How to implement distributed transaction management in Java
How to implement distributed transaction management in Java
Introduction:
In the development process of distributed systems, due to the autonomy and autonomy between various services Data distribution leads to complexity of transaction management. In order to ensure the data consistency and reliability of distributed systems, we need to ensure the consistency of transaction operations between various subsystems through distributed transaction management. This article will introduce how to implement distributed transaction management in Java and provide specific code examples.
1. What is distributed transaction management:
Distributed transaction management refers to a set of atomic operations that operate multiple transaction resources in a distributed system. To put it simply, multiple services participate in a transaction at the same time, and either all succeed or all fail to ensure data consistency.
2. Commonly used distributed transaction management solutions in Java:
- JTA (Java Transaction API): It is defined in Java Enterprise Edition (Java EE) for distributed transactions Transaction API. JTA provides a standard way to implement transactions across multiple resource managers. By using JTA, transactions can be deployed across multiple hosts and distributed systems.
- Seata: It is an open source distributed transaction solution and a distributed transaction open source project of Alibaba. Seata has integrated distributed transaction management functions and solves the consistency problem between application systems and databases by supporting multiple traditional and Internet data access modes.
3. Use JTA to implement distributed transaction management:
JTA is a set of standard APIs for distributed transaction management, which can be used for applications in JavaEE or for independent applications. Java application. The following is a specific example code for using JTA to implement distributed transaction management in Java.
//Import required dependencies
import javax.transaction.*;
import javax.transaction.xa.*;
public class DistributedTransaction {
public static void main(String[] args) throws SystemException, NotSupportedException, HeuristicRollbackException, HeuristicMixedException, RollbackException{ // 初始化全局事务管理器 UserTransactionManager tm = new UserTransactionManager(); tm.setTransactionTimeout(300); // 设置事务超时时间为300秒 UserTransaction ut = new UserTransactionImp(); // 开启全局事务 ut.begin(); try { // 执行业务操作1 doBusiness1(); // 执行业务操作2 doBusiness2(); // 提交事务 ut.commit(); } catch (Exception e) { // 回滚事务 ut.rollback(); } } // 业务操作1 public static void doBusiness1() { // 实现具体的业务逻辑 } // 业务操作2 public static void doBusiness2() { // 实现具体的业务逻辑 }
}
The above sample code demonstrates how to implement distributed transaction management through JTA. When using JTA, we need to manually open and submit transactions, and perform specific business operations in the transaction. If an exception occurs in any step of the business operation, we need to manually roll back the transaction.
4. Summary:
Distributed transaction management is crucial to ensuring the data consistency and reliability of distributed systems. There are many solutions to implement distributed transaction management in Java, such as JTA and Seata. This article describes how to use JTA to implement distributed transaction management in Java and provides specific code examples. Readers can choose a distributed transaction management solution that suits them based on actual needs and practice based on the sample code.
The above is the detailed content of How to implement distributed transaction management in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


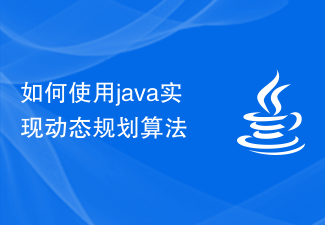
How to use Java to implement dynamic programming algorithm Dynamic programming is an optimization method for solving multi-stage decision-making problems. It decomposes the problem into multiple stages. Each stage makes a decision based on known information and records the results of each decision so that used in subsequent stages. In practical applications, dynamic programming is usually used to solve optimization problems, such as shortest path, maximum subsequence sum, knapsack problem, etc. This article will introduce how to use Java language to implement dynamic programming algorithms and provide specific code examples. 1. Basic principles of dynamic programming algorithms
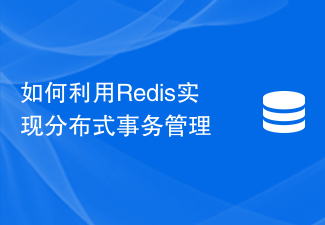
How to use Redis to implement distributed transaction management Introduction: With the rapid development of the Internet, the use of distributed systems is becoming more and more widespread. In distributed systems, transaction management is an important challenge. Traditional transaction management methods are difficult to implement in distributed systems and are inefficient. Using the characteristics of Redis, we can easily implement distributed transaction management and improve the performance and reliability of the system. 1. Introduction to Redis Redis is a memory-based data storage system with efficient read and write performance and rich data
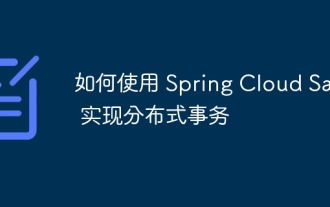
SpringCloudSaga provides a declarative way to coordinate distributed transactions, simplifying the implementation process: add Maven dependency: spring-cloud-starter-saga. Create a Saga orchestrator (@SagaOrchestration). Write participants to implement SagaExecution to execute business logic and compensation logic (@SagaStep). Define state transitions and actors in Saga. By using SpringCloudSaga, atomicity between different microservice operations is ensured.
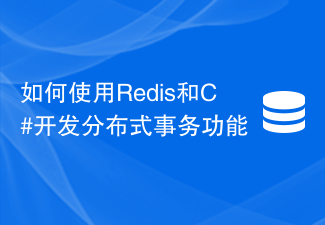
How to use Redis and C# to develop distributed transaction functions Introduction Transaction processing is a very important function in the development of distributed systems. Transaction processing can guarantee that a series of operations in a distributed system will either succeed or be rolled back. Redis is a high-performance key-value store database, while C# is a programming language widely used for developing distributed systems. This article will introduce how to use Redis and C# to implement distributed transaction functions, and provide specific code examples. I.Redis transactionRedis
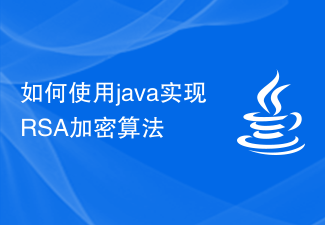
How to use Java to implement the RSA encryption algorithm RSA (Rivest-Shamir-Adleman) is an asymmetric encryption algorithm, which is one of the most commonly used encryption algorithms currently. This article will introduce how to use Java language to implement the RSA encryption algorithm and provide specific code examples. Generate a key pair First, we need to generate a pair of RSA keys, which consists of a public key and a private key. The public key can be used to encrypt data and the private key can be used to decrypt data. The following is a code example to generate an RSA key pair: import
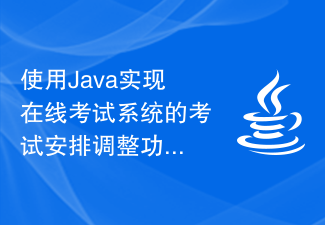
Java implementation of the examination arrangement adjustment function of the online examination system Introduction: With the development of Internet technology, more and more schools and training institutions choose to use online examination systems for examinations and assessments. Examination schedule adjustment is an important function in the online examination system, which can help administrators flexibly adjust examination time and examination-related information according to the actual situation. This article will introduce in detail how to use Java programming to implement the examination schedule adjustment function of the online examination system, and give specific code examples. Database design exam arrangement adjustment function needs
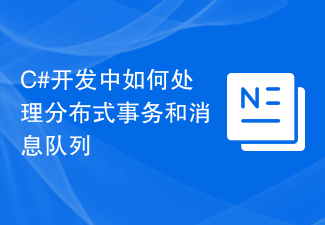
How to handle distributed transactions and message queues in C# development Introduction: In today's distributed systems, transactions and message queues are very important components. Distributed transactions and message queues play a crucial role in handling data consistency and system decoupling. This article will introduce how to handle distributed transactions and message queues in C# development, and give specific code examples. 1. Distributed transactions Distributed transactions refer to transactions that span multiple databases or services. In distributed systems, how to ensure data consistency has become a major challenge. Here are two types of
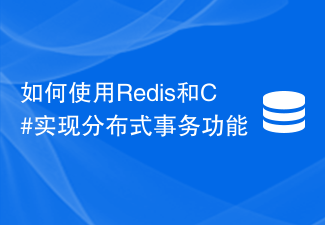
How to use Redis and C# to implement distributed transaction functions Introduction: With the rapid development of the Internet and the continuous expansion of user scale, distributed system architecture has become a common solution. One of the key issues in distributed systems is ensuring data consistency, especially in cross-database transactions involving multiple databases. Redis is an efficient in-memory database that provides features for implementing distributed transactions and can be used in conjunction with the C# language to build distributed systems. This article will introduce how to use Redis and C#
