


How to handle object serialization and deserialization in C# development
Oct 10, 2023 pm 06:07 PMHow to handle object serialization and deserialization in C# development requires specific code examples
In C# development, object serialization and deserialization are very important the concept of. Serialization converts an object into a format that can be transmitted over the network or stored on disk, while deserialization converts the serialized data back into the original object. This article will introduce how to handle the serialization and deserialization of objects in C#, and provide some specific code examples.
- Use Json.NET library for serialization and deserialization
Json.NET is a popular third-party library that allows for convenient object serialization in C# ization and deserialization. First, we need to install the Json.NET library. Json.NET can be installed in Visual Studio via the NuGet package manager.
a) Object serialization example
The following code example demonstrates how to serialize a Person object into a JSON string:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
In this example, we use The JsonConvert.SerializeObject method converts the Person object into a JSON string and outputs the result through Console.WriteLine.
b) Object deserialization example
The following code example demonstrates how to deserialize a JSON string into a Person object:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
In this example, We use the JsonConvert.DeserializeObject method to convert the JSON string to a Person object and output the values of the Name and Age properties through Console.WriteLine.
- Using BinaryFormatter for serialization and deserialization
In addition to the Json.NET library, C# also provides the BinaryFormatter class, which can be used to serialize objects into Binary format and deserialize binary data into objects. The following is an example of serialization and deserialization using BinaryFormatter:
a) Object serialization example
The following code example demonstrates how to serialize a Person object into binary data:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
|
In this example, we create a Person object and serialize it into a memory stream using the BinaryFormatter.Serialize method. Then, get the binary data through the MemoryStream.ToArray method and output it as a string using the BitConverter.ToString method.
b) Object deserialization example
The following code example demonstrates how to deserialize binary data into a Person object:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
|
In this example, we use The BinaryFormatter.Deserialize method deserializes binary data into Person objects and outputs the values of the Name and Age properties through Console.WriteLine.
Summary
Through this article, we learned how to handle object serialization and deserialization in C# development. We used the Json.NET library and the BinaryFormatter class to perform serialization and deserialization examples respectively, and provided specific code snippets. Please choose the appropriate method to handle object serialization and deserialization based on actual needs.
The above is the detailed content of How to handle object serialization and deserialization in C# development. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
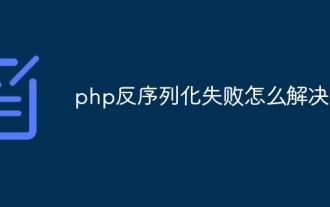
How to solve php deserialization failure
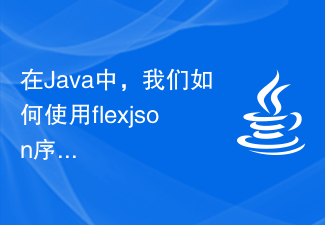
In Java, how can we serialize a list of objects using flexjson?
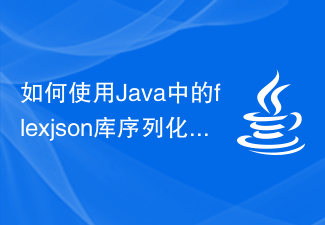
How to serialize a map using the flexjson library in Java?

How to serialize the order of properties using Jackson library in Java?
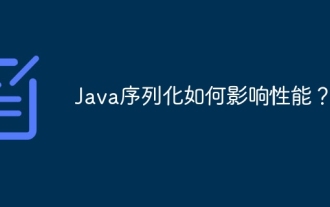
How does Java serialization affect performance?
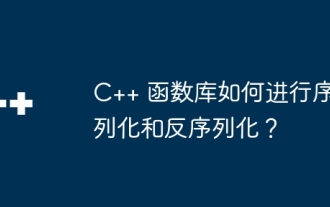
How does the C++ function library perform serialization and deserialization?
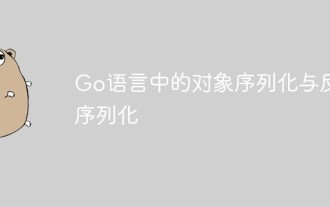
Object serialization and deserialization in Go language
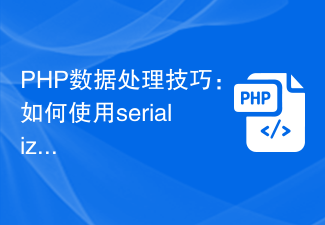
PHP data processing skills: How to use the serialize and unserialize functions to implement data serialization and deserialization
