


The relationship between encapsulation and object-oriented programming in PHP
The relationship between encapsulation and object-oriented programming in PHP
In object-oriented programming, encapsulation is a very important concept. It allows developers to wrap data and methods in a class and provide external interfaces to access and operate data while hiding internal implementation details. In PHP, encapsulation is inseparable from object-oriented programming.
The main purpose of encapsulation is to achieve information hiding. By encapsulating data and methods in classes, we can effectively control access to data and prevent it from being modified inappropriately. Doing so helps improve the security and maintainability of your code.
In PHP, you can use access modifiers to control the access level of properties and methods in a class. Common access modifiers include public, protected, and private. public means public and can be accessed inside and outside the class; protected means protected and can only be accessed within the current class or its subclasses; private means private and can only be accessed within the current class.
Below we use a specific code example to illustrate the relationship between encapsulation and object-oriented programming:
class Person { private $name; protected $age; public $gender; public function __construct($name, $age, $gender) { $this->name = $name; $this->age = $age; $this->gender = $gender; } public function getName() { return $this->name; } public function getAge() { return $this->age; } public function setAge($age) { if ($age >= 0 && $age <= 120) { $this->age = $age; } else { echo "Invalid age!"; } } } $person = new Person("John", 30, "Male"); echo $person->getName(); // 输出:John echo $person->getAge(); // 输出:30 $person->setAge(40); echo $person->getAge(); // 输出:40 $person->setAge(150); // 输出:Invalid age!
In the above code example, we define a Person class, including private, Properties and methods with three different access levels: protected and public. The name attribute is private and can only be accessed within the class; the age attribute is protected and can be accessed within the class and subclasses; the gender attribute is public and can be accessed from anywhere.
We also define the constructor __construct() to initialize the properties of the class, and the getName(), getAge() and setAge() methods to get and set the name and age properties of the object.
Through encapsulation, we can effectively control properties. For example, in the setAge() method, we added a conditional judgment to ensure that the age passed in is within the valid range. If the age is outside the range, an error message will be output.
To sum up, encapsulation is an important principle of object-oriented programming. PHP implements the encapsulation of properties and methods through access modifiers. Reasonable use of encapsulation can improve the security, readability and maintainability of the code, while also enhancing the modularity and reusability of the code. Through the above sample code, we can better understand the relationship between encapsulation and object-oriented programming.
The above is the detailed content of The relationship between encapsulation and object-oriented programming in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


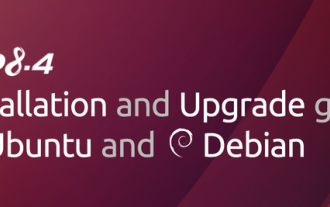
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
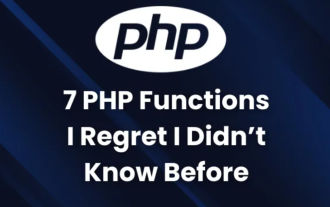
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
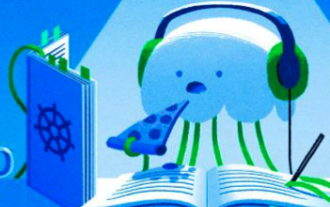
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
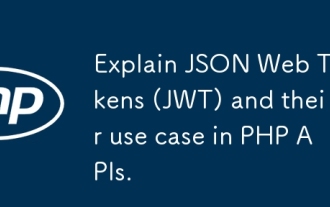
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
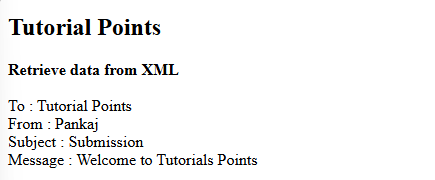
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
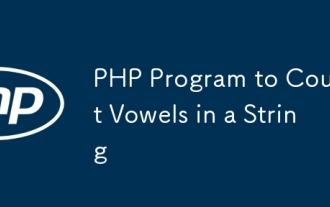
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
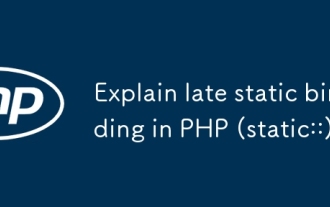
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
