


How to deal with memory allocation and garbage collection issues in C# development
How to deal with memory allocation and garbage collection issues in C# development
In C# development, memory allocation and garbage collection are very important issues. Proper handling of memory allocation and garbage collection can improve program performance and stability. This article will introduce some common techniques for dealing with memory allocation and garbage collection, and provide specific code examples.
- Avoid frequent object creation and destruction
Frequent object creation and destruction will cause the garbage collection mechanism to start frequently, thereby reducing the performance of the program. We can use object pools to manage commonly used objects to avoid frequent creation and destruction.
public class ObjectPool<T> where T : new() { private readonly Stack<T> _pool; public ObjectPool() { _pool = new Stack<T>(); } public T GetObject() { if(_pool.Count > 0) { return _pool.Pop(); } return new T(); } public void ReleaseObject(T item) { _pool.Push(item); } }
Using the object pool can reuse objects, avoid frequent creation and destruction, and improve program performance.
- Use the using statement to release resources
When dealing with some objects that require manual release of resources, we must ensure that resources are released in time to prevent resource leaks. You can use the using statement to automatically release resources.
public void ProcessFile(string filePath) { using (FileStream fileStream = new FileStream(filePath, FileMode.Open)) { // 处理文件流 } }
Using the using statement can ensure that resources are released immediately after use to avoid resource leaks.
- Manually release unmanaged resources
Some objects involve unmanaged resources, such as using Win32 API or COM components. In this case, unmanaged resources need to be released manually to avoid memory leaks.
public class UnmanagedResource : IDisposable { private IntPtr _handle; public UnmanagedResource() { _handle = // 初始化非托管资源的句柄 } // 手动释放非托管资源 protected virtual void Dispose(bool disposing) { if (disposing) { // 释放托管资源 } // 释放非托管资源 // 使用Win32 API或者COM组件来释放资源 } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ~UnmanagedResource() { Dispose(false); } }
Manually release unmanaged resources in the Dispose method, and call the Dispose method when the object is destroyed through the destructor.
- Minimize the use of the Finalize method
The Finalize method is a method used for garbage collection, but the cost of triggering the Finalize method is very high, which will cause the garbage collection mechanism to fail. Performance degrades. So under normal circumstances, try to avoid using the Finalize method. Only use the Finalize method when you really need to do some resource cleanup work.
- Garbage collection control
In C#, we can use the GC class to control garbage collection. For example, manually call the GC.Collect method to perform garbage collection immediately.
// 当前代已使用的内存超过85%,则进行垃圾回收 if (GC.GetTotalMemory(false) > 0.85 * GC.GetTotalMemory(true)) { GC.Collect(); }
It should be noted that excessive use of the GC.Collect method will lead to frequent garbage collection and reduce program performance. Therefore, we must use the related methods of the GC class with caution.
To sum up, dealing with memory allocation and garbage collection issues is very important for C# development. By using object pools, using using statements to release resources, manually releasing unmanaged resources, reducing the use of the Finalize method, and reasonably controlling garbage collection, the performance and stability of the program can be improved.
Reference:
- Microsoft Docs: https://docs.microsoft.com/en-us/dotnet/standard/garbage-collection/
The above is the detailed content of How to deal with memory allocation and garbage collection issues in C# development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
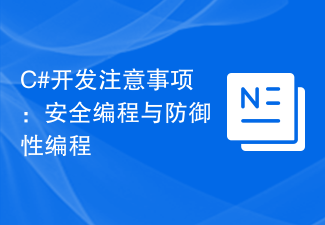
C# is a widely used object-oriented programming language that is easy to learn, strongly typed, safe, reliable, efficient and has high development efficiency. However, C# programs may still be subject to malicious attacks or program errors caused by unintentional negligence. When writing C# programs, we should pay attention to the principles of safe programming and defensive programming to ensure the safety, reliability, and stability of the program. 1. Principles of secure programming 1. Do not trust user input. If there is insufficient verification in a C# program, malicious users can easily enter malicious data and attack the program.
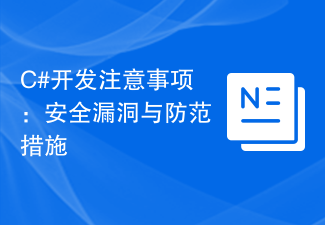
C# is a programming language widely used on Windows platforms. Its popularity is inseparable from its powerful functions and flexibility. However, precisely because of its wide application, C# programs also face various security risks and vulnerabilities. This article will introduce some common security vulnerabilities in C# development and discuss some preventive measures. Input validation of user input is one of the most common security holes in C# programs. Unvalidated user input may contain malicious code, such as SQL injection, XSS attacks, etc. To protect against such attacks, all
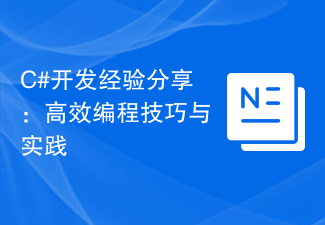
C# development experience sharing: efficient programming skills and practices In the field of modern software development, C# has become one of the most popular programming languages. As an object-oriented language, C# can be used to develop various types of applications, including desktop applications, web applications, mobile applications, etc. However, developing an efficient application is not just about using the correct syntax and library functions. It also requires following some programming tips and practices to improve the readability and maintainability of the code. In this article, I will share some C# programming
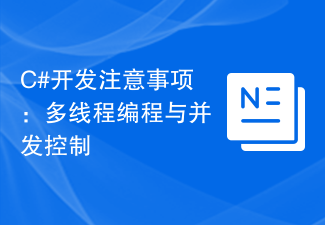
In C# development, multi-threaded programming and concurrency control are particularly important in the face of growing data and tasks. This article will introduce some matters that need to be paid attention to in C# development from two aspects: multi-threaded programming and concurrency control. 1. Multi-threaded programming Multi-threaded programming is a technology that uses multi-core resources of the CPU to improve program efficiency. In C# programs, multi-thread programming can be implemented using Thread class, ThreadPool class, Task class and Async/Await. But when doing multi-threaded programming
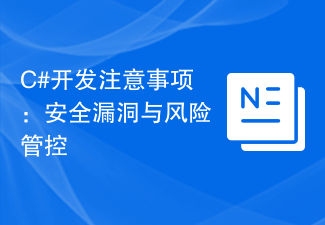
C# is a commonly used programming language in many modern software development projects. As a powerful tool, it has many advantages and applicable scenarios. However, developers should not ignore software security considerations when developing projects using C#. In this article, we will discuss the security vulnerabilities and risk management and control measures that need to be paid attention to during C# development. 1. Common C# security vulnerabilities: SQL injection attack SQL injection attack refers to the process in which an attacker manipulates the database by sending malicious SQL statements to the web application. for
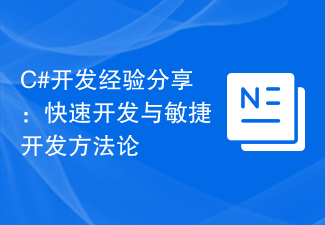
In the process of C# development, rapid development and agile development methodologies are very important, especially in today's rapidly changing market. In this article, I will share my C# development experience, focusing on rapid development and agile development methodologies. 1. What is rapid development? Rapid development is to respond quickly to market demand so that products can be launched as early as possible. This development method can greatly shorten the project development cycle, reduce costs, and enable rapid iterative development based on user needs. Rapid development requires some specific technical means, such as using
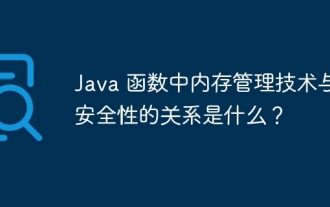
Memory management in Java involves automatic memory management, using garbage collection and reference counting to allocate, use and reclaim memory. Effective memory management is crucial for security because it prevents buffer overflows, wild pointers, and memory leaks, thereby improving the safety of your program. For example, by properly releasing objects that are no longer needed, you can avoid memory leaks, thereby improving program performance and preventing crashes.
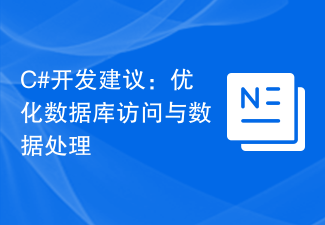
C# development suggestions: Optimize database access and data processing In modern software development, database access and data processing are an indispensable part. Especially in C# development, optimizing database access and data processing is the key to improving software performance and user experience. This article will discuss database access and data processing optimization in C# development, and provide developers with better guidance and suggestions. 1. Use appropriate database access technology. In C# development, common database access technologies include ADO.NET, EntityFr
