


How to solve the problem of concurrent task reordering in Go language?
How to solve the problem of concurrent task reordering in Go language?
In concurrent programming, the execution order of tasks is often uncertain, which may cause some problems, especially for tasks with dependencies. In Go language, we can solve the problem of concurrent task reordering by using channels and coroutines. Below we will explain in detail how to achieve this.
Normally, we use channels to achieve task synchronization and communication. In the Go language, channels can be used as a higher-level synchronization primitive to ensure the execution order of tasks. By using buffered channels, we can start tasks in a coroutine and control the order in which tasks are executed.
First, we define a buffered channel to store the task and pass the channel as a parameter to the task execution function. The task execution function will read the task from the channel and perform the corresponding operations.
func worker(tasks <-chan int, results chan<- int) { for task := range tasks { // 执行任务操作 result := doWork(task) // 将结果发送到结果通道 results <- result } }
Next, we create a main function to control the execution order of tasks. First, we create a task channel and a result channel, and start multiple coroutines to perform tasks.
func main() { // 创建任务通道和结果通道 tasks := make(chan int, 100) results := make(chan int, 100) // 启动多个协程来执行任务 for i := 0; i < 5; i++ { go worker(tasks, results) } // 发送任务到任务通道中 for i := 0; i < 100; i++ { tasks <- i } // 关闭任务通道(以便通知协程任务已经完成) close(tasks) // 接收结果,保证任务的执行顺序 for i := 0; i < 100; i++ { result := <-results // 处理结果 handleResult(result) } }
In this example, we create a buffered task channel and result channel. Then, we started five coroutines (ie, task execution function workers) to execute the task. We send 100 tasks into the task channel. By closing the task channel, we notify the coroutine that the task has completed. Finally, we receive the results from the result channel in the order in which the tasks were sent, and process the corresponding results.
By using channels and coroutines, we can ensure the execution order of concurrent tasks and solve the problem of concurrent task reordering. In actual applications, we can adjust the number of concurrent tasks and the size of the buffer according to actual needs to obtain better performance.
To sum up, by using channels and coroutines, we can solve the problem of concurrent task reordering in the Go language. This approach allows us to safely execute dependent tasks and maintain the order of task execution. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of How to solve the problem of concurrent task reordering in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


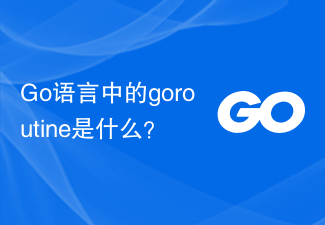
Go language is an open source programming language developed by Google and launched in 2009. This language has attracted more and more attention in recent years and is widely used in the development of network services, cloud computing and other fields. One of the most distinctive features of the Go language is its built-in goroutine (coroutine), a lightweight thread that can easily implement concurrent and parallel computing in code. So what exactly is goroutine? Simply put, goroutine is the Go language

Difference: 1. Goroutine communicates through channels, and coroutine communicates through yield and recovery operations. 2. Goroutine coroutines are not completely synchronized and can be run in parallel using multiple cores; coroutine coroutines are completely synchronized and will not run in parallel. 3. Goroutine can switch between multiple coroutines/threads; coroutine runs in one thread. 4. The application occupies a large amount of CPU for a long time. Users in goroutine have the right to terminate this task, but coroutine does not.
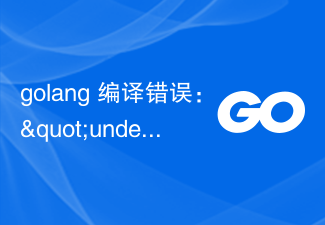
Compilation errors are a very common problem during development using golang. When you encounter the error: "undefined: sync.Mutex", it means that you are trying to use a type called sync.Mutex, which is not imported and declared correctly. So how to solve this problem? First, we need to understand what sync.Mutex is. sync.Mutex is a lock type in the golang standard library, which is used to implement mutually exclusive access to critical sections. in g
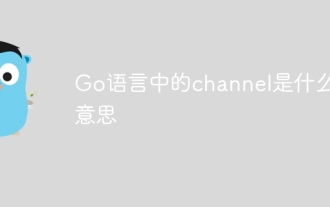
Channel in Go language is a mechanism for communication and data synchronization between coroutines. Can be thought of as a special data type, similar to a queue or pipe, used to transfer data between different coroutines. Channel provides two main operations: send and receive. Both send and receive operations in a channel are blocking, which means that if no sender or receiver is ready, the operation will be blocked until a coroutine is ready to perform the corresponding operation, etc.
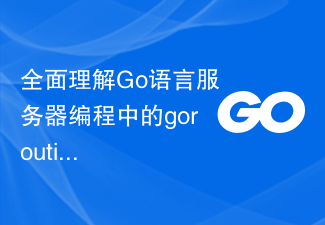
In Go language, we can use goroutine to execute tasks concurrently, which is an important feature that distinguishes Go language from other languages. Goroutine can be understood as a lightweight thread that can run on one or multiple threads at the same time. The concurrency model of Go language is based on the CSP (CommunicatingSequentialProcesses) model. This means that goroutines communicate with each other through channels rather than shared memory. this model

Go language uses channels and goroutines to communicate. After creating the channel, the goroutine can pass

How to solve the problem of concurrent task reordering in Go language? In concurrent programming, the execution order of tasks is often uncertain, which may cause some problems, especially for tasks with dependencies. In Go language, we can solve the problem of concurrent task reordering by using channels and coroutines. Below we will explain in detail how to achieve this. Typically, we use channels to achieve task synchronization and communication. In the Go language, channels can be used as a higher-level synchronization primitive to ensure the execution order of tasks. By using buffered
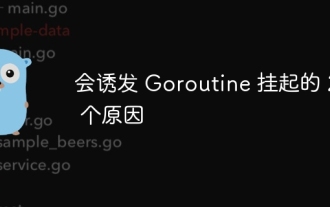
Today’s article will take a look at the 27 causes of gopark. For the convenience of reading, we will explain according to categories.
