


How to deal with file system file caching and hot loading of concurrent files in Go language?
How to deal with file system file caching and hot loading of concurrent files in Go language?
Introduction:
In the Go language, dealing with concurrent access and caching of file system files is a common and important problem. When there are multiple Goroutines in the system operating on the same file at the same time, data inconsistency or race conditions may easily occur. In addition, in order to improve program performance, caching files is a common optimization strategy. This article will introduce how to use the Go language's file system library and built-in concurrency mechanism to deal with these problems, and give specific code examples.
1. File read and write concurrency security
When multiple Goroutines read and write the same file at the same time, it is easy to cause competition conditions and data inconsistency. To avoid this situation, you can use the "sync" package provided in the Go language to implement a mutex lock.
The sample code is as follows:
import ( "os" "sync" ) var mutex sync.Mutex func writeFile(filename string, data []byte) error { mutex.Lock() defer mutex.Unlock() file, err := os.OpenFile(filename, os.O_CREATE|os.O_WRONLY, 0644) if err != nil { return err } defer file.Close() _, err = file.Write(data) return err } func readFile(filename string) ([]byte, error) { mutex.Lock() defer mutex.Unlock() file, err := os.Open(filename) if err != nil { return nil, err } defer file.Close() data, err := ioutil.ReadAll(file) return data, err }
In the above code, we use sync.Mutex
to ensure that only one Goroutine can access the file at the same time, avoiding data competition. question. When writing a file, we first lock the mutex, then open the file for writing, and finally release the lock. When reading a file, the mutex is also first locked, then the read operation is performed, and finally the lock is released. This ensures that only one Goroutine performs file reading and writing operations at the same time, avoiding the problem of data inconsistency.
2. File caching
In order to improve program performance, we can use file caching to reduce the number of accesses to the file system. In Go language, you can use sync.Map
to implement a simple file cache.
The sample code is as follows:
import ( "os" "sync" ) var cache sync.Map func readFileFromCache(filename string) ([]byte, error) { if value, ok := cache.Load(filename); ok { return value.([]byte), nil } data, err := ioutil.ReadFile(filename) if err != nil { return nil, err } cache.Store(filename, data) return data, nil } func clearCache(filename string) { cache.Delete(filename) }
In the above code, we use sync.Map
as the file cache. When a file needs to be read, first check whether it exists in the cache. The file's data. If it exists, the cached data is returned directly; if it does not exist, the file content is read and stored in the cache. When a file changes, the cached data for the file needs to be cleared.
3. Hot loading
In some scenarios, when the file changes, we hope that the program can automatically reload the latest file content. In order to implement hot reloading, we can use the os/signal
package in the Go language to monitor file changes.
The sample code is as follows:
import ( "os" "os/signal" "syscall" ) func watchFile(filename string) { signalChan := make(chan os.Signal) go func() { signal.Notify(signalChan, syscall.SIGINT, syscall.SIGTERM) <-signalChan clearCache(filename) os.Exit(0) }() watcher, err := fsnotify.NewWatcher() if err != nil { panic(err) } defer watcher.Close() err = watcher.Add(filename) if err != nil { panic(err) } for { select { case event := <-watcher.Events: if event.Op&fsnotify.Write == fsnotify.Write { clearCache(filename) } case err := <-watcher.Errors: log.Println("error:", err) } } }
In the above code, we use the fsnotify
package to monitor file changes. When the program receives an interrupt signal, that is, when the signal.Notify
is used to listen to the SIGINT
and SIGTERM
signals, we clear the cached data of the file and exit the program. When monitoring file changes, we add the files that need to be monitored through watcher.Add(filename)
, and then read the event through watcher.Events
. If it is a file writing event, then clear cache.
Conclusion:
By using the file system library and concurrency mechanism provided by the Go language, we can safely handle concurrent file read and write operations, while optimizing program performance through file caching. By monitoring file changes, we implement hot loading of files. The above sample code can help us better understand and apply these technologies. In actual development, we can adjust and optimize according to specific needs.
The above is the detailed content of How to deal with file system file caching and hot loading of concurrent files in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
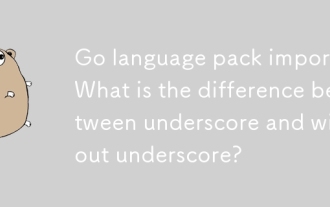
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
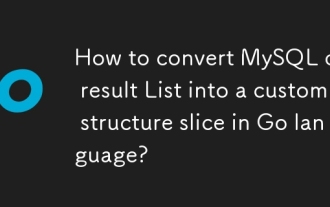
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
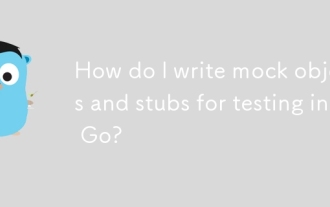
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
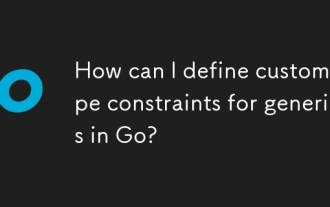
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
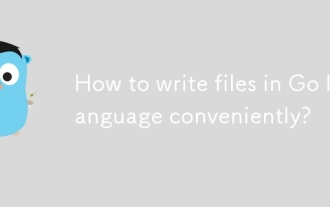
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
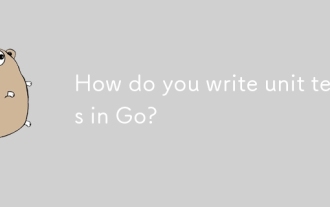
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
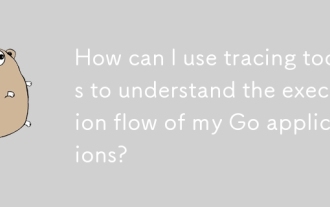
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
