Discussion of data structure problems and solutions in C++
Discussion on data structure problems and solutions in C
Introduction:
In C programming, data structure is an important concept that can help us Store and manage data in an organized manner. However, when faced with complex problems, we may encounter some difficulties, and how to reasonably select and use data structures becomes a key issue. This article will introduce some common data structure problems and give corresponding solutions, as well as specific code examples.
Question 1: How to implement a dynamic array?
Solution: Vector containers can be used in C to implement dynamic arrays. Vectors can automatically resize and dynamically allocate memory as needed. The following is an example of using vector:
#include <iostream> #include <vector> using namespace std; int main() { vector<int> myArray; // 创建一个int类型的vector对象 myArray.push_back(1); // 添加元素1到数组末尾 myArray.push_back(2); // 添加元素2到数组末尾 myArray.push_back(3); // 添加元素3到数组末尾 cout << "数组元素个数:" << myArray.size() << endl; // 输出数组元素个数 cout << "数组第一个元素:" << myArray[0] << endl; // 输出数组第一个元素 cout << "数组最后一个元素:" << myArray.back() << endl; // 输出数组最后一个元素 return 0; }
Question 2: How to implement a linked list?
Solution: Pointers and structures can be used in C to implement linked lists. The following is an example of using a linked list to implement a singly linked list:
#include <iostream> using namespace std; struct ListNode { int val; ListNode *next; // 构造函数 ListNode(int x) : val(x), next(NULL) {} }; int main() { ListNode *head = new ListNode(1); // 创建链表头节点 ListNode *node1 = new ListNode(2); // 创建第一个节点 head->next = node1; // 头节点的next指针指向第一个节点 cout << "链表元素:" << head->val << ", " << head->next->val << endl; delete head; // 释放链表节点的内存 delete node1; return 0; }
Question 3: How to implement a stack?
Solution: You can use vector or deque containers in C to implement the stack. The following is an example of using vector to implement a stack:
#include <iostream> #include <vector> using namespace std; class Stack { private: vector<int> data; public: // 入栈操作 void push(int val) { data.push_back(val); } // 出栈操作 void pop() { if (!isEmpty()) { data.pop_back(); } } // 获取栈顶元素 int top() { return data.back(); } // 判断栈是否为空 bool isEmpty() { return data.empty(); } }; int main() { Stack myStack; myStack.push(1); // 入栈操作 myStack.push(2); myStack.push(3); cout << "栈顶元素:" << myStack.top() << endl; // 获取栈顶元素 myStack.pop(); // 出栈操作 cout << "栈顶元素:" << myStack.top() << endl; return 0; }
Question 4: How to implement a queue?
Solution: The deque container can be used in C to implement the queue. The following is an example of using deque to implement a queue:
#include <iostream> #include <deque> using namespace std; class Queue { private: deque<int> data; public: // 入队操作 void enqueue(int val) { data.push_back(val); } // 出队操作 void dequeue() { if (!isEmpty()) { data.pop_front(); } } // 获取队首元素 int front() { return data.front(); } // 判断队列是否为空 bool isEmpty() { return data.empty(); } }; int main() { Queue myQueue; myQueue.enqueue(1); // 入队操作 myQueue.enqueue(2); myQueue.enqueue(3); cout << "队首元素:" << myQueue.front() << endl; // 获取队首元素 myQueue.dequeue(); // 出队操作 cout << "队首元素:" << myQueue.front() << endl; return 0; }
Conclusion:
In C programming, reasonable selection and use of data structures is the key to solving complex problems. This article introduces some common data structure problems and provides corresponding solutions, as well as specific code examples. I hope it can help readers better understand and apply data structures.
The above is the detailed content of Discussion of data structure problems and solutions in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


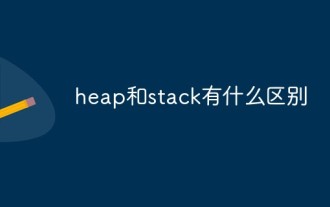
Differences: 1. The heap space is generally allocated and released by the programmer; while the stack space is automatically allocated and released by the operating system. 2. The heap is stored in the second-level cache, and the life cycle is determined by the garbage collection algorithm of the virtual machine; while the stack uses the first-level cache, which is usually in the storage space when it is called, and is released immediately after the call is completed. 3. The data structures are different. Heap can be regarded as a tree, while stack is a first-in, last-out data structure.
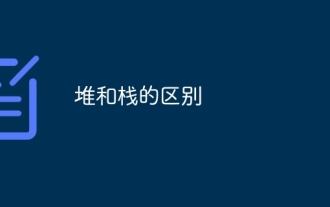
The difference between heap and stack: 1. The memory allocation method is different. The heap is manually allocated and released by the programmer, while the stack is automatically allocated and released by the operating system. 2. The size is different. The size of the stack is fixed, while the stack is automatically allocated and released by the operating system. The size of is growing dynamically; 3. Data access methods are different. In the heap, data access is achieved through pointers, while in the stack, data access is achieved through variable names; 4. Data life cycle , In the heap, the life cycle of data can be very long, while in the stack, the life cycle of variables is determined by the scope in which they are located.
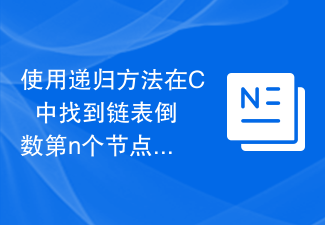
Given a singly linked list and a positive integer N as input. The goal is to find the Nth node from the end of the given list using recursion. If the input list has nodes a→b→c→d→e→f and N is 4, then the 4th node from the last will be c. We will first traverse until the last node in the list and when returning from the recursive (backtracking) increment count. When count equals N, a pointer to the current node is returned as the result. Let's look at various input and output scenarios for this - Input - List: -1→5→7→12→2→96→33N=3 Output − The Nth node from the last is: 2 Explanation − The third node is 2 . Input − List: -12→53→8→19→20→96→33N=8 Output – Node does not exist

The difference between Java heap and stack: 1. Memory allocation and management; 2. Storage content; 3. Thread execution and life cycle; 4. Performance impact. Detailed introduction: 1. Memory allocation and management. The Java heap is a dynamically allocated memory area, mainly used to store object instances. In Java, objects are allocated through heap memory. When an object is created, the Java virtual machine Allocate corresponding memory space on the system, and automatically perform garbage collection and memory management. The size of the heap can be dynamically adjusted at runtime, configured through JVM parameters, etc.
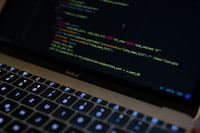
Overview of the PHPSPL Data Structure Library The PHPSPL (Standard PHP Library) data structure library contains a set of classes and interfaces for storing and manipulating various data structures. These data structures include arrays, linked lists, stacks, queues, and sets, each of which provides a specific set of methods and properties for manipulating data. Arrays In PHP, an array is an ordered collection that stores a sequence of elements. The SPL array class provides enhanced functions for native PHP arrays, including sorting, filtering, and mapping. Here is an example of using the SPL array class: useSplArrayObject;$array=newArrayObject(["foo","bar","baz"]);$array
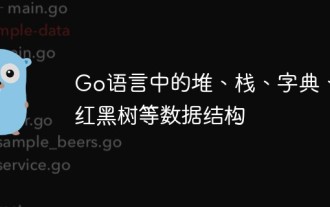
With the development of computer science, data structure has become an important subject. In software development, data structures are very important. They can improve program efficiency and readability, and can also help solve various problems. In the Go language, data structures such as heap, stack, dictionary, and red-black tree are also very important. This article will introduce these data structures and their implementation in Go language. Heap is a classic data structure used to solve priority queue problems. A priority queue refers to a queue that when taking out elements is
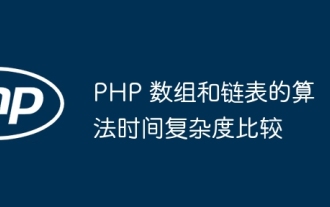
Comparison of the algorithm time complexity of arrays and linked lists: accessing arrays O(1), linked lists O(n); inserting arrays O(1), linked lists O(1)/O(n); deleting arrays O(1), linked lists O(n) (n); Search array O(n), linked list O(n).
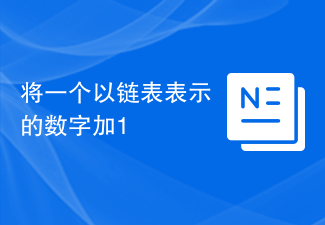
A linked list representation of a number is provided like this: All nodes of the linked list are considered to be one digit of the number. Nodes store numbers such that the first element of the linked list holds the most significant digit of the number, and the last element of the linked list holds the least significant digit of the number. For example, the number 202345 is represented in the linked list as (2->0->2->3->4->5). To add 1 to this linked list representing numbers, we must check the value of the least significant bit in the list. If it's less than 9 it's ok, otherwise the code will change the next number and so on. Now let us see an example to understand how to do this, 1999 is represented as (1->9->9->9) and adding 1 should change it
