Scalability and flexibility of encapsulation in PHP
PHP is a powerful and flexible programming language, and its encapsulated scalability is a key feature. Encapsulation refers to grouping code and related functions together, hiding internal implementation details, and accessing these functions through public interfaces. This encapsulation brings many advantages, such as code maintainability, code reusability, security, etc. This article will illustrate the scalability and flexibility of encapsulation in PHP through specific code examples.
In PHP, class is the basic unit to achieve encapsulation. A class can contain attributes (variables) and methods (functions). Attributes are used to store object data, and methods are used to process these data and perform related operations. Through encapsulation, we can set the properties in the class to be private, which can only be accessed within the class and cannot be directly accessed from the outside. Through public methods, we can modify, read and operate properties, thereby ensuring data security.
The following is a simple example that shows how to define a class in PHP and use encapsulation to implement data access control:
class Person { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function getName() { return $this->name; } public function getAge() { return $this->age; } public function changeName($newName) { $this->name = $newName; } } $person = new Person("John Doe", 25); echo $person->getName(); // 输出 "John Doe" echo $person->getAge(); // 输出 25 $person->changeName("Jane Smith"); echo $person->getName(); // 输出 "Jane Smith"
In the above example, Person
The class contains two private properties $name
and $age
. We initialize these properties through the constructor __construct
. The getName
and getAge
methods are used to obtain the value of the attribute, and the changeName
method is used to modify the value of $name
. Since these methods are public, we can access and manipulate these properties from outside the class.
Encapsulation scalability can be achieved through inheritance. Inheritance means that one class inherits the properties and methods of another class, and can add or modify them on this basis. Through inheritance, we can build more specific and specialized classes. Here is an example that shows how to use inheritance to extend a base Person
class:
class Student extends Person { private $studentId; public function __construct($name, $age, $studentId) { parent::__construct($name, $age); $this->studentId = $studentId; } public function getStudentId() { return $this->studentId; } public function changeStudentId($newId) { $this->studentId = $newId; } } $student = new Student("Alice Smith", 20, "123456"); echo $student->getName(); // 输出 "Alice Smith" echo $student->getAge(); // 输出 20 echo $student->getStudentId(); // 输出 "123456" $student->changeName("Bob Brown"); echo $student->getName(); // 输出 "Bob Brown"
In the above example, we define a class that inherits from Person
Student
class. The Student
class adds a new private attribute $studentId
based on the parent class, and defines corresponding public methods to access and modify this attribute. This way we can easily extend and customize existing classes.
In addition to inheritance, PHP also provides an interface mechanism to implement polymorphism. An interface defines a set of methods, and a class can implement these interfaces and provide corresponding implementation code. Through interfaces, we can write scalable and flexible code to adapt to different needs. Here is an example that shows how to use interfaces to achieve polymorphism:
interface Shape { public function calculateArea(); } class Rectangle implements Shape { private $length; private $width; public function __construct($length, $width) { $this->length = $length; $this->width = $width; } public function calculateArea() { return $this->length * $this->width; } } class Circle implements Shape { private $radius; public function __construct($radius) { $this->radius = $radius; } public function calculateArea() { return 3.14 * $this->radius * $this->radius; } } $rectangle = new Rectangle(4, 5); echo $rectangle->calculateArea(); // 输出 20 $circle = new Circle(3); echo $circle->calculateArea(); // 输出 28.26
In the above example, we define a Shape
interface which contains a calculateArea
method. The Rectangle
and Circle
classes respectively implement this interface and provide their own implementation code. In this way, we can call the methods of these objects polymorphically without caring about specific implementation details.
Through the above code examples, we can see that in PHP, the scalability and flexibility of encapsulation can be achieved through classes, inheritance, and interfaces. This encapsulation brings many benefits, such as improving code maintainability, readability, and reusability. At the same time, it also provides developers with more flexibility and scalability to adapt to different needs and scenarios. Whether we are building a simple application or a complex system, encapsulation is a very important concept that deserves our in-depth study and application.
The above is the detailed content of Scalability and flexibility of encapsulation in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
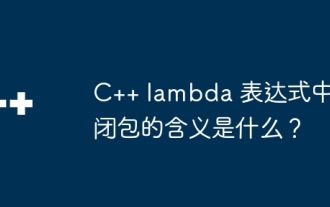
In C++, a closure is a lambda expression that can access external variables. To create a closure, capture the outer variable in the lambda expression. Closures provide advantages such as reusability, information hiding, and delayed evaluation. They are useful in real-world situations such as event handlers, where the closure can still access the outer variables even if they are destroyed.
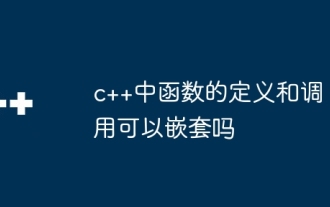
Can. C++ allows nested function definitions and calls. External functions can define built-in functions, and internal functions can be called directly within the scope. Nested functions enhance encapsulation, reusability, and scope control. However, internal functions cannot directly access local variables of external functions, and the return value type must be consistent with the external function declaration. Internal functions cannot be self-recursive.
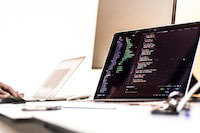
Access restrictions: Encapsulation limits access to internal data and sometimes it may be difficult to access necessary information. Potential inflexibility: Strict encapsulation can limit the customizability of code, making it difficult to adjust it to specific needs. Testing difficulty: Encapsulation may make it difficult to test the internal implementation because external access is restricted. Code redundancy: To maintain encapsulation, it is sometimes necessary to duplicate code, such as creating multiple getter and setter methods. Performance overhead: Accessing private members requires getter and setter methods, which may incur additional performance overhead. Weigh privacy and maintainability: When weighing privacy and maintainability, the following factors should be considered: Security requirements: If the data is highly sensitive, the priority for privacy may be high

Using STL function objects can improve reusability and includes the following steps: Define the function object interface (create a class and inherit from std::unary_function or std::binary_function) Overload operator() to define the function behavior in the overloaded operator() Implement the required functionality using function objects via STL algorithms (such as std::transform)

Friend functions have an impact on the encapsulation of a class, including reducing encapsulation, increasing attack surface, and improving flexibility. It can access the private data of the class. For example, the printPerson function defined as a friend of the Person class in the example can access the private data members name and age of the Person class. Programmers need to weigh the risks and benefits and use friend functions only when necessary.
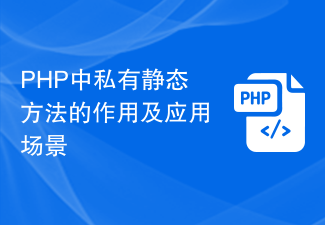
The role and application scenarios of private static methods in PHP In PHP programming, a private static method is a special method type. It can only be accessed within the class in which it is defined and cannot be directly called from the outside. Private static methods are usually used for the internal logic implementation of a class, providing a way to encapsulate and hide details. At the same time, they have the characteristics of static methods and can be called without instantiating the class object. The following will discuss the role and application scenarios of private static methods, and provide specific code examples. Function: encapsulate and hide implementation details: private static
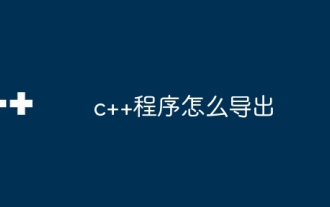
Symbols, including functions, variables, and classes, are exported in C++ through the extern "C" keyword. Exported symbols are extracted and used according to C language rules between compilation units or when interacting with other languages.
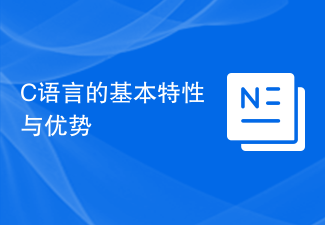
Basic Characteristics and Advantages of C Language As a widely used programming language, C language has many unique characteristics and advantages, making it an important tool in the field of programming. This article will explore the basic features of the C language and its advantages, and explain it with specific code examples. 1. The basic characteristics of C language are concise and efficient: The syntax of C language is concise and clear, and it can implement complex functions with less code, so the programs written are efficient and readable. Procedural programming: C language mainly supports procedural programming, that is, executing statements in sequence
