How to implement user authentication and authorization in Vue project
How to implement user authentication and authorization in Vue projects
In recent years, the front-end framework Vue has gradually become the mainstream choice for web development. When developing a Vue project, user authentication and authorization are indispensable functions. This article will introduce in detail how to implement user authentication and authorization in the Vue project from the perspective of technical implementation, and provide specific code examples.
1. User authentication
User authentication refers to the process of verifying the user's identity to ensure that the user has legal permission to access the system. Common user authentication methods include username and password verification, third-party login, etc. The following uses username and password verification as an example to introduce the implementation of user authentication in the Vue project.
- Create the login page component
In the Vue project, you first need to create the login page component. This component contains input boxes for username and password and a login button. When the user clicks the login button, authentication is performed by calling the backend API.
The sample code is as follows:
<template> <div> <input v-model="username" placeholder="请输入用户名" /> <input v-model="password" placeholder="请输入密码" type="password" /> <button @click="login">登录</button> </div> </template> <script> export default { data() { return { username: '', password: '', }; }, methods: { login() { // 调用登录API,验证用户名和密码 // 如果验证成功,将用户信息存储到本地或会话存储中 }, }, }; </script>
- Verify username and password
In the login method, call the backend API to verify the correctness of the username and password. If the verification is successful, the user information can be saved to local storage or session storage to facilitate subsequent access and permission control.
The sample code is as follows:
methods: { login() { // 调用登录API,验证用户名和密码的正确性 api.login(this.username, this.password) .then(response => { const { token, userInfo } = response.data; // 将token和用户信息存储到本地存储或会话存储中 localStorage.setItem('token', token); localStorage.setItem('userInfo', JSON.stringify(userInfo)); // 跳转到主页或其他需要认证的页面 this.$router.push('/home'); }) .catch(error => { console.error('登录失败', error); }); }, },
- Authentication strategy
After user authentication, authentication usually needs to be performed on each page that requires authorization. . You can determine whether the user has permission to access the page by checking the user information in local storage or session storage.
The sample code is as follows:
beforeRouteEnter(to, from, next) { // 检查本地存储或会话存储中是否存在用户信息 const userInfo = localStorage.getItem('userInfo'); if (userInfo) { next(); } else { // 用户未登录,跳转到登录页面 next('/login'); } },
2. User authorization
User authorization refers to determining the user's access rights to system resources. In the Vue project, user authorization management can be achieved through the routing guard mechanism. The following uses routing guards as an example to introduce the implementation of user authorization in the Vue project.
- Define the routing table
In the Vue project, define the routing table, including pages that require permission control.
The sample code is as follows:
const routes = [ { path: '/home', component: Home, // 需要进行权限控制的页面,在路由元信息中定义所需的角色 meta: { requiresAuth: true, roles: ['admin', 'user'] }, }, // 其他路由... ];
- Authorization in the route guard
Authorization is performed before the route jump through Vue's navigation guard beforeEach judge. Determine whether the user has the permission to access the page based on the role information defined in the user information and routing meta-information.
The sample code is as follows:
router.beforeEach((to, from, next) => { const requiresAuth = to.matched.some(record => record.meta.requiresAuth); if (requiresAuth) { // 检查本地存储或会话存储中是否存在用户信息 const userInfo = localStorage.getItem('userInfo'); if (userInfo) { const { roles } = JSON.parse(userInfo); const requiredRoles = to.meta.roles; if (roles.some(role => requiredRoles.includes(role))) { // 用户具有访问页面的权限 next(); } else { // 用户权限不足,跳转到无权限页面 next('/denied'); } } else { // 用户未登录,跳转到登录页面 next('/login'); } } else { // 公开页面,无需授权 next(); } });
Through the above code examples, we can implement user authentication and authorization functions in the Vue project. User authentication ensures the legal identity of the user by verifying user name, password and other information. User authorization uses the route guard mechanism to determine whether the user has the authority to access the page before the route jumps. The implementation of these functions can help us build safe and reliable Vue projects.
The above is the detailed content of How to implement user authentication and authorization in Vue project. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
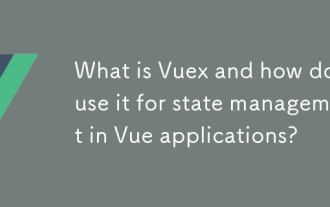
This article explains Vuex, a state management library for Vue.js. It details core concepts (state, getters, mutations, actions) and demonstrates usage, emphasizing its benefits for larger projects over simpler alternatives. Debugging and structuri
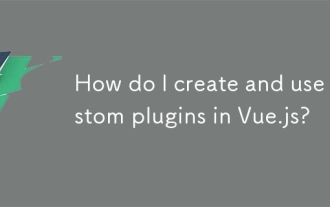
Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.
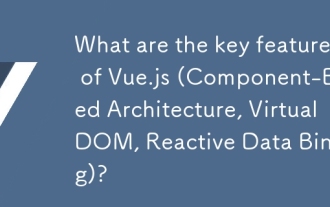
Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.

This article explores advanced Vue Router techniques. It covers dynamic routing (using parameters), nested routes for hierarchical navigation, and route guards for controlling access and data fetching. Best practices for managing complex route conf
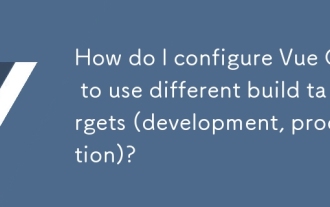
The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.
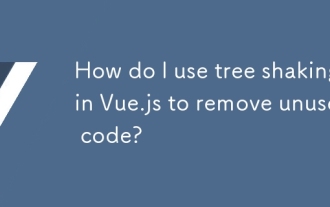
The article discusses using tree shaking in Vue.js to remove unused code, detailing setup with ES6 modules, Webpack configuration, and best practices for effective implementation.Character count: 159

The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.
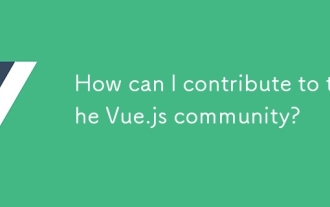
The article discusses various ways to contribute to the Vue.js community, including improving documentation, answering questions, coding, creating content, organizing events, and financial support. It also covers getting involved in open-source proje
