


In-depth understanding of the extensibility and customization of PHP trait DTO
In-depth understanding of the scalability and customization of PHP trait DTO requires specific code examples
In object-oriented programming, the Data Transfer Object (DTO) pattern is widely used For managing and transmitting data. In PHP, using traits can realize the scalability and customization of DTO, which provides convenience for code writing and maintenance. This article will delve into the concepts related to PHP trait DTO and provide specific code examples to help readers better understand and apply this pattern.
First of all, we need to clarify the definition and role of DTO. DTO is a design pattern used to encapsulate object data and can be used to transfer data between different levels of applications. It is mainly used to decouple data transmission logic and business logic to improve the maintainability and reusability of code. In PHP, DTOs usually consist of pure data structures and do not contain any business logic.
In PHP, traits can be used to achieve the scalability and customization of DTO. A trait is a code reuse mechanism that allows you to group together a set of properties and methods and then use them in multiple classes. By using traits, the properties and methods of a DTO can be encapsulated in a reusable code fragment and used in the required classes.
The following is a simple code example to demonstrate how to use traits to implement DTO:
trait UserDTO { private $name; private $age; public function getName() { return $this->name; } public function setName($name) { $this->name = $name; } public function getAge() { return $this->age; } public function setAge($age) { $this->age = $age; } } class User { use UserDTO; private $email; public function getEmail() { return $this->email; } public function setEmail($email) { $this->email = $email; } } $user = new User(); $user->setName('John'); $user->setAge(30); $user->setEmail('john@example.com'); echo 'Name: ' . $user->getName() . '<br>'; echo 'Age: ' . $user->getAge() . '<br>'; echo 'Email: ' . $user->getEmail() . '<br>';
In the above code, we define a trait UserDTO to encapsulate the user's name and age attributes and related access methods. Then, we use the UserDTO trait in the User class and add an additional email attribute and related access methods.
By using traits, we can reuse the properties and methods defined in the UserDTO trait in the User class, and at the same time extend and customize it according to specific business needs. This approach not only improves the maintainability and reusability of the code, but also provides better flexibility.
In addition to basic properties and methods, we can also implement more complex custom logic through traits. For example, we can define a serialize method in the trait to serialize a DTO object into a JSON string:
trait SerializableDTO { public function serialize() { return json_encode(get_object_vars($this)); } } class User { use SerializableDTO; // ... } $user = new User(); $user->setName('John'); $user->setAge(30); $user->setEmail('john@example.com'); echo $user->serialize();
In the above code, we define a SerializableDTO trait, which contains a serialize method , which can serialize DTO objects into JSON strings. Then, we used the trait in the User class and called the serialize method to print out the serialized JSON string.
Through traits, we can easily apply and customize DTO patterns in different classes, thereby improving the scalability and maintainability of the code. Whether it's simple properties and methods, or more complex custom logic, traits can help us better organize and manage code.
To sum up, this article deeply explores the scalability and customization of PHP trait DTO, and provides specific code examples to help readers better understand and apply this pattern. By using traits, we can easily reuse and customize DTOs, thereby improving the maintainability and reusability of the code. I hope this article can help readers in actual development.
The above is the detailed content of In-depth understanding of the extensibility and customization of PHP trait DTO. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


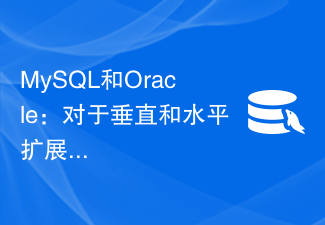
MySQL and Oracle: Vertical and horizontal expansion flexibility comparison In today's big data era, database scalability has become a crucial consideration. Scalability can be divided into two aspects: vertical expansion and horizontal expansion. In this article, we will focus on comparing the flexibility of two common relational databases, MySQL and Oracle, in terms of vertical and horizontal expansion. Vertical expansion Vertical expansion improves database performance by increasing the processing power of the server. This can be achieved by adding more CPU cores and expanding memory capacity
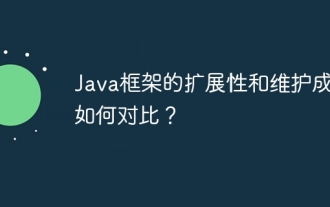
When choosing a Java framework, Spring Framework is known for its high scalability, but as complexity increases, maintenance costs also increase. In contrast, Dropwizard is generally less expensive to maintain but less scalable. Developers should evaluate frameworks based on specific needs.
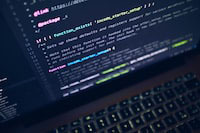
In modern software development, creating scalable, maintainable applications is crucial. PHP design patterns provide a set of proven best practices that help developers achieve code reuse and increase scalability, thereby reducing complexity and development time. What are PHP design patterns? Design patterns are reusable programming solutions to common software design problems. They provide a unified and common way to organize and structure code, thereby promoting code reuse, extensibility, and maintainability. SOLID Principles The PHP design pattern follows the SOLID principles: S (Single Responsibility): Each class or function should be responsible for a single responsibility. O (Open-Closed): The class should be open for extension, but closed for modification. L (Liskov replacement): subclasses should
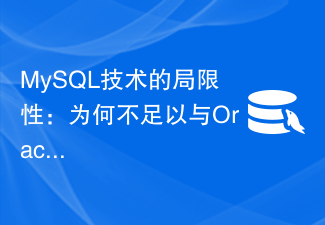
The limitations of MySQL technology: Why is it not enough to compete with Oracle? Introduction: MySQL and Oracle are one of the most popular relational database management systems (RDBMS) in the world today. While MySQL is very popular in web application development and small businesses, Oracle has always dominated the world of large enterprises and complex data processing. This article will explore the limitations of MySQL technology and explain why it is not enough to compete with Oracle. 1. Performance and scalability limitations: MySQL is
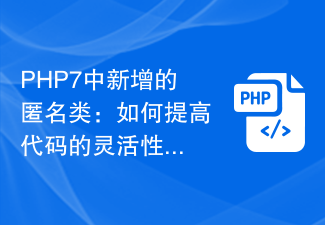
PHP7 adds the feature of anonymous classes, which brings greater flexibility and scalability to developers. Anonymous classes are classes that are not explicitly named and can be defined on the fly where needed, making it easy to use the functionality of the class without having to name it. Anonymous classes are particularly useful in certain scenarios, such as in the case of callback functions, closures, and single-use classes. Using anonymous classes can better organize the code, avoid defining a temporary class, and make the code more concise and readable. The following is a few specific examples to show how to use anonymous classes to improve
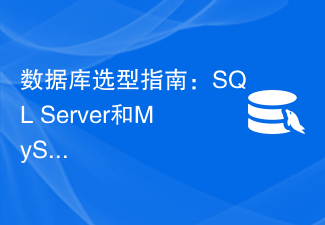
Database Selection Guide: Comparing SQL Server and MySQL, who is better? Introduction: In the era of rapid development of modern technology, data has become one of the indispensable assets of enterprises. In order to store and manage data efficiently, it is particularly important to choose a suitable database management system (DBMS). For many enterprises, the two most common choices are SQL Server and MySQL. This article will compare the two in terms of performance, scalability, security and cost to help enterprises make more informed decisions.
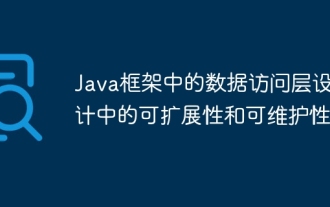
Following the principles of scalability and maintainability, the Java framework data access layer can achieve: Scalability: Abstract data access layer: Separate logic and database implementation Support multiple databases: Respond to changes in requirements Use a connection pool: Manage connections to prevent exhaustion Maintainability: Clear naming convention: Improves readability Separation of queries and code: Enhances clarity and maintainability Use logging: Eases debugging and tracing system behavior
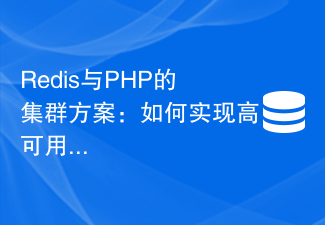
Redis and PHP cluster solution: How to achieve high availability and scalability Introduction: Redis is an open source, high-performance in-memory database that is often used to build fast and scalable applications. As a popular server-side scripting language, PHP can be used with Redis to achieve high availability and scalability cluster solutions. This article will introduce how to use Redis and PHP to build a high-availability and scalable cluster, and explain in detail through code examples. 1. Construction, installation and configuration of Redis cluster Re
