


How to solve the error handling problem of concurrent requests in Go language?
How to solve the problem of error handling of concurrent requests in Go language?
When developing concurrent applications, we often need to send multiple concurrent requests to improve the concurrent performance of the program. However, when an error occurs in one of the requests, it becomes very important how to effectively catch and handle these errors.
Go language provides some technologies and patterns to solve the problem of error handling of concurrent requests. In this article, we will discuss several commonly used methods and provide code examples for better understanding.
- Use goroutine and channel for error transmission
Goroutine and channel are important features for concurrent programming in the Go language. You can use a goroutine to handle multiple requests in parallel in the background and use a channel to pass errors from the goroutine back to the main function.
Code example:
package main import ( "fmt" ) func fetchData(url string, ch chan<- error) { // 模拟请求数据 // 如果请求发生错误,将错误写入channel // 如果没有错误,写入nil到channel if err != nil { ch <- fmt.Errorf("fetch data error: %v", err) return } ch <- nil } func main() { urls := []string{"https://example.com", "https://google.com", "https://facebook.com"} ch := make(chan error) // 并发处理多个请求 for _, url := range urls { go fetchData(url, ch) } // 接收并处理错误 for range urls { if err := <-ch; err != nil { fmt.Println(err) } } }
In the above example, the fetchData function is used to simulate request data. If an error occurs in the request, the error is written to the channel; if there is no error, nil is written to the channel. In the main function, we create a channel to receive errors and use goroutine to handle multiple requests concurrently. Finally, we use a for loop to receive and handle each error.
- Use sync.WaitGroup to wait for all requests to complete
sync.WaitGroup is used to wait for a group of goroutines to complete their tasks. You can use a WaitGroup to wait for all concurrent requests to complete and handle errors in the main function.
Code example:
package main import ( "fmt" "sync" ) func fetchData(url string, wg *sync.WaitGroup, m *sync.Mutex, errors *[]error) { defer wg.Done() // 模拟请求数据 // 如果请求发生错误,将错误添加到errors切片(注意需要使用互斥锁保证并发安全) m.Lock() *errors = append(*errors, fmt.Errorf("fetch data error: %s", url)) m.Unlock() } func main() { urls := []string{"https://example.com", "https://google.com", "https://facebook.com"} var wg sync.WaitGroup var m sync.Mutex var errors []error // 增加等待的goroutine数量 wg.Add(len(urls)) // 并发处理多个请求 for _, url := range urls { go fetchData(url, &wg, &m, &errors) } // 等待所有goroutine完成 wg.Wait() // 处理错误 for _, err := range errors { fmt.Println(err) } }
In the above example, the fetchData function is used to simulate request data. If an error occurs with the request, add the error to the errors slice. Note that in order to ensure concurrency safety, we use a mutex m to ensure that access to the errors slice is thread-safe. In the main function, we use sync.WaitGroup to wait for all goroutines to complete and handle errors.
Summary:
The above two methods are common patterns for how to effectively capture and handle errors when multiple requests are processed concurrently. Using goroutines and channels makes it easy to propagate errors back to the main function and handle them appropriately. Using sync.WaitGroup can more flexibly control goroutine waiting and handle errors.
By using these methods, we can better manage error handling for concurrent requests, thereby improving application reliability and performance. Of course, in actual development, different modes and technologies may be selected to solve the problem of error handling of concurrent requests based on specific needs and scenarios.
The above is the detailed content of How to solve the error handling problem of concurrent requests in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


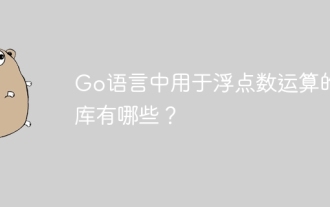
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
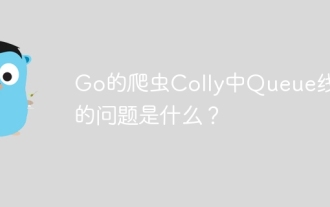
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
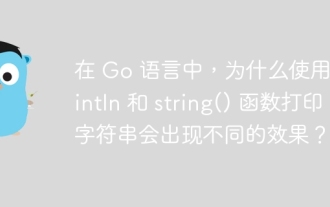
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
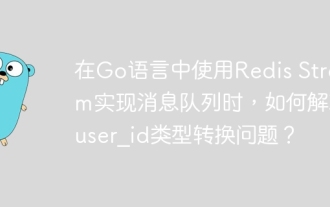
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
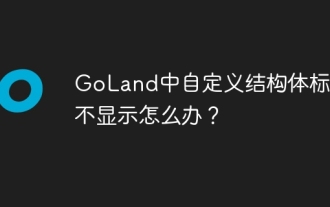
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
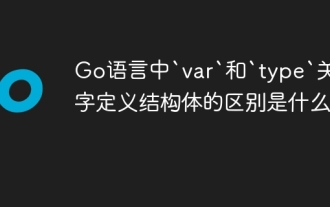
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
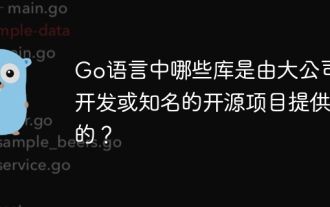
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
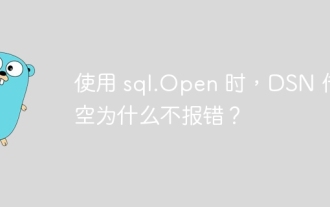
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
