


Common network communication and security problems and solutions in C#
Common network communication and security problems and solutions in C
#In today's Internet era, network communication has become an indispensable part of software development. In C#, we usually encounter some network communication problems, such as data transmission security, network connection stability, etc. This article will discuss in detail common network communication and security issues in C# and provide corresponding solutions and code examples.
1. Network communication problems
- Interruption of network connection:
During network communication, interruption of network connection may occur, which will lead to interruption and incomplete data transmission. . In order to solve this problem, we can use the TCP protocol in C# to establish a stable connection, while performing error detection and data retransmission during the transmission process.
The following is a sample code to establish a TCP connection and transmit data:
using System; using System.Net.Sockets; using System.Text; public class TCPClientExample { public static void Main() { try { // 创建TCP客户端 TcpClient client = new TcpClient(); // 连接到服务器 client.Connect("serverIP", serverPort); // 获取网络流 NetworkStream networkStream = client.GetStream(); // 发送数据 string message = "Hello Server!"; byte[] data = Encoding.UTF8.GetBytes(message); networkStream.Write(data, 0, data.Length); // 接收数据 byte[] buffer = new byte[1024]; int bytesRead = networkStream.Read(buffer, 0, buffer.Length); string response = Encoding.UTF8.GetString(buffer, 0, bytesRead); Console.WriteLine("Server Response: " + response); // 关闭连接 networkStream.Close(); client.Close(); } catch (Exception ex) { Console.WriteLine(ex.ToString()); } } }
- Efficiency of data transmission:
In network communication, the efficiency of data transmission often decreases is limited, especially when the data size is large. In order to improve the efficiency of data transmission, we can use data compression and streaming.
The following is a sample code that uses the Gzip data compression algorithm for data transmission:
using System; using System.IO; using System.IO.Compression; using System.Net; using System.Net.Sockets; using System.Text; public class TCPClientExample { public static void Main() { try { // 创建TCP客户端 TcpClient client = new TcpClient(); // 连接到服务器 client.Connect("serverIP", serverPort); // 获取网络流 NetworkStream networkStream = client.GetStream(); // 发送数据 string message = "Hello Server!"; byte[] data = Encoding.UTF8.GetBytes(message); byte[] compressedData; using (MemoryStream ms = new MemoryStream()) { using (GZipStream gzipStream = new GZipStream(ms, CompressionMode.Compress)) { gzipStream.Write(data, 0, data.Length); } compressedData = ms.ToArray(); } networkStream.Write(compressedData, 0, compressedData.Length); // 接收数据 byte[] buffer = new byte[1024]; int bytesRead = networkStream.Read(buffer, 0, buffer.Length); byte[] decompressedData; using (MemoryStream ms = new MemoryStream(buffer, 0, bytesRead)) { using (GZipStream gzipStream = new GZipStream(ms, CompressionMode.Decompress)) { using (MemoryStream decompressedMs = new MemoryStream()) { gzipStream.CopyTo(decompressedMs); decompressedData = decompressedMs.ToArray(); } } } string response = Encoding.UTF8.GetString(decompressedData); Console.WriteLine("Server Response: " + response); // 关闭连接 networkStream.Close(); client.Close(); } catch (Exception ex) { Console.WriteLine(ex.ToString()); } } }
2. Security issues
- Security of data transmission :
In network communication, the security of data transmission is very important. We need to take some security measures to protect the confidentiality and integrity of the data. A common solution is to use the SSL/TLS protocol for encrypted communication.
The following is a sample code that uses SslStream for encrypted communication:
using System; using System.Net.Security; using System.Net.Sockets; using System.Security.Authentication; using System.Security.Cryptography.X509Certificates; using System.Text; public class SSLClientExample { public static void Main() { try { // 创建TCP客户端 TcpClient client = new TcpClient(); // 连接到服务器 client.Connect("serverIP", serverPort); // 创建SslStream SslStream sslStream = new SslStream(client.GetStream(), false, new RemoteCertificateValidationCallback(ValidateServerCertificate), null); // 进行SSL握手 sslStream.AuthenticateAsClient("serverName"); // 发送数据 string message = "Hello Server!"; byte[] data = Encoding.UTF8.GetBytes(message); sslStream.Write(data, 0, data.Length); // 接收数据 byte[] buffer = new byte[1024]; int bytesRead = sslStream.Read(buffer, 0, buffer.Length); string response = Encoding.UTF8.GetString(buffer, 0, bytesRead); Console.WriteLine("Server Response: " + response); // 关闭连接 sslStream.Close(); client.Close(); } catch (Exception ex) { Console.WriteLine(ex.ToString()); } } // 验证服务器证书 private static bool ValidateServerCertificate(object sender, X509Certificate certificate, X509Chain chain, SslPolicyErrors sslPolicyErrors) { // 验证证书的合法性 if (sslPolicyErrors == SslPolicyErrors.None) return true; // 验证证书的合法性失败 Console.WriteLine("Certificate error: {0}", sslPolicyErrors); // 可以选择忽略证书验证 // return true; return false; } }
- Cross-domain request problem:
In web development, cross-domain requests are a common security issues. In order to solve the cross-domain request problem, we can set the CORS (Cross-Origin Resource Sharing) policy on the server side to allow specific cross-domain requests.
The following is a sample code for setting a CORS policy using ASP.NET Web API:
using System.Web.Http; using System.Web.Http.Cors; public class MyWebApiController : ApiController { [EnableCors(origins: "http://clientDomain", headers: "*", methods: "*")] public IHttpActionResult Get() { // 处理请求 return Ok(); } }
The above are some examples of common network communication and security problems and solutions in C# code. By using these solutions, we can ensure data integrity and security during network communication and improve the efficiency of data transmission. Of course, in practical applications, we need to select and adjust according to specific needs and scenarios. I hope this article can be helpful to everyone in dealing with network communication and security issues in C#!
The above is the detailed content of Common network communication and security problems and solutions in C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


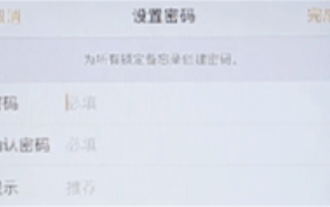
In Apple mobile phones, users can encrypt photo albums according to their own needs. Some users don't know how to set it up. You can add the pictures that need to be encrypted to the memo, and then lock the memo. Next, the editor will introduce the method of setting up the encryption of mobile photo albums for users. Interested users, come and take a look! Apple mobile phone tutorial How to set up iPhone photo album encryption A: After adding the pictures that need to be encrypted to the memo, go to lock the memo for detailed introduction: 1. Enter the photo album, select the picture that needs to be encrypted, and then click [Add to] below. 2. Select [Add to Notes]. 3. Enter the memo, find the memo you just created, enter it, and click the [Send] icon in the upper right corner. 4. Click [Lock Device] below
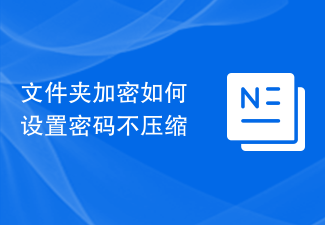
Folder encryption is a common data protection method that encrypts the contents of a folder so that only those who have the decryption password can access the files. When encrypting a folder, there are some common ways to set a password without compressing the file. First, we can use the encryption function that comes with the operating system to set a folder password. For Windows users, you can set it up by following the following steps: Select the folder to be encrypted, right-click the folder, and select "Properties"
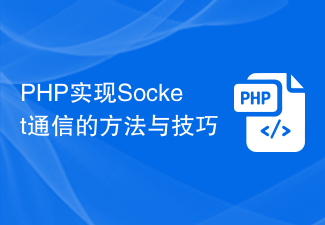
PHP is a commonly used development language that can be used to develop various web applications. In addition to common HTTP requests and responses, PHP also supports network communication through Sockets to achieve more flexible and efficient data interaction. This article will introduce the methods and techniques of how to implement Socket communication in PHP, and attach specific code examples. What is Socket Communication Socket is a method of communication in a network that can transfer data between different computers. by S
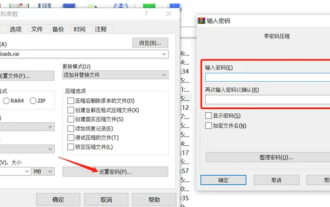
The editor will introduce to you three methods of encryption and compression: Method 1: Encryption The simplest encryption method is to enter the password you want to set when encrypting the file, and the encryption and compression are completed. Method 2: Automatic encryption Ordinary encryption method requires us to enter a password when encrypting each file. If you want to encrypt a large number of compressed packages and the passwords are the same, then we can set automatic encryption in WinRAR, and then just When compressing files normally, WinRAR will add a password to each compressed package. The method is as follows: Open WinRAR, click Options-Settings in the setting interface, switch to [Compression], click Create Default Configuration-Set Password Enter the password we want to set here, click OK to complete the setting, we only need to correct
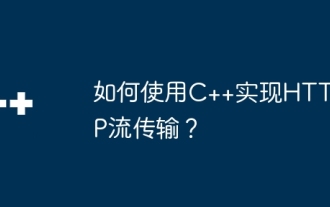
How to implement HTTP streaming in C++? Create an SSL stream socket using Boost.Asio and the asiohttps client library. Connect to the server and send an HTTP request. Receive HTTP response headers and print them. Receives the HTTP response body and prints it.
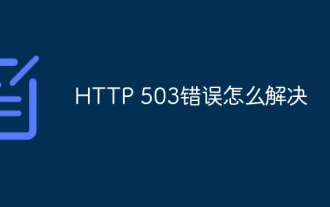
Solution: 1. Retry: You can wait for a period of time and try again, or refresh the page; 2. Check the server load: Check the server's CPU, memory and disk usage. If the capacity limit is exceeded, you can try to optimize the server configuration or increase the capacity. Server resources; 3. Check server maintenance and upgrades: You can only wait until the server returns to normal; 4. Check network connection: Make sure the network connection is stable, check whether the network device, firewall or proxy settings are correct; 5. Ensure cache or CDN configuration Correct; 6. Contact the server administrator, etc.
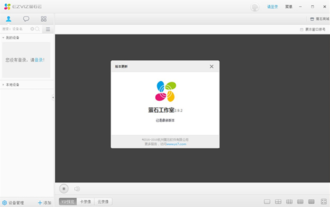
How to de-encrypt videos on EZVIZ Cloud: There are many ways to de-encrypt videos on EZVIZ Cloud, one of which is by using the EZVIZ Cloud Mobile App. Users only need to enter the device list, select the camera to be decrypted and enter the device details page. On the device details page, find the "Settings" option, and then select "Video Encryption" to make relevant settings. In the video encryption settings interface, you can choose the option to turn off video encryption, and save the settings to complete the decryption operation. This simple step allows users to easily decrypt videos and improves the convenience of using the camera. If you use the computer client of EZVIZ Cloud, you can also cancel video encryption through similar steps. Just log in and select the corresponding camera, enter the device details interface, and then look for video addition in the settings.

Original author: Meteor, ChainCatcher Original editor: Marco, ChainCatcher Recently, the full-chain interoperability protocol Analog has entered the public eye with the disclosure of US$16 million in financing. Investment institutions include TribeCapital, NGCVentures, Wintermute, GSR, NEAR, OrangeDAO, and Mike Novogratz’s Alternative asset management companies Samara Asset Group, Balaji Srinivasan, etc. At the end of 2023, Analog caused some excitement in the industry. They released information on the open testnet registration event on the X platform.
