we can easily handle database transaction issues and ensure the consistency and integrity of database operations. In actual development, we need to select an appropriate transaction isolation level based on specific business needs and system requirements, and commit or rollback transactions according to specific circumstances to ensure the validity and stability of data.
The above is an introduction and sample code for handling database transaction issues in C# development. I hope to be helpful!
The above is the detailed content of How to deal with database transaction issues in C# development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


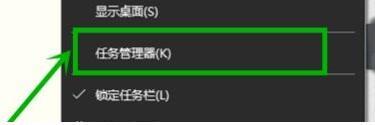
1. First, we right-click the blank space of the taskbar and select the [Task Manager] option, or right-click the start logo, and then select the [Task Manager] option. 2. In the opened Task Manager interface, we click the [Services] tab on the far right. 3. In the opened [Service] tab, click the [Open Service] option below. 4. In the [Services] window that opens, right-click the [InternetConnectionSharing(ICS)] service, and then select the [Properties] option. 5. In the properties window that opens, change [Open with] to [Disabled], click [Apply] and then click [OK]. 6. Click the start logo, then click the shutdown button, select [Restart], and complete the computer restart.
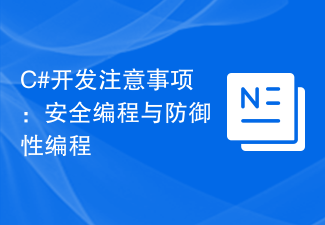
C# is a widely used object-oriented programming language that is easy to learn, strongly typed, safe, reliable, efficient and has high development efficiency. However, C# programs may still be subject to malicious attacks or program errors caused by unintentional negligence. When writing C# programs, we should pay attention to the principles of safe programming and defensive programming to ensure the safety, reliability, and stability of the program. 1. Principles of secure programming 1. Do not trust user input. If there is insufficient verification in a C# program, malicious users can easily enter malicious data and attack the program.
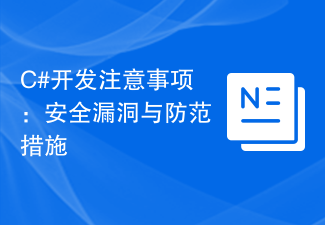
C# is a programming language widely used on Windows platforms. Its popularity is inseparable from its powerful functions and flexibility. However, precisely because of its wide application, C# programs also face various security risks and vulnerabilities. This article will introduce some common security vulnerabilities in C# development and discuss some preventive measures. Input validation of user input is one of the most common security holes in C# programs. Unvalidated user input may contain malicious code, such as SQL injection, XSS attacks, etc. To protect against such attacks, all
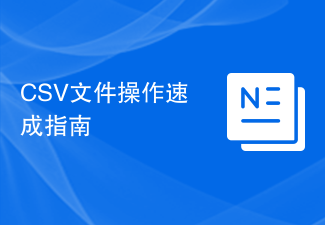
Quickly learn how to open and process CSV format files. With the continuous development of data analysis and processing, CSV format has become one of the widely used file formats. A CSV file is a simple and easy-to-read text file with different data fields separated by commas. Whether in academic research, business analysis or data processing, we often encounter situations where we need to open and process CSV files. The following guide will show you how to quickly learn to open and process CSV format files. Step 1: Understand the CSV file format First,
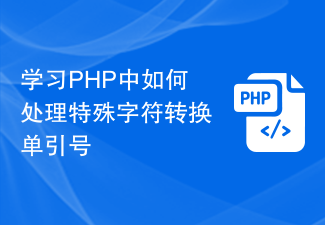
In the process of PHP development, dealing with special characters is a common problem, especially in string processing, special characters are often escaped. Among them, converting special characters into single quotes is a relatively common requirement, because in PHP, single quotes are a common way to wrap strings. In this article, we will explain how to handle special character conversion single quotes in PHP and provide specific code examples. In PHP, special characters include but are not limited to single quotes ('), double quotes ("), backslash (), etc. In strings
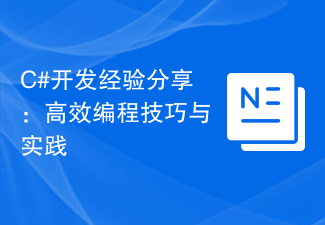
C# development experience sharing: efficient programming skills and practices In the field of modern software development, C# has become one of the most popular programming languages. As an object-oriented language, C# can be used to develop various types of applications, including desktop applications, web applications, mobile applications, etc. However, developing an efficient application is not just about using the correct syntax and library functions. It also requires following some programming tips and practices to improve the readability and maintainability of the code. In this article, I will share some C# programming

With the booming development of e-commerce, more and more companies are beginning to realize the importance of establishing their own e-commerce platform. As a developer, I was fortunate to participate in an e-commerce platform development project based on C#, and I would like to share some experiences and lessons with you. First, create a clear project plan. Before the project started, we spent a lot of time analyzing market needs and competitors, and determined the goals and scope of the project. The work at this stage is very important for subsequent development and implementation. It can help us better understand our customers.
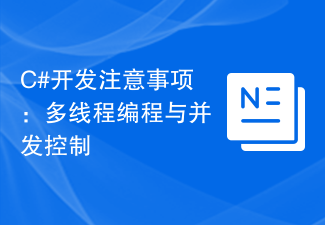
In C# development, multi-threaded programming and concurrency control are particularly important in the face of growing data and tasks. This article will introduce some matters that need to be paid attention to in C# development from two aspects: multi-threaded programming and concurrency control. 1. Multi-threaded programming Multi-threaded programming is a technology that uses multi-core resources of the CPU to improve program efficiency. In C# programs, multi-thread programming can be implemented using Thread class, ThreadPool class, Task class and Async/Await. But when doing multi-threaded programming
