


How to solve data security and encrypted storage in PHP development
How to solve data security and encrypted storage in PHP development
With the popularity of the Internet and the development of applications, data security and encrypted storage are becoming more and more important important. In PHP development, we need to take some measures to ensure the security of sensitive data to prevent potential attacks and data leaks. This article will introduce some common methods and examples to help you solve the problems of data security and encrypted storage in PHP development.
- Using HTTPS protocol
HTTPS is a way to communicate securely on the Internet by using the SSL/TLS protocol. By using an HTTPS connection, you can ensure the security of your data during transmission. In PHP development, you can use the HTTPS protocol by simply setting the URL's protocol to HTTPS.
Sample code:
$url = "https://example.com";
- Using the password hashing algorithm
The password hashing algorithm is a method of converting a user's password into an irreversible string . In PHP, commonly used password hashing algorithms include MD5, SHA1, BCrypt, etc. By using a password hashing algorithm, you can ensure the security of user passwords during storage.
Sample code:
$password = "myPassword"; $hashedPassword = password_hash($password, PASSWORD_DEFAULT);
- Use prepared statements
Prepared statements are a method of compiling SQL statements and binding parameters in advance. Effectively prevent SQL injection attacks. In PHP, we can use PDO or MySQLi to execute prepared statements.
Sample code (using PDO):
$pdo = new PDO("mysql:host=localhost;dbname=myDatabase", "username", "password"); $stmt = $pdo->prepare("SELECT * FROM users WHERE id = :id"); $stmt->bindParam(":id", $id); $stmt->execute();
- Using encryption algorithms
In addition to password hashing algorithms, you can also use encryption algorithms to encrypt sensitive data. In PHP, commonly used encryption algorithms include AES and RSA. By encrypting data, even if the data is leaked, sensitive information will not be directly exposed.
Sample code (using AES encryption):
$data = "secretData"; $key = "mySecretKey"; $encryptedData = openssl_encrypt($data, "AES-128-ECB", $key);
- Using the verification code
The verification code is a test used to confirm the identity of the user. In PHP development, by using verification codes, you can prevent bots or malicious attackers from automatically submitting forms.
Sample code:
// 生成验证码 function generateCaptcha() { $captcha = rand(1000, 9999); $_SESSION["captcha"] = $captcha; return $captcha; } // 验证验证码 function validateCaptcha($input) { if (!isset($_SESSION["captcha"]) || empty($_SESSION["captcha"])) { return false; } return $input == $_SESSION["captcha"]; }
In summary, ensuring data security and encrypted storage are important issues that cannot be ignored in PHP development. By following the above measures and sample code, you can effectively protect the security of sensitive data during the development process. At the same time, we also recommend that you regularly update and upgrade your development frameworks and libraries to keep up with the latest security work.
The above is the detailed content of How to solve data security and encrypted storage in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


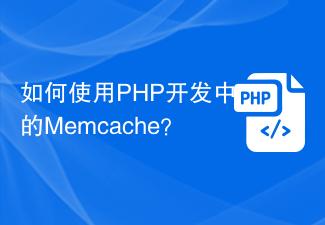
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
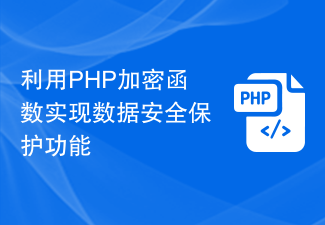
In the Internet age, data security protection has become an important issue that enterprises and individuals must face. For the protection of sensitive data, encrypting data using appropriate encryption algorithms is a common solution. As a programming language widely used in web development, PHP has a rich encryption function library that can well implement data security protection functions. PHP provides a variety of encryption functions, including symmetric encryption algorithms and asymmetric encryption algorithms. The symmetric encryption algorithm uses the same key for encryption and decryption. The encryption and decryption process is highly efficient and is suitable for large-scale encryption.
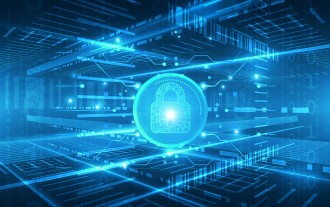
Introduction In the era of information explosion, data has become one of the most valuable assets of enterprises. However, if a large amount of data cannot be effectively classified and classified, it will become disordered and chaotic, data security cannot be effectively guaranteed, and its true data value cannot be exerted. Therefore, data classification and grading have become crucial for both data security and data value. This article will discuss the importance of data classification and classification, and introduce how to use machine learning to achieve intelligent classification and classification of data. 1. The Importance of Data Classification and Grading Data classification and grading is the process of classifying and sorting data according to certain rules and standards. It can help enterprises better manage data and improve data confidentiality, availability, integrity and accessibility, thereby better supporting business decisions.
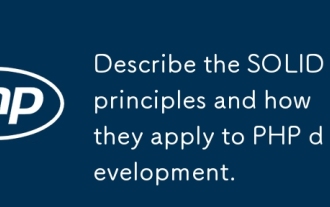
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
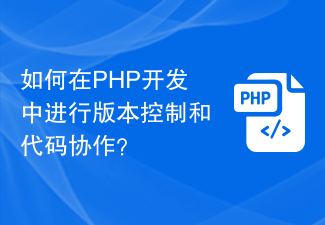
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
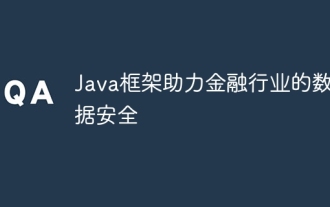
The Java framework helps ensure data security in the financial industry by providing authentication, data validation, encryption, and web application security tools. For example, Spring Security can be used to implement user authentication, authorization, and session management to ensure that only authorized users can access sensitive data.
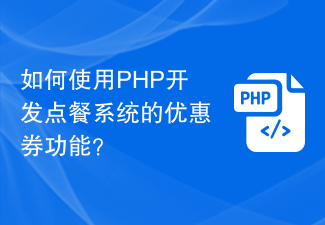
How to use PHP to develop the coupon function of the ordering system? With the rapid development of modern society, people's life pace is getting faster and faster, and more and more people choose to eat out. The emergence of the ordering system has greatly improved the efficiency and convenience of customers' ordering. As a marketing tool to attract customers, the coupon function is also widely used in various ordering systems. So how to use PHP to develop the coupon function of the ordering system? 1. Database design First, we need to design a database to store coupon-related data. It is recommended to create two tables: one
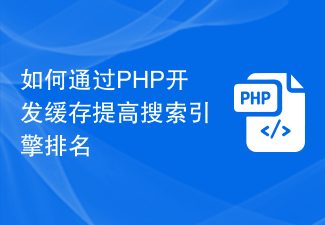
How to improve search engine rankings through PHP cache development Introduction: In today's digital era, the search engine ranking of a website is crucial to the website's traffic and exposure. In order to improve the ranking of the website, an important strategy is to reduce the loading time of the website through caching. In this article, we'll explore how to improve search engine rankings by developing caching with PHP and provide concrete code examples. 1. The concept of caching Caching is a technology that stores data in temporary storage so that it can be quickly retrieved and reused. for net
