


How to deal with multi-task scheduling and parallel processing problems and solutions in C# development
How to deal with multi-task scheduling and parallel processing problems and solutions in C# development
In C# development, dealing with multi-task scheduling and parallel processing is a very common requirement . How to efficiently handle multitasking and parallel tasks can improve program performance and response speed. This article will introduce how to use C#'s multi-threading and task parallel libraries to implement multi-task scheduling and parallel processing, and provide specific code examples.
1. Multi-threading
Multi-threading is a method of handling multi-tasking. In C#, you can use the Thread class to create and start threads. The following is a simple multi-threading example:
using System; using System.Threading; class Program { static void Main() { Thread t1 = new Thread(DoWork); Thread t2 = new Thread(DoWork); t1.Start(); t2.Start(); t1.Join(); t2.Join(); Console.WriteLine("All tasks completed."); } static void DoWork() { // 执行一些耗时的操作 } }
In the above code, we use the Thread class to create two threads t1 and t2 and start them respectively. Then use the Join method to wait for the two threads to complete execution. The final output of "All tasks completed." indicates that all tasks have been completed.
By using multi-threading, we can assign tasks to multiple threads for execution at the same time to improve the program's processing power and response speed.
2. Task Parallel Library
After C# 4.0, Microsoft introduced the Task Parallel Library (TPL) to simplify parallel programming. Through TPL, multi-task scheduling and parallel processing can be handled more conveniently.
The following is an example of using TPL:
using System; using System.Threading.Tasks; class Program { static void Main() { Task t1 = Task.Run(() => DoWork()); Task t2 = Task.Run(() => DoWork()); Task.WhenAll(t1, t2).ContinueWith(t => { Console.WriteLine("All tasks completed."); }); Console.ReadLine(); } static void DoWork() { // 执行一些耗时的操作 } }
In the above code, we use the Task.Run method to create two tasks t1 and t2, and wait for the two tasks through the Task.WhenAll method Finished. Then use the ContinueWith method to output "All tasks completed." after all tasks are completed.
Compared with multi-threading, TPL provides a more advanced parallel processing method, making it easier to write and maintain code. At the same time, TPL also provides more task scheduling and control methods, such as setting task priority, canceling tasks, etc.
3. Parallel processing
In addition to using multi-threading and task parallel libraries to handle multitasking, C# also provides parallel processing methods that can better utilize the performance of multi-core processors.
The following is an example of using parallel processing:
using System; using System.Threading.Tasks; class Program { static void Main() { Parallel.For(0, 10, i => { DoWork(i); }); Console.WriteLine("All tasks completed."); Console.ReadLine(); } static void DoWork(int i) { // 执行一些耗时的操作 } }
In the above code, we use the Parallel.For method to process tasks in parallel. By specifying the scope of the task and the operations to be performed, you can let the program use multiple cores to process tasks in parallel. After all tasks are completed, "All tasks completed." is output.
It should be noted that parallel processing has no order requirements between tasks, so when designing parallel processing, the independence and mutually exclusive access between tasks need to be considered.
Conclusion
By using C#’s multi-threading and task parallel libraries, we can easily handle the needs of multi-task scheduling and parallel processing. Whether it is simple multi-thread processing or advanced TPL parallel processing, it can improve the performance and response speed of the program.
When writing code for multitasking and parallel tasks, you need to pay attention to issues such as thread safety and task scheduling to ensure the correctness and stability of the program. Through reasonable design and optimization, the advantages of multi-threading and parallel processing can be fully utilized to achieve efficient multi-task scheduling and parallel processing.
The above is the detailed content of How to deal with multi-task scheduling and parallel processing problems and solutions in C# development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


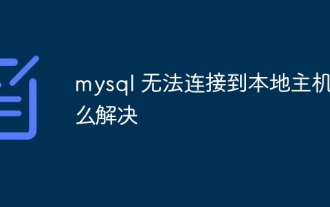
The MySQL connection may be due to the following reasons: MySQL service is not started, the firewall intercepts the connection, the port number is incorrect, the user name or password is incorrect, the listening address in my.cnf is improperly configured, etc. The troubleshooting steps include: 1. Check whether the MySQL service is running; 2. Adjust the firewall settings to allow MySQL to listen to port 3306; 3. Confirm that the port number is consistent with the actual port number; 4. Check whether the user name and password are correct; 5. Make sure the bind-address settings in my.cnf are correct.
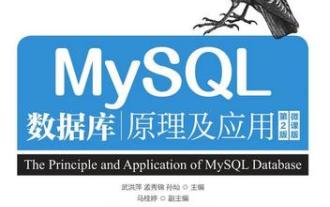
The solution to MySQL installation error is: 1. Carefully check the system environment to ensure that the MySQL dependency library requirements are met. Different operating systems and version requirements are different; 2. Carefully read the error message and take corresponding measures according to prompts (such as missing library files or insufficient permissions), such as installing dependencies or using sudo commands; 3. If necessary, try to install the source code and carefully check the compilation log, but this requires a certain amount of Linux knowledge and experience. The key to ultimately solving the problem is to carefully check the system environment and error information, and refer to the official documents.
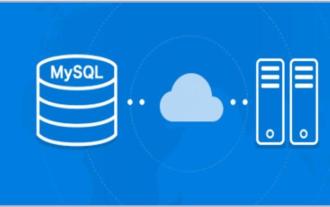
The main reasons for MySQL installation failure are: 1. Permission issues, you need to run as an administrator or use the sudo command; 2. Dependencies are missing, and you need to install relevant development packages; 3. Port conflicts, you need to close the program that occupies port 3306 or modify the configuration file; 4. The installation package is corrupt, you need to download and verify the integrity; 5. The environment variable is incorrectly configured, and the environment variables must be correctly configured according to the operating system. Solve these problems and carefully check each step to successfully install MySQL.
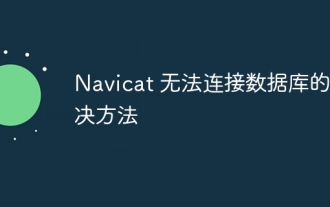
The following steps can be used to resolve the problem that Navicat cannot connect to the database: Check the server connection, make sure the server is running, address and port correctly, and the firewall allows connections. Verify the login information and confirm that the user name, password and permissions are correct. Check network connections and troubleshoot network problems such as router or firewall failures. Disable SSL connections, which may not be supported by some servers. Check the database version to make sure the Navicat version is compatible with the target database. Adjust the connection timeout, and for remote or slower connections, increase the connection timeout timeout. Other workarounds, if the above steps are not working, you can try restarting the software, using a different connection driver, or consulting the database administrator or official Navicat support.
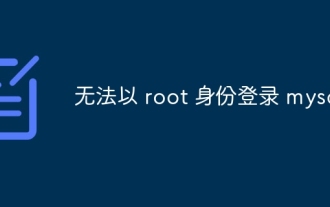
The main reasons why you cannot log in to MySQL as root are permission problems, configuration file errors, password inconsistent, socket file problems, or firewall interception. The solution includes: check whether the bind-address parameter in the configuration file is configured correctly. Check whether the root user permissions have been modified or deleted and reset. Verify that the password is accurate, including case and special characters. Check socket file permission settings and paths. Check that the firewall blocks connections to the MySQL server.
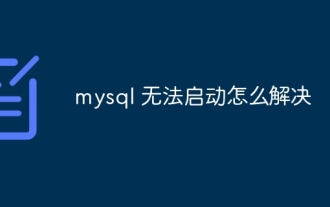
There are many reasons why MySQL startup fails, and it can be diagnosed by checking the error log. Common causes include port conflicts (check port occupancy and modify configuration), permission issues (check service running user permissions), configuration file errors (check parameter settings), data directory corruption (restore data or rebuild table space), InnoDB table space issues (check ibdata1 files), plug-in loading failure (check error log). When solving problems, you should analyze them based on the error log, find the root cause of the problem, and develop the habit of backing up data regularly to prevent and solve problems.
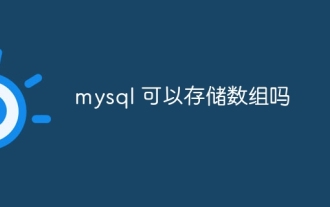
MySQL does not support array types in essence, but can save the country through the following methods: JSON array (constrained performance efficiency); multiple fields (poor scalability); and association tables (most flexible and conform to the design idea of relational databases).
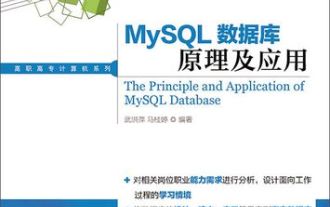
MySQL configuration file corruption can be repaired through the following solutions: 1. Simple fix: If there are only a small number of errors (such as missing semicolons), use a text editor to correct it, and be sure to back up before modifying; 2. Complete reconstruction: If the corruption is serious or the configuration file cannot be found, refer to the official document or copy the default configuration file of the same version, and then modify it according to the needs; 3. Use the installation program to provide repair function: Try to automatically repair the configuration file using the repair function provided by the installer. After selecting the appropriate solution to repair it, you need to restart the MySQL service and verify whether it is successful and develop good backup habits to prevent such problems.
