


Data backup and restoration of PHP applications through Docker Compose, Nginx and MariaDB
Data backup and restoration of PHP applications through Docker Compose, Nginx and MariaDB
With the rapid development of cloud computing and containerization technology, more and more of applications choose to use Docker to deploy and run. In the Docker ecosystem, Docker Compose is a very popular tool that can define and manage multiple containers through a single configuration file.
This article will introduce how to use Docker Compose, Nginx and MariaDB to implement data backup and restoration of PHP applications. We'll use a sample project to demonstrate this process.
The sample project structure is as follows:
1 2 3 4 5 6 7 8 9 10 |
|
First, we need to create a docker-compose.yml
file to define the container configuration of the entire project. The sample configuration is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
|
In the above configuration, we defined three services: Nginx, MariaDB and PHP. The Nginx service is responsible for processing HTTP requests and forwarding the requests to the application on the PHP container; the MariaDB service provides database services; the PHP container runs the PHP application.
Next, we need to create an Nginx configuration file default.conf
for forwarding HTTP requests to the application on the PHP container. The sample configuration is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
In the above configuration, we defined two location blocks. The first location block is used to handle static file requests, such as images, CSS, and JavaScript files; the second location block is used to forward PHP script requests to the FastCGI process on the PHP container.
Then, we need to create a MariaDB container Dockerfile and initialization script init.sql
. The sample Dockerfile is as follows:
1 2 3 |
|
Sampleinit.sql
The script is used to create a mydb
database and a users
table:
1 2 3 4 5 6 7 8 |
|
Finally, we need to create a Dockerfile for the PHP container and a sample PHP application index.php
. The sample Dockerfile is as follows:
1 2 3 4 5 6 7 8 9 10 |
|
Sampleindex.php
The application is used to demonstrate the process of data backup and restoration:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
|
Now, we can run docker- compose up
command to start the entire project. Visit http://localhost
in the browser, and you should be able to see the inserted data and printed results.
In order to achieve data backup, we can add a Shell script to perform database backup operations regularly. The sample script is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
The above script first defines the backup directory and backup file name. It then uses the docker exec
command to execute the mysqldump
command to export the database and saves the exported data to a backup file.
In order to achieve data restoration, we can create a Shell script to perform database restore operations. The sample script is as follows:
1 2 3 4 5 6 7 8 9 10 11 |
|
The above script will use the docker exec
command to import the data in the backup file into the database.
Through the above steps, we successfully implemented data backup and restoration of PHP applications using Docker Compose, Nginx and MariaDB. By executing the backup script regularly, we can create a snapshot of the database so that we can restore it when needed. This provides our application with higher availability and fault tolerance.
The above is the detailed content of Data backup and restoration of PHP applications through Docker Compose, Nginx and MariaDB. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










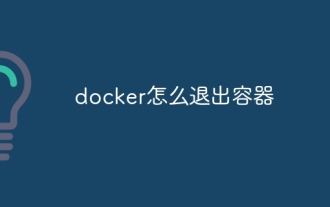
Four ways to exit Docker container: Use Ctrl D in the container terminal Enter exit command in the container terminal Use docker stop <container_name> Command Use docker kill <container_name> command in the host terminal (force exit)
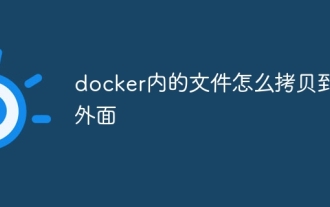
Methods for copying files to external hosts in Docker: Use the docker cp command: Execute docker cp [Options] <Container Path> <Host Path>. Using data volumes: Create a directory on the host, and use the -v parameter to mount the directory into the container when creating the container to achieve bidirectional file synchronization.
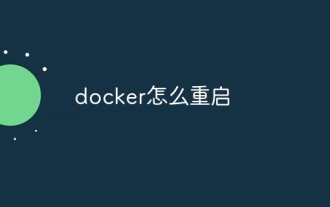
How to restart the Docker container: get the container ID (docker ps); stop the container (docker stop <container_id>); start the container (docker start <container_id>); verify that the restart is successful (docker ps). Other methods: Docker Compose (docker-compose restart) or Docker API (see Docker documentation).
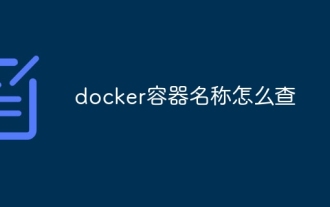
You can query the Docker container name by following the steps: List all containers (docker ps). Filter the container list (using the grep command). Gets the container name (located in the "NAMES" column).
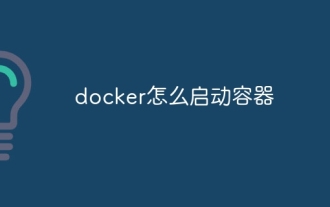
Docker container startup steps: Pull the container image: Run "docker pull [mirror name]". Create a container: Use "docker create [options] [mirror name] [commands and parameters]". Start the container: Execute "docker start [Container name or ID]". Check container status: Verify that the container is running with "docker ps".
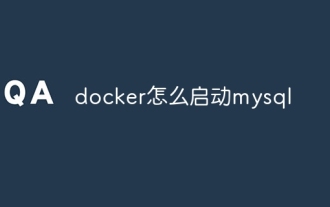
The process of starting MySQL in Docker consists of the following steps: Pull the MySQL image to create and start the container, set the root user password, and map the port verification connection Create the database and the user grants all permissions to the database
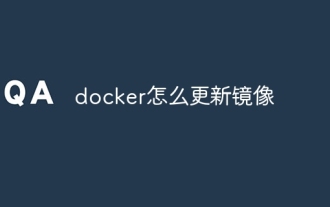
The steps to update a Docker image are as follows: Pull the latest image tag New image Delete the old image for a specific tag (optional) Restart the container (if needed)
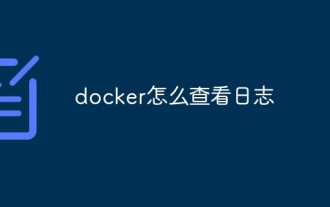
The methods to view Docker logs include: using the docker logs command, for example: docker logs CONTAINER_NAME Use the docker exec command to run /bin/sh and view the log file, for example: docker exec -it CONTAINER_NAME /bin/sh ; cat /var/log/CONTAINER_NAME.log Use the docker-compose logs command of Docker Compose, for example: docker-compose -f docker-com
