


How to solve the problem of failure recovery of concurrent tasks in Go language?
How to solve the problem of failure recovery of concurrent tasks in Go language?
In modern software development, the use of concurrent processing can significantly improve the performance of the program. In the Go language, we can achieve efficient concurrent task processing by using goroutine and channel. However, concurrent tasks also bring some new challenges, such as handling failure recovery. This article will introduce some methods to solve the problem of concurrent task failure recovery in Go language and provide specific code examples.
- Error handling in concurrent tasks
When dealing with concurrent tasks, we often want to be able to detect and handle errors that may occur during task execution. In Go language, you can use Go statements and anonymous functions to create goroutines, and you can use the defer keyword to capture and handle errors that occur in goroutines.
func main() { // 创建一个带有缓冲的channel,用于接收任务的执行结果和错误信息 results := make(chan string, 10) // 启动多个goroutine,并行执行任务 for i := 0; i < 10; i++ { go func() { result, err := doTask() if err != nil { fmt.Println("Error:", err) results <- "" return } results <- result }() } // 等待所有任务执行完毕 for i := 0; i < 10; i++ { <-results } }
In the above sample code, we use a buffered channel to receive the task execution results and error information. Each goroutine will send execution results or error information to this channel. The main goroutine uses a for loop and the channel's receive operation to wait for all tasks to be completed.
- Failure recovery of concurrent tasks
In real applications, no matter how much we pay attention to the correctness of the program, failures cannot be completely avoided. When an error occurs in a goroutine, we may need to perform fault recovery, such as retry, rollback, etc. In Go language, we can use the recover function to capture and handle panic exceptions in goroutine, and then perform corresponding failure recovery operations.
func main() { // 创建一个带有缓冲的channel,用于接收任务的执行结果和错误信息 results := make(chan string, 10) // 启动多个goroutine,并行执行任务 for i := 0; i < 10; i++ { go func() { defer func() { if err := recover(); err != nil { fmt.Println("Panic:", err) // 进行故障恢复,如重试、回滚等操作 time.Sleep(time.Second) } }() result, err := doTask() if err != nil { fmt.Println("Error:", err) results <- "" return } results <- result }() } // 等待所有任务执行完毕 for i := 0; i < 10; i++ { <-results } }
In the above sample code, we added an anonymous function after the main function using the defer keyword. This anonymous function uses the recover function to capture and handle panic exceptions in goroutine, and perform corresponding failure recovery operations. In this example, we simply sleep for one second when a panic exception occurs to simulate failure recovery operations.
Summary
By using goroutine and channel, we can easily implement concurrent task processing in the Go language. In order to solve the problem of failure recovery in concurrent tasks, we can use error handling and panic/recover mechanisms to detect and handle errors and exceptions that may occur during task execution. Compared with other programming languages, the Go language provides a concise and powerful concurrent programming model, making it more convenient and efficient to solve the failure recovery problem of concurrent tasks.
The above is the detailed content of How to solve the problem of failure recovery of concurrent tasks in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


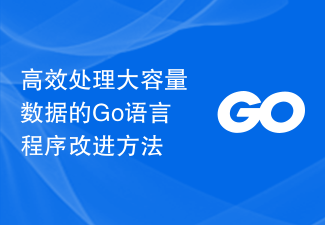
The method of optimizing Go language programs to process large-capacity data requires specific code examples. Overview: As the size of data continues to grow, large-scale data processing has become an important topic in modern software development. As an efficient and easy-to-use programming language, Go language can also well meet the needs of large-capacity data processing. This article will introduce some methods to optimize Go language programs to handle large volumes of data, and provide specific code examples. 1. Batch processing of data When processing large-capacity data, one of the common optimization methods is to use batch processing of data.
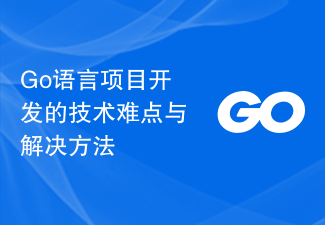
Technical Difficulties and Solutions in Go Language Project Development With the popularization of the Internet and the development of informatization, the development of software projects has received more and more attention. Among many programming languages, Go language has become the first choice of many developers because of its powerful performance, efficient concurrency capabilities and simple and easy-to-learn syntax. However, there are still some technical difficulties in the development of Go language projects. This article will explore these difficulties and provide corresponding solutions. 1. Concurrency control and race conditions The concurrency model of Go language is called "goroutine", which makes
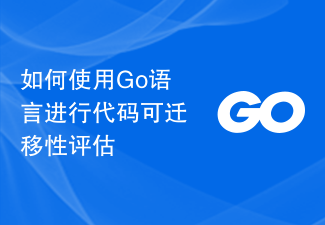
How to use Go language to evaluate code portability Introduction: With the development of software development, code portability has gradually become an important issue that program developers pay attention to. In the process of software development, in order to improve efficiency, reduce costs, and cope with multi-platform requirements, we often need to migrate code to different target environments. For Go language developers, some features of the Go language make it an ideal choice because the Go language has excellent portability and scalability. This article will introduce how to use Go language
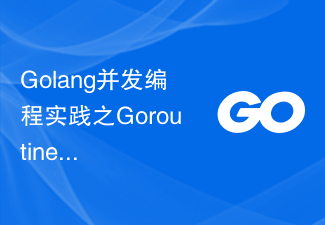
Introduction to the application scenario analysis of Goroutines in Golang concurrent programming practice: With the continuous improvement of computer performance, multi-core processors have become mainstream. In order to make full use of the advantages of multi-core processors, we need to use concurrent programming technology to implement multi-threaded operations. In the Go language, Goroutines (coroutines) are a very powerful concurrent programming mechanism that can be used to achieve efficient concurrent operations. In this article, we will explore the application scenarios of Goroutines and give some examples.
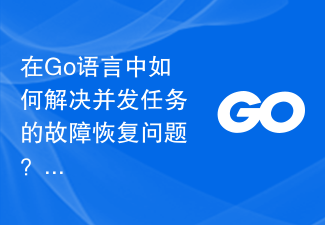
How to solve the problem of failure recovery of concurrent tasks in Go language? In modern software development, the use of concurrent processing can significantly improve the performance of the program. In the Go language, we can achieve efficient concurrent task processing by using goroutine and channels. However, concurrent tasks also bring some new challenges, such as handling failure recovery. This article will introduce some methods to solve the problem of concurrent task failure recovery in Go language and provide specific code examples. Error handling in concurrent tasks When processing concurrent tasks,
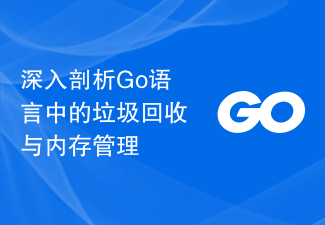
In-depth analysis of garbage collection and memory management in Go language 1. Introduction With the development of technology, the needs of software development have become more and more complex, and the performance and efficiency of programs have also become the focus of developers. For a programming language, efficient garbage collection and memory management are key to ensuring stable program performance. As an open source programming language, Go language is popular among many developers for its simplicity, efficiency and concurrency. This article will provide an in-depth analysis of the garbage collection and memory management mechanism in the Go language, and explain it through specific code examples.
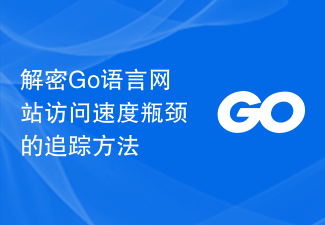
Deciphering the tracking method of Go language website access speed bottleneck Introduction: In the Internet era, website access speed is one of the important factors of user experience. When access to a website is slow, users tend to feel impatient and even give up access. Therefore, understanding and solving access speed bottlenecks has become one of the essential skills for developers. This article will introduce how to use Go language to track and solve website access speed bottlenecks. 1. Understand the causes of access speed bottlenecks. Before we start to solve the access speed bottleneck problem, we first need to understand the occurrence of bottlenecks.
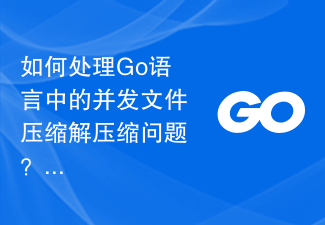
How to deal with concurrent file compression and decompression in Go language? File compression and decompression is one of the tasks frequently encountered in daily development. As file sizes increase, compression and decompression operations can become time-consuming, so concurrency becomes an important means of improving efficiency. In the Go language, you can use the features of goroutine and channel to implement concurrent processing of file compression and decompression operations. File compression First, let's take a look at how to implement file compression in the Go language. Go language standard
