


How to deal with file reading and writing problems in Python
How to handle file reading and writing in Python requires specific code examples
In Python, file reading and writing is a common operation task. Whether processing text files or binary files, Python provides powerful and flexible file reading and writing functions. This article will introduce how to handle file reading and writing in Python and give specific code examples.
1. File reading operation
- Open the file
In Python, use the open() function to open the file. The open() function accepts two parameters: file name and open mode. There are many options for opening modes, the commonly used ones are:
- 'r': read-only mode, only reading operations can be performed after opening the file.
- 'w': Write mode, if the file exists, clear the file content; if the file does not exist, create a new file.
- 'a': append mode, if the file exists, append to the end of the file; if the file does not exist, create a new file.
Sample code:
file = open("file.txt", "r")
- Read file content
There are many ways to read file content, the most common is to use The read() method can be used to read the entire file content at once, or the readline() method can be used to read the file content line by line.
Sample code (read the entire file content at once):
content = file.read()
Sample code (read the file content line by line):
line = file.readline() while line: print(line) line = file.readline()
- Close the file
After reading the file, be sure to remember to close the file. Closing a file frees system resources and ensures that the file is saved and consistent.
Sample code:
file.close()
2. File write operation
- Open file
Similar to file read operation, use open( ) function to open the file. But when opening a file, you need to specify the opening mode as 'w' or 'a'.
Sample code (write mode):
file = open("file.txt", "w")
- Write file content
There are many ways to write file content, the most common Use the write() method to write string content. You can also use the writelines() method to write multiple lines of content.
Sample code (write single line content):
file.write("Hello, World!")
Sample code (write multiple lines content):
lines = ["Line 1 ", "Line 2 ", "Line 3 "] file.writelines(lines)
- Close the file
After writing the file, be sure to remember to close the file. Closing a file frees up system resources and ensures that the file is saved and consistent.
Sample code:
file.close()
3. Exception handling
During the file reading and writing process, some abnormal situations may occur, such as the file does not exist, file permissions are insufficient, etc. In order to ensure the stability of the program, you can use the try-except statement to catch and handle these exceptions.
Sample code:
try: file = open("file.txt", "r") # 文件读取操作 except FileNotFoundError: print("文件不存在!") except PermissionError: print("权限不足!") finally: file.close()
4. Summary
Through the introduction of this article, we have learned how to handle file reading and writing in Python. Use the open() function to open the file and select the appropriate opening mode when needed. When reading a file, you can read the entire file at once or read the file line by line. When writing to a file, you can use the write() method to write string content or the writelines() method to write multi-line content. Finally, don't forget to close the file and use exception handling mechanisms to catch and handle exceptions. I hope this article will help you deal with file reading and writing problems in Python!
The above is the detailed content of How to deal with file reading and writing problems in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


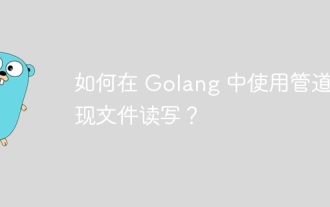
File reading and writing through pipes: Create a pipe to read data from the file and pass it through the pipe Receive the data from the pipe and process it Write the processed data to the file Use goroutines to perform these operations concurrently to improve performance
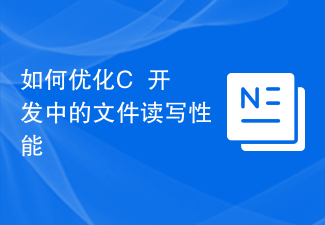
How to optimize file reading and writing performance in C++ development. In the C++ development process, file reading and writing operations are one of the common tasks. However, since file reading and writing are disk IO operations, they are more time-consuming than memory IO operations. In order to improve the performance of the program, we need to optimize file read and write operations. This article will introduce some common optimization techniques and suggestions to help developers improve performance during C++ file reading and writing. Use appropriate file reading and writing methods. In C++, file reading and writing can be achieved in a variety of ways, such as C-style file IO.
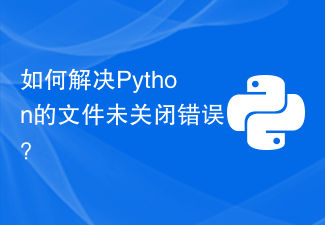
Python is a high-level programming language that is widely used in fields such as data science and artificial intelligence. In Python programming, we often encounter file not closed errors, which may cause program crashes, data loss and other problems, so solving file not closed errors is an essential skill in Python programming. This article will explain how to solve Python's file not closed error. 1. What is a file not closed error? In Python, you need to use the open() function when opening a file.
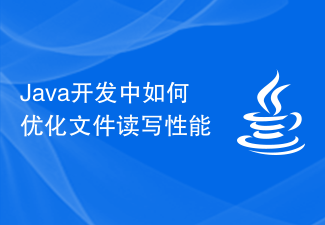
Java is a programming language widely used in software development and is highly portable and flexible. In the Java development process, file reading and writing operations are one of the most common tasks. However, the performance of file reading and writing can have a significant impact on the overall performance of the application. Therefore, it is very important to understand how to optimize file read and write performance. First, the key to optimizing file read and write performance is to reduce the number of disk accesses. Disk I/O is a relatively slow and expensive operation, so reducing the number of disk accesses can significantly improve file reads and writes.
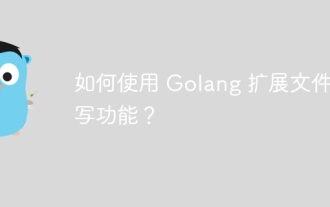
How to extend Go file reading and writing capabilities: Use the io package for general input and output operations, such as reading from a file to a memory buffer. Use the os package for operating system file system operations such as creating, deleting, and renaming files. Use these packages together to perform complex operations such as reading files and counting words.
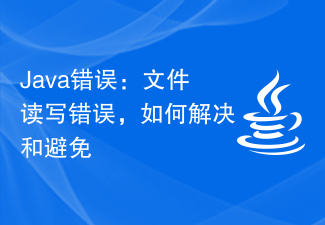
Java Error: File reading and writing errors, how to solve and avoid them. In Java programming, file reading and writing is a very common operation, but you may encounter various errors when reading and writing files. Among them, file reading and writing errors may be the most common. A common one. This article will discuss the causes, solutions, and avoidance of Java file reading and writing errors. Causes of file read and write errors In Java, the main causes of file read and write errors are as follows: The file does not exist or the path is wrong: When the specified file does not exist or the path is wrong, Java cannot open it.
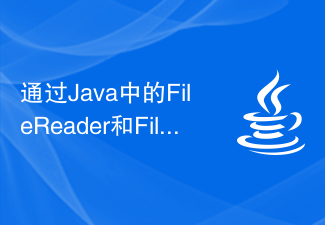
Use the FileReader and FileWriter classes to implement simple Java file reading and writing. File reading and writing is one of the very common operations in daily programming. Java provides a variety of classes and methods for file reading and writing. Among them, FileReader and FileWriter are two commonly used classes for reading and writing text files. The FileReader class is used to read text files. The file content can be read by characters or by character arrays. FileWriter class is used for writing
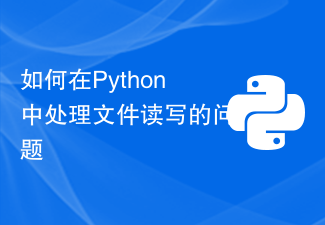
How to deal with the problem of file reading and writing in Python requires specific code examples. In Python, file reading and writing is a common operation task. Whether processing text files or binary files, Python provides powerful and flexible file reading and writing functions. This article will introduce how to handle file reading and writing in Python and give specific code examples. 1. File reading operation to open a file In Python, use the open() function to open the file. The open() function accepts two parameters: file
