


Python problems encountered in multi-threaded programming and their solutions
Python problems and solutions encountered in multi-threaded programming
When doing multi-threaded programming, we often encounter issues related to thread synchronization, resource competition and Deadlock and other related issues. This article will introduce some common Python multi-threaded programming problems and provide corresponding solutions and code examples.
- Thread synchronization issues
Multiple threads may access shared resources at the same time, resulting in data inconsistency or errors. In order to solve this problem, we can use mechanisms such as thread locks or condition variables to achieve thread synchronization. The following is a code example that uses thread locks to solve thread synchronization problems:
import threading count = 0 lock = threading.Lock() def increase(): global count with lock: count += 1 threads = [] for _ in range(10): t = threading.Thread(target=increase) threads.append(t) t.start() for t in threads: t.join() print(count) # 输出 10
In the above example, we define a global variable count
, and then use threading.Lock
Created a thread locklock
. In the increase
function, we use the context manager with
to obtain the thread lock lock
to ensure that only one thread can modify the count## at a time # Variables. Finally, we created 10 threads to call the
increase function and waited for all threads to finish executing to output the value of
count.
- Resource competition problem
import threading count = 0 lock = threading.RLock() def increase(): global count with lock: count += 1 threads = [] for _ in range(10): t = threading.Thread(target=increase) threads.append(t) t.start() for t in threads: t.join() print(count) # 输出 10
threading.RLocklock
, it can be acquired multiple times by the same thread without causing deadlock. In the
increase function, we use the context manager
with to obtain the mutex lock
lock to ensure that only one thread can modify the
count# at a time ## Variables. Finally, we created 10 threads to call the increase
function and waited for all threads to finish executing to output the value of count
.
- Deadlock refers to a problem in which multiple threads wait for each other to release resources, causing the program to be unable to continue execution. In order to avoid deadlock, we need to reasonably design the resource dependencies between threads to avoid forming circular dependencies. The following is a code example that uses resource request order to solve the deadlock problem:
import threading lock1 = threading.Lock() lock2 = threading.Lock() def thread1(): lock1.acquire() lock2.acquire() print("Thread 1") lock2.release() lock1.release() def thread2(): lock2.acquire() lock1.acquire() print("Thread 2") lock1.release() lock2.release() t1 = threading.Thread(target=thread1) t2 = threading.Thread(target=thread2) t1.start() t2.start() t1.join() t2.join()
In the above example, we defined two mutex locks
lock1 and lock2
, and then acquire these two locks in the same order in the thread1
and thread2
functions to ensure that the order of resource requests between threads is consistent. Finally, we create two threads to call the thread1
and thread2
functions, and wait for the two threads to finish executing before ending the program. Summary:
When doing Python multi-threaded programming, we often encounter problems such as thread synchronization, resource competition, and deadlock. In order to solve these problems, we can use mechanisms such as thread locks, mutex locks, and resource request sequences to achieve thread synchronization and resource management. By properly designing the resource dependencies between threads, we can avoid some common problems in multi-threaded programming and ensure the correctness and stability of the program.
The above is the detailed content of Python problems encountered in multi-threaded programming and their solutions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
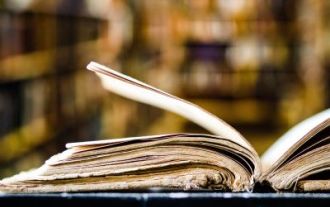
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
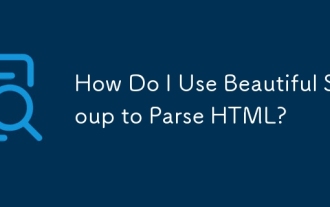
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
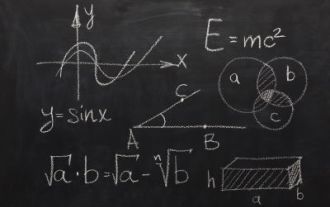
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
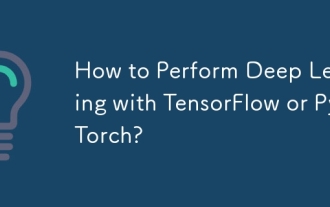
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
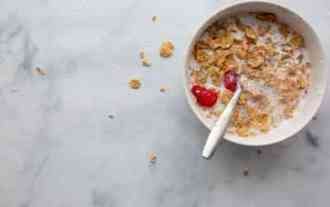
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
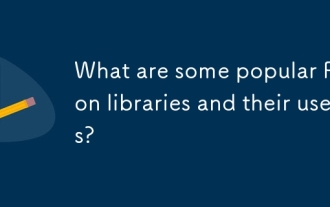
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H

This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
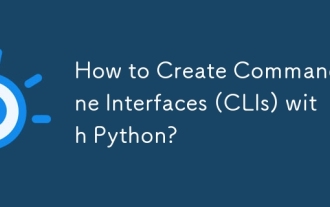
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
