How to drag and drop data in Vue technology development
How to drag and drop sort data in Vue technology development requires specific code examples
In modern Web development, drag and drop sorting is a common functional requirement . As a popular JavaScript framework, Vue provides a wealth of tools and functions to simplify the development of drag-and-drop sorting. This article will introduce how to use Vue technology to drag and drop data and provide some specific code examples.
First, we need to install Vue and some related dependencies, such as vue-draggable. In the Vue project, you can use npm or yarn to install:
npm install vue npm install vue-draggable
After the installation is complete, we can start writing code. Suppose we have a list to be sorted. Each item in the list has a unique id and a text content to be displayed. We can use a Vue component to render this list and handle the drag and drop sorting logic.
First, in the <template>
part of the Vue component, we can use the v-for
directive to traverse the list to be sorted, and use v The -bind
directive binds the id of each item to the data-id
attribute of the HTML element. In this way, during the dragging process, we can use this attribute to get the id of the dragged item.
<template> <div> <div v-for="item in items" :key="item.id" :data-id="item.id">{{ item.text }}</div> </div> </template>
Then, in the <script>
section of the Vue component, we can define the list to be sorted in the data
attribute and mounted
Use Sortable
in the life cycle hook to initialize the sortable list. Sortable
is a plug-in provided in the vue-draggable library, which can easily implement the drag-and-drop sorting function.
<script> import Sortable from 'sortablejs'; export default { data() { return { items: [ { id: 1, text: 'Item 1' }, { id: 2, text: 'Item 2' }, { id: 3, text: 'Item 3' }, { id: 4, text: 'Item 4' }, // ... ], }; }, mounted() { const container = document.querySelector('div'); // 或者使用其他合适的选择器指定容器 Sortable.create(container, { onEnd: this.handleDragEnd, }); }, methods: { handleDragEnd(event) { const draggedItemId = event.item.getAttribute('data-id'); // 根据拖拽的项的id进行一些排序逻辑 }, }, }; </script>
In the above code, we use the Sortable.create
method in the mounted
lifecycle hook to create a sortable list. We use the onEnd
event to listen for the drag end event, and call the handleDragEnd
method to handle the drag end logic. In the handleDragEnd
method, we can perform the necessary sorting logic based on the id of the dragged item.
When the user drags an item and releases it, the handleDragEnd
method will be called and an event
object will be passed in. The HTML element of the dragged item can be obtained through event.item
, and the id of the dragged item can be obtained through getAttribute('data-id')
.
Now, we have completed the basic settings of drag sorting. According to specific business needs, we can perform some sorting logic based on the ID of the dragged item, such as updating data or sending a request. It can be expanded according to the actual situation.
In summary, Vue technology provides a wealth of functions and tools to simplify the development of drag-and-drop sorting. We can use the vue-draggable library to quickly implement a sortable list, and use Vue's data binding and life cycle hooks to handle the drag and drop sorting logic. Through the above code examples, I believe readers will have a deeper understanding of how to drag and drop data in Vue technology.
The above is the detailed content of How to drag and drop data in Vue technology development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


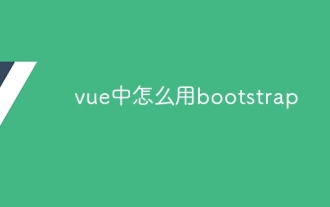
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
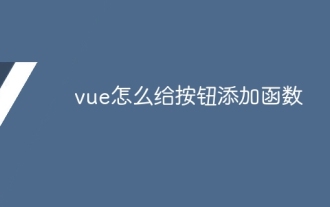
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
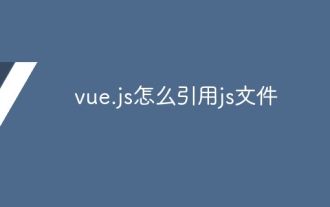
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
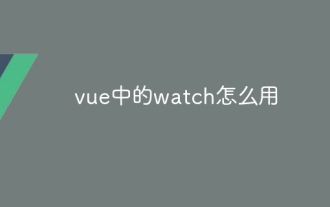
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
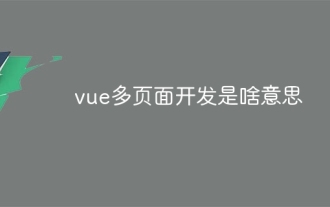
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
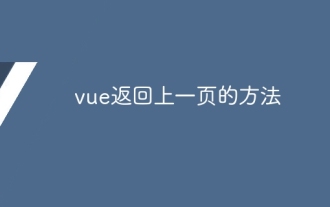
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
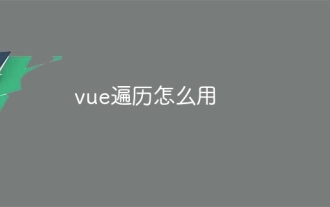
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
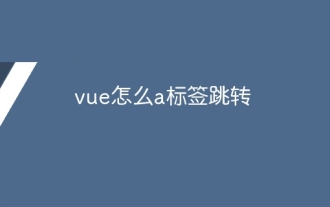
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
