


Applicable scenarios and limitations of single column mode in PHP development
Applicable scenarios and limitations of single-column mode in PHP development, specific code examples are required
Title: Applicable scenarios and limitations of single-column mode in PHP development
Abstract: Single column mode is a commonly used design pattern, used to limit the number of instantiations of a class and provide a global access interface. This article will introduce the applicable scenarios, implementation methods and limitations of single column mode in PHP development, and provide specific code examples.
- Introduction
The singleton pattern is a creational design pattern that ensures that a class has only one instance and provides a global access point. In PHP development, the singleton pattern is often used to manage global resources, state or shared objects, and to ensure the number of instantiations of a certain class. - Applicable scenarios
The following are some applicable scenarios, you can consider using the singleton mode:
2.1 Global resource management
Some resources only require one in the application Examples, such as database connections, logging systems, etc. Using the singleton mode can ensure that there is only one instance globally, avoiding resource waste and conflicts.
2.2 Configuration Management
The configuration information of the application is usually shared globally. Using the singleton mode can easily manage and access the configuration information while ensuring global consistency.
2.3 Cache Management
Cache is an important means to improve application performance. Using the singleton mode can achieve global cache management and ensure the consistency and effectiveness of the cache.
2.4 Status Management
In some cases, it is necessary to maintain global status information, such as user login status, application running status, etc. The singleton pattern can easily manage and access this state information.
- Implementation method
There are many ways to implement the singleton pattern. The following is a commonly used implementation method (hungry Chinese style):
class Singleton { private static $instance; // 保存唯一实例的静态成员变量 private function __construct() {} // 私有构造函数,防止外部实例化 public static function getInstance() { if (!isset(self::$instance)) { self::$instance = new self(); } return self::$instance; } }
- Restrictions
Be aware of the following restrictions when using singleton mode:
4.1 Thread safety
In a multi-threaded environment, multiple threads may call the getInstance method at the same time, resulting in multiple The instance is created. You can ensure thread safety by locking, or use a lazy implementation.
4.2 The singleton mode can be inherited
The singleton mode allows inheritance, and subclasses can create new instances by overriding the getInstance method. If you need to restrict inheritance, you can make the constructor private and throw an exception in the getInstance method or return an instance of the parent class.
- Code example
The following is a code example of a log system based on singleton mode:
class Logger { private static $instance; private $logFileName; private function __construct($logFileName) { $this->logFileName = $logFileName; } public static function getInstance($logFileName) { if (!isset(self::$instance)) { self::$instance = new self($logFileName); } return self::$instance; } public function log($message) { $logTime = date('Y-m-d H:i:s'); $logMessage = "[$logTime] $message" . PHP_EOL; file_put_contents($this->logFileName, $logMessage, FILE_APPEND); } } // 使用示例 $logger = Logger::getInstance('app.log'); $logger->log('Hello, World!');
The above code implements a log system, which is obtained through the getInstance method An instance of the Logger class, and then call the log method to record the log. Due to the use of singleton mode, there will only be one Logger instance globally, which can facilitate log management and access.
Conclusion:
The singleton mode has a wide range of applicable scenarios in PHP development, and can be used for global resource management, configuration management, cache management and status management. However, when using the singleton mode, you need to pay attention to thread safety and inheritance issues, and make careful decisions during design and implementation. I hope the introduction and code examples in this article can help readers better understand and apply the singleton pattern.
The above is the detailed content of Applicable scenarios and limitations of single column mode in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
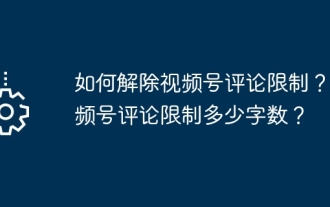
With the popularity of video accounts on social media, more and more people are beginning to use video accounts to share their daily lives, insights and stories. However, some users may experience comments being restricted, which can leave them confused and dissatisfied. 1. How to remove comment restrictions on video accounts? To lift the restriction on commenting on a video account, you must first ensure that the account has been properly registered and real-name authentication has been completed. Video accounts have requirements for comments. Only accounts that have completed real-name authentication can lift comment restrictions. If there are any abnormalities in the account, these issues need to be resolved before comment restrictions can be lifted. 2. Comply with the community standards of the video account. Video accounts have certain standards for comment content. If the comment involves illegal content, you will be restricted from speaking. To lift comment restrictions, you need to abide by the community of the video account
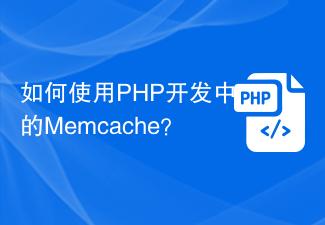
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
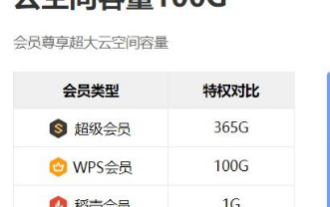
WPS is an office software that integrates comprehensive operations. You can now download WPS for use, but if you want to have more functions, you need to register as a member. Some people may wonder what is the maximum file size that a WPS member can upload? If you are a WPS member user, you can upload files up to 1G each time, and all files can add up to 365G. There may be some differences in different terminals, but the overall display is basically similar. What should I do if I cannot upload beyond the limit? We will explain it next. 1. When uploading files, such as cloud documents, there is a certain amount of space. If it exceeds the size, it cannot be uploaded. 2. Click on the membership logo, purchase membership according to your needs, and expand the space. 3. Coupons may appear occasionally, so don’t forget to use them.
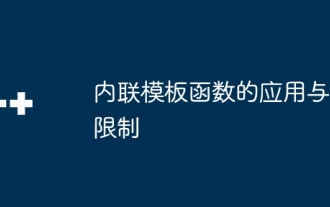
Inline template functions insert code directly into the call point without generating a separate function object. Applications include code optimization, performance improvement, constant evaluation, and code simplification. But be aware of its limitations, such as longer compilation times, increased code size, reduced debuggability, and limitations across compilation units.
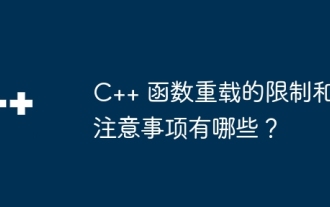
Restrictions on function overloading include: parameter types and orders must be different (when the number of parameters is the same), and default parameters cannot be used to distinguish overloading. In addition, template functions and non-template functions cannot be overloaded, and template functions with different template specifications can be overloaded. It's worth noting that excessive use of function overloading can affect readability and debugging, the compiler searches from the most specific to the least specific function to resolve conflicts.
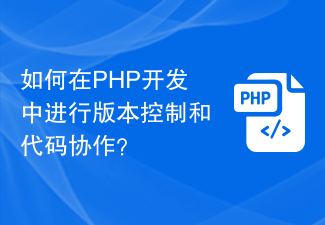
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
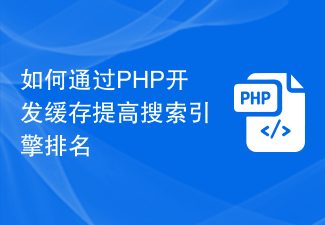
How to improve search engine rankings through PHP cache development Introduction: In today's digital era, the search engine ranking of a website is crucial to the website's traffic and exposure. In order to improve the ranking of the website, an important strategy is to reduce the loading time of the website through caching. In this article, we'll explore how to improve search engine rankings by developing caching with PHP and provide concrete code examples. 1. The concept of caching Caching is a technology that stores data in temporary storage so that it can be quickly retrieved and reused. for net
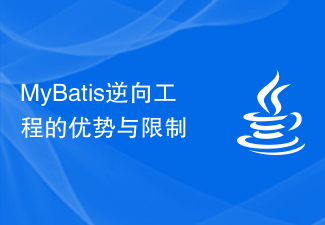
MyBatis is a popular persistence framework that provides reverse engineering functions, which allows developers to automatically generate entity classes, Mapper interfaces, and XML mapping files based on the table structure in the database. Reverse engineering is an important feature of MyBatis, which can greatly reduce the developer's workload and improve the maintainability of the code. However, reverse engineering also has some limitations. This article will introduce the advantages and limitations of MyBatis reverse engineering and illustrate it with specific code examples. First, let's
