


PHP Session cross-domain session management and authentication
PHP Session Cross-domain session management and authentication
Introduction:
In modern network application development, session management and authentication are very important Security Measures. PHP provides a convenient and powerful session management mechanism - PHP Session. However, when applications require cross-domain access, session management and authentication become more complex. This article will introduce how to use PHP Session for cross-domain session management and authentication, and give specific code examples.
1. What is session management and authentication
Session management refers to the server-side method of tracking user activity on the website. In PHP, sessions are managed by PHP Session. PHP Session uses a cookie-based mechanism to uniquely identify the user and store the user's session data on the server side. Through session management, we can keep users logged in when they visit different pages and share data between different pages.
Authentication is the process of verifying a user's identity. Through identity verification, we can confirm that the user is a legitimate user and provide them with corresponding permissions and services. PHP provides various authentication mechanisms such as Basic Authentication, Forms Authentication, OAuth Authentication, etc.
2. Basic use of PHP Session
Before using PHP Session, we need to call the session_start()
function to start the session. Once a session is started, session data can be accessed and modified using the $_SESSION
global variables.
<?php session_start(); // 在会话中存储数据 $_SESSION['user_id'] = 1; $_SESSION['username'] = 'John'; // 访问会话数据 echo $_SESSION['username']; // 输出:John // 销毁会话 session_destroy(); ?>
3. Cross-domain session management
When our application requires cross-domain access, session management becomes more complicated. Due to browser origin policy restrictions, we cannot directly share session data between cross-domain servers. In this case, we can implement cross-domain session management through the following methods.
- JSON Web Tokens (JWT)
JWT is a method of authentication in a cross-domain environment. It uses encryption to store the user's identity information in a token and sends the token to the client, which carries the token in subsequent requests for authentication. The server can parse the token and verify the user's identity.
The following is a sample code for cross-domain session management using JWT:
<?php use FirebaseJWTJWT; // 生成JWT令牌 function generateToken($userId, $username) { $key = 'secret_key'; $payload = array( "user_id" => $userId, "username" => $username, "exp" => time() + 3600 ); return JWT::encode($payload, $key); } // 验证JWT令牌 function validateToken($token) { $key = 'secret_key'; try { $decoded = JWT::decode($token, $key, array('HS256')); return $decoded->user_id; } catch (Exception $e) { return false; } } // 在登录时生成并发送JWT令牌 function login() { // 验证用户输入的用户名和密码 // ... // 生成JWT令牌 $token = generateToken($userId, $username); // 将令牌发送给客户端 setcookie('token', $token, time() + 3600, '/', 'example.com', false, true); } // 在每个请求中验证JWT令牌 function validateSession() { $token = $_COOKIE['token']; $userId = validateToken($token); if(!$userId) { // 未通过身份验证 // ... } else { // 已通过身份验证 // ... } } ?>
- Server-side shared Session
Another method of cross-domain session management is to use Shared storage on the server side, such as Redis or a database. When a user logs in, the server generates a unique session ID and stores the session data in shared storage. In a cross-domain server, session data can be obtained through the session ID, and session management and authentication can be implemented.
The following is a sample code for using a shared session:
<?php // 在登录时生成会话ID,并存储会话数据 function login() { // 验证用户输入的用户名和密码 // ... // 生成会话ID $session_id = uniqid(); // 存储会话数据到共享存储 redis_set($session_id, array("user_id" => $userId, "username" => $username)); // 将会话ID发送给客户端 setcookie('session_id', $session_id, time() + 3600, '/', 'example.com', false, true); } // 在每个请求中验证会话ID function validateSession() { $session_id = $_COOKIE['session_id']; $session_data = redis_get($session_id); if(!$session_data) { // 未通过身份验证 // ... } else { // 已通过身份验证 // ... } } ?>
Summary:
Session management and authentication in a cross-domain environment is a complex and critical task. This article introduces two methods to implement cross-domain session management and authentication, and gives specific code examples. Through appropriate methods and technologies, we can ensure the security and consistency of users' session and identity information during cross-domain access.
The above is the detailed content of PHP Session cross-domain session management and authentication. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


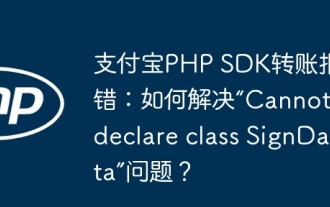
Alipay PHP...
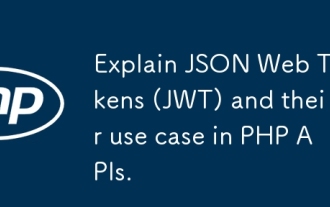
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
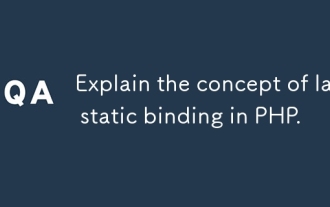
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
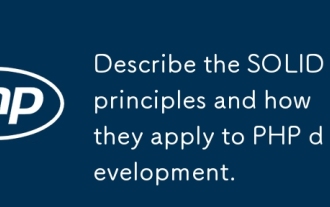
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
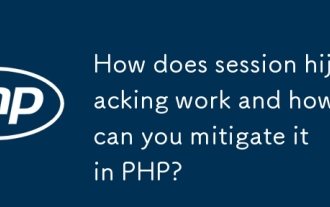
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
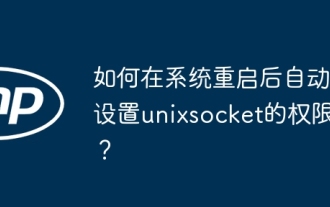
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
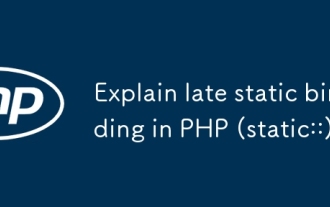
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
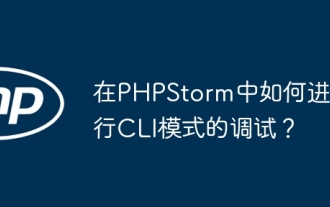
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
