


How to solve the problem of concurrent memory access conflicts in Go language?
How to solve the problem of concurrent memory access conflicts in Go language?
In the Go language, we can use goroutine to implement concurrent programming, which undoubtedly brings us more powerful performance and parallel processing capabilities. However, concurrent programming can also cause some problems, the most common of which is memory access conflicts.
The memory access conflict problem refers to the race condition that may result when multiple goroutines read and write shared variables at the same time. For example, data inconsistency occurs when two goroutines try to write to the same variable at the same time.
In order to solve the problem of concurrent memory access conflicts, the Go language provides some mechanisms. Below we will introduce several common methods.
1. Use mutex (mutex)
Mutex is a common concurrency control mechanism, which can ensure that only one goroutine can access shared variables at the same time. In Go language, we can use the Mutex structure in the sync package to implement a mutex lock.
The specific example code is as follows:
package main import ( "fmt" "sync" ) var count int var mutex sync.Mutex func increment() { mutex.Lock() defer mutex.Unlock() count++ } func main() { var wg sync.WaitGroup for i := 0; i < 1000; i++ { wg.Add(1) go func() { defer wg.Done() increment() }() } wg.Wait() fmt.Println("count:", count) }
In the above code, we define a global variable count and a mutex lock mutex. In the increment function, we use mutex.Lock() to acquire the lock and defer mutex.Unlock() to release the lock. This ensures that only one goroutine can modify the count variable at a time, thus avoiding memory access conflicts.
2. Use a read-write mutex (RWMutex)
The read-write mutex is a special mutex that allows multiple goroutines to read shared variables at the same time, but only Allows a goroutine to perform write operations. The sync package in Go language provides the RWMutex structure to implement read and write mutex locks.
The specific example code is as follows:
package main import ( "fmt" "sync" ) var count int var rwMutex sync.RWMutex func read() { rwMutex.RLock() defer rwMutex.RUnlock() fmt.Println("count:", count) } func increment() { rwMutex.Lock() defer rwMutex.Unlock() count++ } func main() { var wg sync.WaitGroup for i := 0; i < 10; i++ { wg.Add(1) go func() { defer wg.Done() read() }() } for i := 0; i < 1000; i++ { wg.Add(1) go func() { defer wg.Done() increment() }() } wg.Wait() }
In the above code, we define a global variable count and a read-write mutex rwMutex. In the read function, we use rwMutex.RLock() to acquire the read lock, and defer rwMutex.RUnlock() to release the read lock. This ensures that multiple goroutines can read the count variable at the same time. For the increment function, we use rwMutex.Lock() to acquire the write lock, and defer rwMutex.Unlock() to release the write lock. This ensures that only one goroutine can modify the count variable at a time, thus avoiding memory access conflicts.
3. Using channels
Channels are a mechanism used for communication between multiple goroutines in the Go language. By using channels, we can avoid explicitly adding shared variables. lock and unlock operations. When a goroutine needs to update a shared variable, it sends the data to the channel, and other goroutines get the latest value by receiving data from the channel.
The specific example code is as follows:
package main import ( "fmt" "sync" ) func increment(ch chan int, wg *sync.WaitGroup) { count := <-ch count++ ch <- count wg.Done() } func main() { ch := make(chan int, 1) var wg sync.WaitGroup wg.Add(1000) ch <- 0 for i := 0; i < 1000; i++ { go increment(ch, &wg) } wg.Wait() count := <-ch fmt.Println("count:", count) }
In the above code, we define a channel ch and a waiting group wg. In the increment function, we receive the value in the ch channel through
Summary:
By using methods such as mutex locks, read-write mutex locks, and channels, we can effectively solve the problem of concurrent memory access conflicts in the Go language. Different scenarios and needs may be suitable for different solutions, and developers need to choose the most appropriate method based on the specific situation. At the same time, these methods also need to pay attention to avoiding deadlock, livelock and other problems to ensure the correctness and performance of the program.
The above is the detailed content of How to solve the problem of concurrent memory access conflicts in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
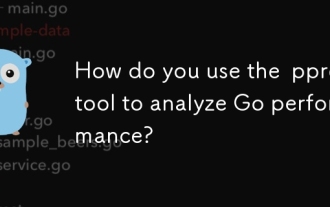
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
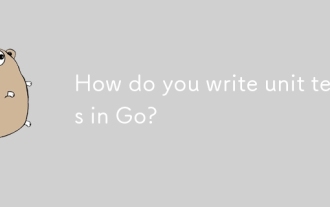
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
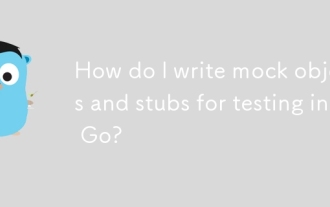
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
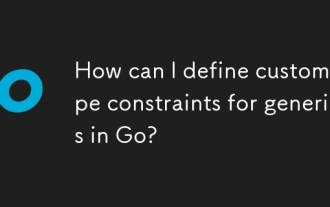
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
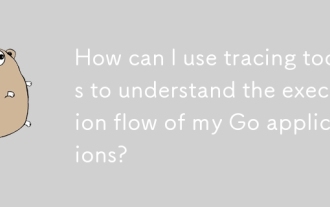
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
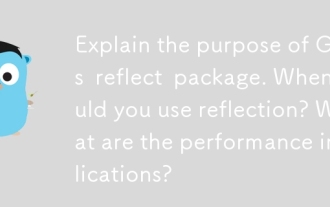
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
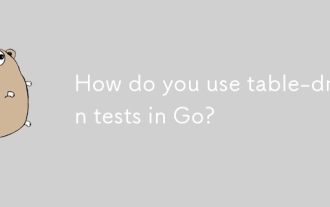
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
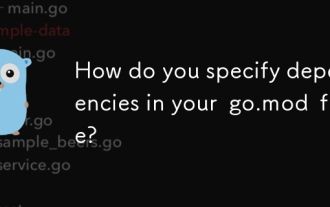
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
