


How to solve the connection pool expansion problem of concurrent database connections in Go language?
How to solve the connection pool expansion problem of concurrent database connections in Go language?
Introduction:
In the Go language, database operations are a common concurrency scenario. When multiple goroutines need to access the database at the same time, in order to avoid frequently opening and closing database connections, we usually use a connection pool to manage the reuse of database connections. However, the size of the connection pool is limited, and when concurrent requests increase, the connection pool may become full. In order to solve this problem, we need to implement a connection pool expansion mechanism.
Basic implementation of connection pool:
First, we need to implement a basic connection pool. We can use sync.Pool to manage connection objects. The connection object can be a database connection structure, pointer, or other custom type. We store the connection object in a variable of type sync.Pool. The connection object needs to implement a Close() method to close the connection.
The following is a basic connection pool code example:
package main import ( "database/sql" "sync" ) type Conn struct { DB *sql.DB } func NewConn() *Conn { // 实现数据库连接的创建逻辑 db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/test") if err != nil { panic(err) } return &Conn{DB: db} } func (c *Conn) Close() { // 实现数据库连接的关闭逻辑 c.DB.Close() } var pool sync.Pool func main() { pool.New = func() interface{} { // 创建新的连接对象 return NewConn() } conn := pool.Get().(*Conn) defer pool.Put(conn) // 使用数据库连接执行操作 }
Implementation of the connection pool expansion mechanism:
The key to realizing the connection pool expansion is to define a dynamic expansion condition . When the connection pool has insufficient free connections, we can trigger expansion by checking the number of connections in the connection pool when acquiring connections. We can add a judgment on the number of connection pool connections in the function that obtains the connection.
The following is a code example of the connection pool expansion mechanism:
package main import ( "database/sql" "sync" ) const ( MaxConnections = 100 // 最大连接数 IdleThreshold = 10 // 空闲连接数的阈值,小于该值时触发扩容 ) type Conn struct { DB *sql.DB } func NewConn() *Conn { db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/test") if err != nil { panic(err) } return &Conn{DB: db} } func (c *Conn) Close() { c.DB.Close() } var pool sync.Pool var connCount int // 当前连接数 func init() { pool.New = func() interface{} { return NewConn() } } func GetConn() *sql.DB { // 检查空闲连接数 if connCount < IdleThreshold { expandPool() } conn := pool.Get().(*Conn) return conn.DB } func PutConn(db *sql.DB) { conn := &Conn{DB: db} pool.Put(conn) } func expandPool() { for i := 0; i < MaxConnections/10; i++ { conn := NewConn() pool.Put(conn) connCount++ } } func main() { // 使用连接池的连接进行数据库操作 db := GetConn() defer PutConn(db) }
In the above code, we use connCount to record the number of connections in the current connection pool. When the number of connections is less than IdleThreshold, trigger Expansion. The expandPool function expands according to the setting of MaxConnections, expanding the number of connections by 10% each time.
Summary:
The above is a specific code example to solve the problem of connection pool expansion of concurrent database connections in Go language. Through reasonable connection pool design and expansion mechanism, we can improve the efficiency and performance of database connections in concurrent scenarios. Of course, the specific implementation needs to be adjusted and optimized according to the actual situation to meet business needs.
The above is the detailed content of How to solve the connection pool expansion problem of concurrent database connections in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


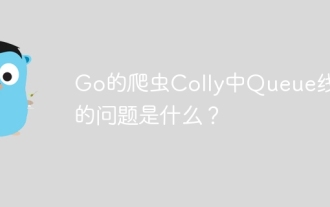
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
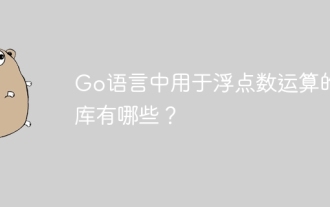
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
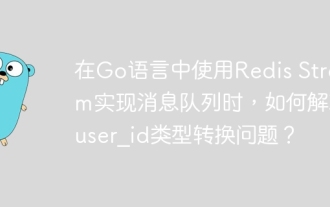
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
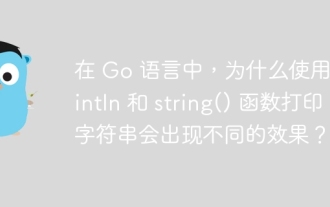
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
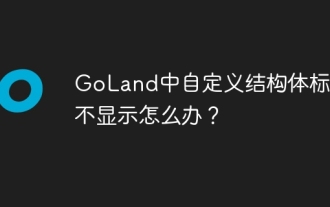
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
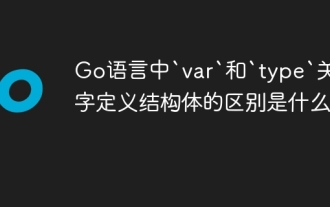
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
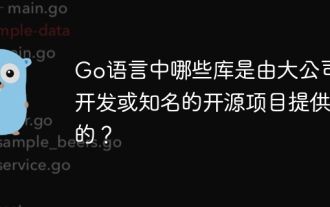
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
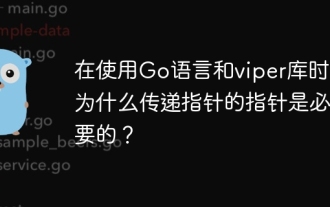
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
