How to achieve consistency and fault tolerance of distributed cache in Java
How to achieve consistency and fault tolerance of distributed cache in Java
Introduction:
In modern distributed systems, cache is the key to improving performance One of the methods is widely used in various scenarios. However, when the cache needs to be distributed across multiple nodes, ensuring data consistency and fault tolerance becomes particularly important. This article will introduce how to achieve the consistency and fault tolerance of distributed cache in Java, and give specific code examples.
1. Consistency
- Data consistency issue
In a distributed cache system, the cached data of different nodes needs to be consistent. However, data inconsistency may occur due to network delays, node failures, etc. - Consistent Hash Algorithm
Consistent hash algorithm is a common method to solve the consistency problem of distributed cache. The principle is to distribute the cache nodes on a ring based on the hash value. When data needs to be queried or written, the corresponding node is selected based on the hash value of the data. This ensures that when the node changes, only a small amount of cached data needs to be remapped to the new node, improving the stability and performance of the system. - Java code example
The following is a Java code example of a simple consistent hashing algorithm implementation:
public class ConsistentHashing { private TreeMap<Integer, String> nodes = new TreeMap<>(); // 添加节点 public void addNode(String node) { int hash = getHash(node); nodes.put(hash, node); } // 移除节点 public void removeNode(String node) { int hash = getHash(node); nodes.remove(hash); } // 获取节点 public String getNode(String key) { int hash = getHash(key); // 顺时针找到第一个大于等于该哈希值的节点 Integer nodeKey = nodes.ceilingKey(hash); if (nodeKey == null) { // 没有找到,则返回第一个节点 nodeKey = nodes.firstKey(); } return nodes.get(nodeKey); } // 计算哈希值 private int getHash(String key) { // 模拟哈希函数 return key.hashCode() % 360; } }
2. Fault tolerance
- Fault Tolerance Issue
In a distributed cache system, nodes may fail due to network failures, downtime and other reasons. In order to ensure the availability of the system, these faults need to be fault-tolerant. - Fault tolerance of consistent hashing algorithm
The consistent hashing algorithm has natural fault tolerance when a node fails. When a node fails, cached data is automatically mapped to other nodes and will not be lost. At the same time, the problem of data skew can be solved by introducing virtual nodes and the load balancing capability of the system can be improved. - Java code example
The following is a Java code example of a simple distributed cache system, using consistent hashing algorithm and multi-threading technology to achieve fault tolerance:
public class DistributedCache { private Map<String, String> cache = new ConcurrentHashMap<>(); private ConsistentHashing consistentHashing = new ConsistentHashing(); private List<String> nodes = new ArrayList<>(); // 初始化节点 public void initNodes(List<String> nodes) { for (String node : nodes) { consistentHashing.addNode(node); } this.nodes = nodes; } // 获取缓存数据 public String get(String key) { String node = consistentHashing.getNode(key); return cache.getOrDefault(key, getNodeFromOtherNode(node, key)); } // 从其他节点获取数据 private String getNodeFromOtherNode(String node, String key) { for (String otherNode : nodes) { if (!otherNode.equals(node)) { // 从其他节点获取数据 // ... } } return null; } // 写入缓存数据 public void put(String key, String value) { String node = consistentHashing.getNode(key); cache.put(key, value); updateNode(node, key); } // 更新节点数据 private void updateNode(String node, String key) { for (String otherNode : nodes) { if (!otherNode.equals(node)) { // 发送更新请求到其他节点 // ... } } } }
Conclusion:
The consistent hash algorithm can ensure the data consistency of the distributed cache system and have a certain fault tolerance. Through the above Java code examples, we can see how to achieve the consistency and fault tolerance of distributed cache in Java. Of course, more details and optimizations need to be considered in actual applications, but the above code example can be used as a basic framework for your reference and expansion.
The above is the detailed content of How to achieve consistency and fault tolerance of distributed cache in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
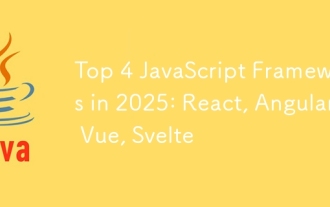
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
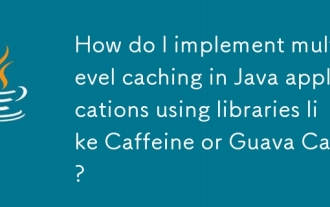
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
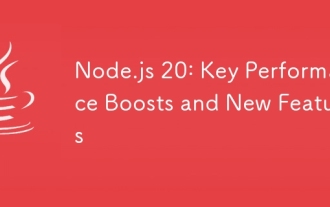
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
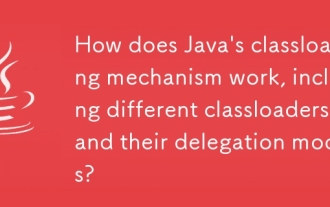
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
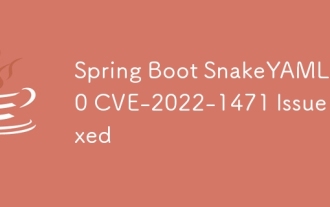
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
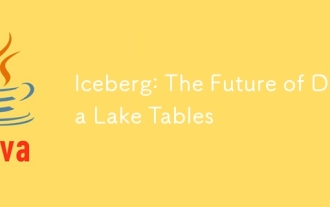
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
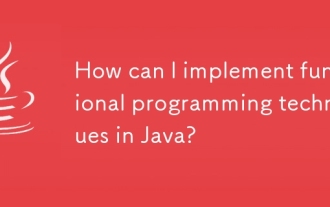
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
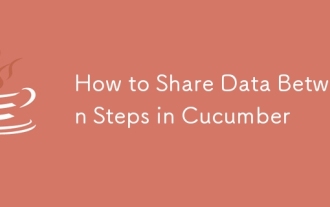
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
