PHP study notes: modular development and code reuse
PHP study notes: modular development and code reuse
Introduction:
In software development, modular development and code reuse are very important concept. Modular development can decompose complex systems into manageable small modules, improving development efficiency and code maintainability; while code reuse can reduce redundant code and improve code reusability. In PHP development, we can achieve modular development and code reuse through some technical means. This article will introduce some commonly used techniques and specific code examples to help readers better understand and apply these concepts.
1. Use namespace for modular development
Namespace is a feature introduced in PHP5.3 version, which can help us achieve modular development in the project. It can group and name related classes, interfaces, functions, etc., and avoid naming conflicts. The following is a simple example showing how to use namespaces for modular development:
// 定义命名空间 namespace MyProjectModule; // 定义一个类 class MyClass { // 类的代码 } // 使用类时,需要指定命名空间 $obj = new MyProjectModuleMyClass();
By using namespaces, we can place the code of different modules in different namespaces to achieve modular development. .
2. Use class inheritance to achieve code reuse
PHP is an object-oriented programming language. We can use class inheritance to achieve code reuse. Subclasses can inherit the properties and methods of the parent class, and can add their own properties and methods. The following is a simple example that shows how to use class inheritance to achieve code reuse:
// 定义一个基类 class BaseClass { protected $name; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } } // 定义一个子类,继承基类 class SubClass extends BaseClass { public function sayHello() { return "Hello, " . $this->name; } } // 使用子类 $obj = new SubClass("John"); echo $obj->getName(); // 输出 "John" echo $obj->sayHello(); // 输出 "Hello, John"
By using class inheritance, we can put shared code in a base class and then inherit it in subclasses base class code and add your own specific code. This reduces the need to rewrite similar code.
3. Use Trait to achieve code reuse
PHP5.4 version introduces the Trait feature, which can help us achieve code reuse. Trait is a code reuse mechanism. By using Trait in a class, some common code fragments can be injected into the class. The following is a simple example showing how to use Trait to achieve code reuse:
trait Logger { public function log($message) { echo $message; } } class MyClass { use Logger; } $obj = new MyClass(); $obj->log("This is a log message"); // 输出 "This is a log message"
By using Trait, we can encapsulate some reusable code fragments in the class in Trait and then use it in the required class Trait. This takes code reusability to a new level.
Conclusion:
Modular development and code reuse are crucial concepts in software development. In PHP development, we can use namespaces, class inheritance, traits and other technical means to achieve modular development and code reuse. This article introduces the basic concepts and specific code examples of these technologies. It is hoped that readers can better apply these technologies and improve development efficiency and code quality through learning and practice.
The above is the detailed content of PHP study notes: modular development and code reuse. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


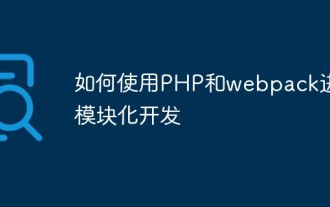
With the continuous development of web development technology, front-end and back-end separation and modular development have become a widespread trend. PHP is a commonly used back-end language. When doing modular development, we need to use some tools to manage and package modules. Webpack is a very easy-to-use modular packaging tool. This article will introduce how to use PHP and webpack for modular development. 1. What is modular development? Modular development refers to decomposing a program into different independent modules. Each module has its own function.
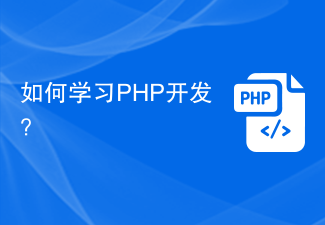
With the development of the Internet, the demand for dynamic web pages is increasing. As a mainstream programming language, PHP is widely used in web development. So, for beginners, how to learn PHP development? 1. Understand the basic knowledge of PHP. PHP is a scripting language that can be directly embedded in HTML code and parsed and run through a web server. Therefore, before learning PHP, you can first understand the basics of front-end technologies such as HTML, CSS, and JavaScript to better understand the operation of PHP.
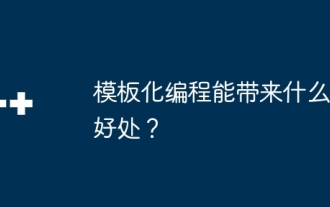
Templated programming improves code quality because it: Enhances readability: Encapsulates repetitive code, making it easier to understand. Improved maintainability: Just change the template to accommodate data type changes. Optimization efficiency: The compiler generates optimized code for specific data types. Promote code reuse: Create common algorithms and data structures that can be reused.
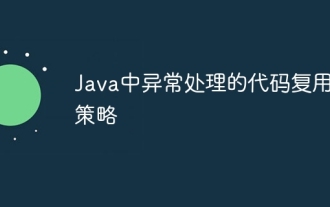
Code reuse strategy for exception handling in Java: catch and handle common exceptions (NullPointerException, IllegalArgumentException, IndexOutOfBoundsException, IOException). Use try-catch block to catch all exceptions. Use separate catch blocks for specific exceptions. Create custom exception classes to handle custom exceptions. Use code reuse to simplify exception handling, such as encapsulating error handling into the readFileWithErrorHandler method in the file reading example.
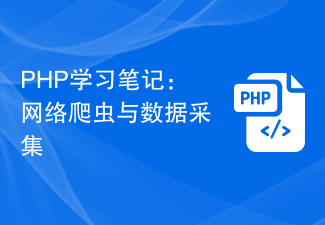
PHP study notes: Web crawler and data collection Introduction: A web crawler is a tool that automatically crawls data from the Internet. It can simulate human behavior, browse web pages and collect the required data. As a popular server-side scripting language, PHP also plays an important role in the field of web crawlers and data collection. This article will explain how to write a web crawler using PHP and provide practical code examples. 1. Basic principles of web crawlers The basic principles of web crawlers are to send HTTP requests, receive and parse the H response of the server.
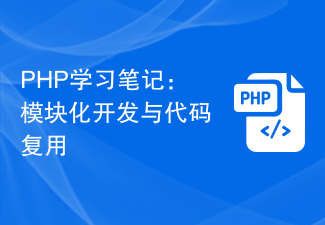
PHP study notes: Modular development and code reuse Introduction: In software development, modular development and code reuse are very important concepts. Modular development can decompose complex systems into manageable small modules, improving development efficiency and code maintainability; while code reuse can reduce redundant code and improve code reusability. In PHP development, we can achieve modular development and code reuse through some technical means. This article will introduce some commonly used technologies and specific code examples to help readers better understand and apply these concepts.
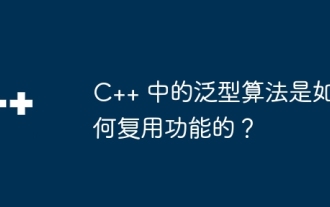
C++ generic algorithms can reuse common operations, including: sorting algorithms (such as sort) search algorithms (such as find) set operations (such as set_difference) conversion algorithms (such as transform) When using generic algorithms, you need to provide input containers and output containers ( optional) and a function object as parameters. For example, the sort algorithm can be used to sort arrays of integers. Custom comparators can be used to sort data according to specific rules. In practical cases, the std::max_element algorithm can be used to find the maximum value in a container, improving code simplicity and maintainability.
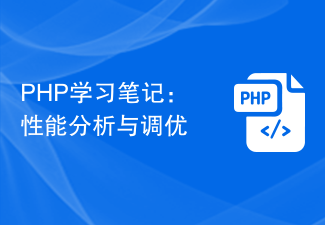
PHP study notes: Performance analysis and tuning Introduction: In web development, performance is a very critical factor. A high-performance website can provide a better user experience, improve user retention, and increase business revenue. In PHP development, performance optimization is a common and important issue. This article will introduce performance analysis and tuning methods in PHP, and provide specific code examples to help readers better understand and apply these techniques. 1. Performance analysis tool Xdebug extension Xdebug is a powerful P
