Encapsulated performance tuning methods in PHP
Encapsulation in PHP refers to encapsulating a series of related functions in a module or class, making the code more modular and easy to maintain and reuse. However, encapsulation may also lead to certain performance issues. This article will introduce some performance tuning methods for PHP encapsulation and provide specific code examples to help developers optimize the performance of their PHP applications.
- Avoid too deep nesting of function levels
Function calls will cause a certain amount of overhead. If the function level is nested too deep, it will increase the frequency and cost of function calls. . Therefore, try to avoid nesting functions too deeply. The following is a sample code:
// 不推荐的写法 function funcA(){ // 一些逻辑处理 funcB(); } function funcB(){ // 一些逻辑处理 funcC(); } function funcC(){ // 一些逻辑处理 } // 推荐的写法 function funcA(){ // 一些逻辑处理 // funcB(); // funcC(); } function funcB(){ // 一些逻辑处理 } function funcC(){ // 一些逻辑处理 }
- Merge multiple small functions into one large function
Frequently calling small functions will increase the function calling overhead. Therefore, multiple small functions will be combined into one large function. Merging functions into one large function can reduce the number of function calls and improve performance. The following is a sample code:
// 不推荐的写法 function funcA(){ // 一些逻辑处理 } function funcB(){ // 一些逻辑处理 } function funcC(){ // 一些逻辑处理 } // 推荐的写法 function funcABC(){ // 一些逻辑处理 // funcA(); // funcB(); // funcC(); }
- Reduce the visibility of the method
The visibility of the method refers to the scope within which the method can be accessed. When the visibility of a method is high, the overhead of method calls increases. Therefore, the visibility of methods can be reduced by making some methods that do not need to be called externally private or protected. The following is a sample code:
// 不推荐的写法 class MyClass{ public function funcA(){ // 一些逻辑处理 } public function funcB(){ // 一些逻辑处理 $this->funcA(); } } // 推荐的写法 class MyClass{ private function funcA(){ // 一些逻辑处理 } public function funcB(){ // 一些逻辑处理 $this->funcA(); } }
- Reduce access to properties and methods
Access to properties and methods will involve overhead such as memory reading and function calls. Therefore, in actual development, performance can be improved by reducing the number of accesses to properties and methods. The following is a sample code:
// 不推荐的写法 class MyClass{ private $attribute; public function setAttribute($value){ $this->attribute = $value; } public function getAttribute(){ return $this->attribute; } } $myObj = new MyClass(); $myObj->setAttribute(5); echo $myObj->getAttribute(); // 推荐的写法 class MyClass{ private $attribute; public function setAttribute($value){ $this->attribute = $value; } public function getAttribute(){ return $this->attribute; } } $myObj = new MyClass(); $myObj->setAttribute(5); $attribute = $myObj->getAttribute(); echo $attribute;
Through the above optimization methods, the performance of PHP applications can be improved, especially when facing large and complex applications. When performing performance tuning, developers should choose appropriate optimization methods based on specific business scenarios and needs, and conduct testing and adjustments based on actual conditions.
The above is the detailed content of Encapsulated performance tuning methods in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


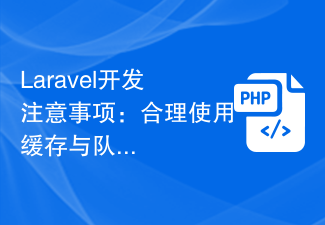
Laravel is a very popular PHP development framework. It provides rich functions and convenient development methods, which can help developers quickly build stable and reliable web applications. During the development process of Laravel, it is very important to use cache and queue properly. This article will introduce some precautions to help developers make better use of cache and queue. 1. Reasonable use of cache The definition and function of cache Cache is a technology that temporarily stores frequently used data in memory, which can greatly improve the response speed of the system.
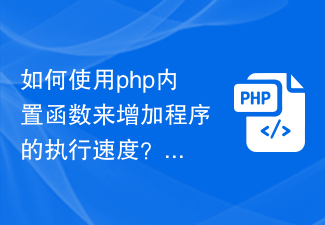
How to use PHP built-in functions to increase program execution speed? As the complexity of network applications increases, program execution speed becomes a very important consideration. As a widely used server-side scripting language, PHP is particularly critical for improving program execution speed. This article will introduce some techniques for using PHP's built-in functions to increase program execution speed, and provide specific code examples. Using String Processing Functions String processing is one of the operations that is often required in developing web applications. Use within PHP
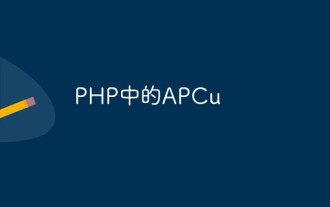
APCuAPCu (UserCacheforPHP) in PHP is a caching mechanism that can be used to improve the performance and responsiveness of applications. APCu is a lightweight cache that can be used to cache PHP scripts and other related data. It is a PHP kernel extension module, available in PHP5.4 and above. The role of APCu APCu is mainly used to cache data in PHP scripts, including variable values, objects, function return values, SQL query results, file lists and
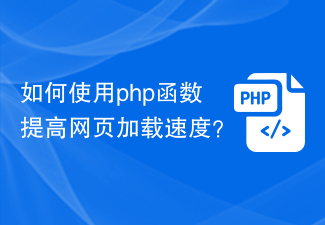
How to use PHP functions to improve web page loading speed? As the Internet develops, the loading speed of web pages is crucial to user experience and search engine rankings. As a commonly used server-side scripting language, PHP can effectively improve the loading speed of web pages by optimizing the use of PHP functions. This article will introduce some commonly used PHP functions and their specific code examples to help readers improve the performance of web pages. Use caching to reduce the number of database queries. The use of cache can effectively reduce the number of database queries in web pages and improve the performance of web pages.
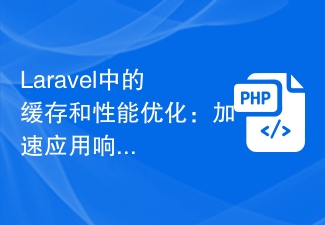
Caching and Performance Optimization in Laravel: Accelerating Application Response and Processing Performance optimization is a very important task when developing web applications. A high-performance application provides a better user experience and is more scalable. In the Laravel framework, caching and performance optimization are two very important topics. This article will introduce how to use Laravel's caching system to speed up application response and processing. Introduction to Laravel Caching System Laravel provides a powerful
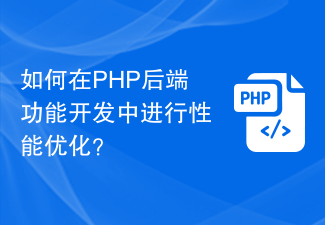
How to optimize performance in PHP backend function development? PHP is a popular server-side scripting language that is widely used for web development. However, PHP's performance can face challenges when developing large applications. This article will introduce some performance optimization techniques in PHP back-end function development and provide code examples to help you understand better. Use Proper Data Structures In PHP, using the correct data structures can significantly improve performance. For example, when you need to store large amounts of data, using arrays is more efficient than using objects. use array
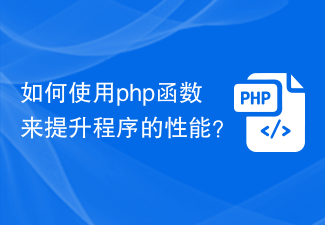
How to use PHP functions to improve program performance? Performance is a very important factor when developing web applications. Users expect fast response and efficient operating experience. PHP is a popular server-side development language that provides many built-in functions to accomplish various tasks. When writing PHP code, reasonable use of these functions can significantly improve the performance of the program. This article will introduce some commonly used PHP functions and give specific code examples to help developers optimize their programs. 1. Use strlen(
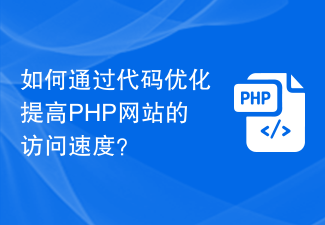
How to improve the access speed of PHP website through code optimization? Introduction: In today's Internet era, users have put forward higher requirements for website access speed. When users visit a slow website, it's easy for them to become impatient and even choose to abandon browsing. Therefore, improving your website's access speed is crucial to your website's success. In PHP website development, the access speed of the website can be significantly improved by optimizing the code. This article will introduce some common code optimization techniques and provide corresponding sample code. 1. Reduce the number of database queries
