


PHP trait DTO: key technology to improve data transmission efficiency
PHP trait DTO: Key technology to improve data transmission efficiency
Introduction:
In modern software development, data transmission is a very important link. In most applications, data needs to be transferred from one place to another, such as from the database to the front-end page, from the front-end form to the back-end processing logic, etc. The efficiency of data transmission directly affects the performance and user experience of the entire system. In order to improve the efficiency of data transfer, we can use PHP's trait DTO (Data Transfer Object) technology. This article will introduce the concept and advantages of trait DTO, and illustrate its use through specific code examples.
What is trait DTO?
trait DTO is a PHP programming technology used to transfer data from one place to another to improve the efficiency of data transfer. It can encapsulate data in a unified object and provide some convenient methods to access and manipulate data. Trait DTO is similar to the traditional DTO pattern, but is implemented using traits, making the code more concise and flexible.
Trait Advantages of DTO:
- Improve code reusability: By using traits, we can encapsulate the logic of data transmission in a trait and reference it in multiple classes This trait enables code reuse.
- Improve data transmission efficiency: trait DTO can encapsulate multiple related data in one object and transmit the object at once instead of transmitting each data one by one, thus reducing the number of network requests. Improved data transmission efficiency.
- Simplify code logic: Using trait DTO can encapsulate the logic and details of data transmission in an independent trait, making the code more concise and easier to maintain.
Specific code example:
The following is a simple example code that demonstrates how to use trait DTO to improve data transmission efficiency:
trait UserDTO { protected $id; protected $username; protected $email; public function setId($id) { $this->id = $id; } public function getId() { return $this->id; } public function setUsername($username) { $this->username = $username; } public function getUsername() { return $this->username; } public function setEmail($email) { $this->email = $email; } public function getEmail() { return $this->email; } } class UserController { use UserDTO; public function getUser(int $id) { // 模拟从数据库获取用户数据 $userData = [ 'id' => $id, 'username' => 'John Doe', 'email' => 'john.doe@example.com', ]; // 创建DTO对象并设置属性值 $user = new User(); $user->setId($userData['id']); $user->setUsername($userData['username']); $user->setEmail($userData['email']); return $user; } } // 调用示例 $userController = new UserController(); $user = $userController->getUser(1); echo "ID: " . $user->getId() . "<br>"; echo "Username: " . $user->getUsername() . "<br>"; echo "Email: " . $user->getEmail() . "<br>";
In the above example, we define A UserDTO trait is created, which contains some commonly used user properties and access methods. Then the trait is referenced in the UserController class, a User object is created in the getUser method, and the corresponding property values are set. Finally, in the calling example, we can access and output the user's attribute values directly through the User object.
Conclusion:
By using trait DTO technology, we can effectively improve the efficiency of data transmission. It can not only improve code reusability and simplify code logic, but also reduce the number of network requests, thereby improving system performance and user experience. In actual software development, we can flexibly use trait DTO to optimize data transmission according to specific needs and business logic.
The above is the detailed content of PHP trait DTO: key technology to improve data transmission efficiency. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


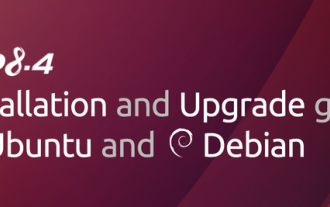
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
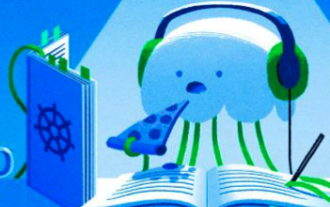
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
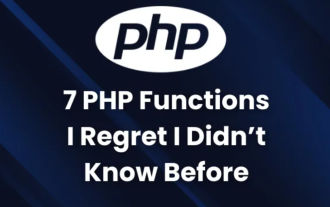
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
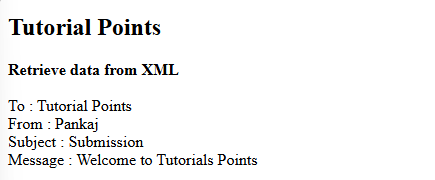
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
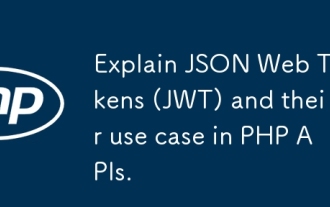
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
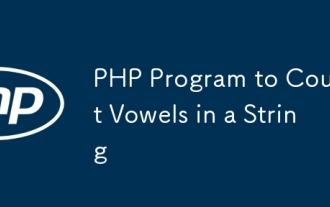
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
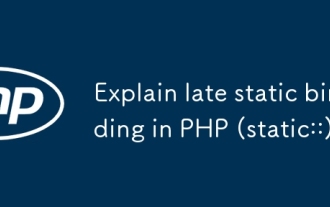
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
