


How to implement queue message confirmation and consumption failure handling in PHP and MySQL
How to implement queue message confirmation and consumption failure processing in PHP and MySQL
Queue is a common message delivery mechanism, which can help solve problems in the system To solve high concurrency issues, asynchronous processing and decoupling are achieved. In the design of the queue, message confirmation and consumption failure handling are very important links. This article will explore how to use PHP and MySQL to implement queue message confirmation and consumption failure handling, and provide specific code examples.
- Message confirmation
In the queue, message confirmation means that after the consumer successfully processes the message, it sends a confirmation signal to the queue, indicating that the message has been successfully consumed. This way, the queue can mark the message as complete and clean up related resources. In PHP, you can use the MySQL database to implement the message confirmation function.
First, we need to create a queue table to store messages. The structure of the table can be as follows:
CREATE TABLE `queue` ( `id` int(11) NOT NULL AUTO_INCREMENT, `message` text NOT NULL, `status` tinyint(1) NOT NULL DEFAULT '0', PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
Among them, id is the unique identifier of the message, message is the content of the message, status indicates the status of the message, 0 means unconfirmed, and 1 means confirmed.
Then, we can use the following code to implement the message confirmation function:
<?php function confirmMessage($id) { // 更新消息状态为已确认 $query = "UPDATE queue SET status = 1 WHERE id = :id"; $stmt = $pdo->prepare($query); $stmt->bindParam(':id', $id, PDO::PARAM_INT); $stmt->execute(); } // 示例:确认消息ID为1的消息 confirmMessage(1); ?>
By calling the confirmMessage function and passing in the message ID, the message status can be changed to confirmed.
- Consumption failure processing
In the queue, when the consumer is processing the message, exceptions or processing failures may occur. In order to ensure that messages are not lost, we need to implement a consumption failure handling mechanism. In PHP, you can use MySQL transactions to implement the consumption failure handling function.
First, we need to add a retry count field to the queue table to record the number of retries of the message. The structure of the table can be as follows:
ALTER TABLE `queue` ADD COLUMN `retry_count` int(11) NOT NULL DEFAULT '0' AFTER `status`;
Then, we can use the following code example to implement the consumption failure processing function:
<?php function consumeMessage($id) { // TODO: 处理消息的业务逻辑 // 事务开始 $pdo->beginTransaction(); // 更新消息状态为已消费 $query = "UPDATE queue SET status = 1 WHERE id = :id"; $stmt = $pdo->prepare($query); $stmt->bindParam(':id', $id, PDO::PARAM_INT); $stmt->execute(); // 提交事务 $pdo->commit(); } // 示例:消费消息ID为1的消息 try { consumeMessage(1); } catch (Exception $e) { // 发生异常时,进行消费失败处理 $pdo->rollBack(); // 回滚事务 $retryCount = getRetryCount(1); // 获取重试次数 if ($retryCount < 3) { // 重试处理 retryConsume(1, $retryCount); } else { // 重试次数达到上限,进行其他处理(例如记录日志) // ... } } function getRetryCount($id) { // 查询消息的重试次数 $query = "SELECT retry_count FROM queue WHERE id = :id"; $stmt = $pdo->prepare($query); $stmt->bindParam(':id', $id, PDO::PARAM_INT); $stmt->execute(); return $stmt->fetchColumn(); } function retryConsume($id, $retryCount) { // 更新消息重试次数 $query = "UPDATE queue SET retry_count = :retry_count WHERE id = :id"; $stmt = $pdo->prepare($query); $stmt->bindParam(':id', $id, PDO::PARAM_INT); $stmt->bindParam(':retry_count', $retryCount+1, PDO::PARAM_INT); $stmt->execute(); // 重试消费 consumeMessage($id); } ?>
In the above code, retry processing will be performed when consumption fails, and Determine whether to perform the next round of retries based on the number of retries. When the number of retries reaches the upper limit, other processing can be performed based on the actual situation, such as logging.
This article introduces the method of using PHP and MySQL to implement queue message confirmation and consumption failure processing, and provides specific code examples. By understanding and applying these methods, we can use queues more efficiently and safely to handle message delivery in the system.
The above is the detailed content of How to implement queue message confirmation and consumption failure handling in PHP and MySQL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
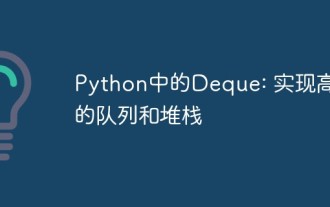
deque in Python is a low-level, highly optimized deque useful for implementing elegant and efficient Pythonic queues and stacks, which are the most common list-based data types in computing. In this article, Mr. Yun Duo will learn the following together with you: Start using deque to effectively pop up and append elements. Access any element in deque. Use deque to build an efficient queue. Start using deque to append elements to the right end of a Python list and pop up elements. The operations are generally very Efficient. If time complexity is expressed in Big O, then we can say that they are O(1). And when Python needs to reallocate memory to increase the underlying list to accept new elements, these
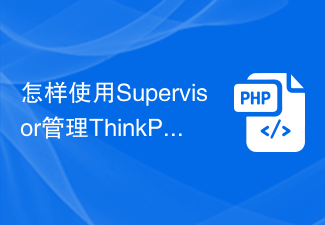
As web applications continue to develop, we need to handle a large number of tasks to maintain the stability and availability of the application. Using a queuing system is one solution. ThinkPHP6 provides a built-in queue system to manage tasks. However, handling a large number of tasks requires better queue management, which can be achieved using Supervisor. This article will introduce how to use Supervisor to manage ThinkPHP6 queues. Before that, we need to understand some basic concepts: queue system queue system is
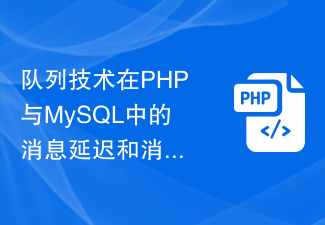
Application summary of queue technology in message delay and message retry in PHP and MySQL: With the continuous development of web applications, the demand for high concurrency processing and system reliability is getting higher and higher. As a solution, queue technology is widely used in PHP and MySQL to implement message delay and message retry functions. This article will introduce the application of queue technology in PHP and MySQL, including the basic principles of queues, methods of using queues to implement message delay, and methods of using queues to implement message retries, and give
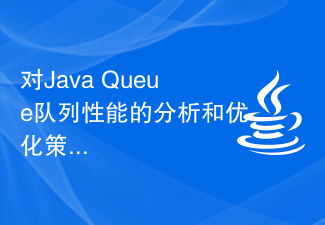
Performance Analysis and Optimization Strategy of JavaQueue Queue Summary: Queue (Queue) is one of the commonly used data structures in Java and is widely used in various scenarios. This article will discuss the performance issues of JavaQueue queues from two aspects: performance analysis and optimization strategies, and give specific code examples. Introduction Queue is a first-in-first-out (FIFO) data structure that can be used to implement producer-consumer mode, thread pool task queue and other scenarios. Java provides a variety of queue implementations, such as Arr

Queue in Java is a linear data structure with multiple functions. A queue has two endpoints and it follows the first-in-first-out (FIFO) principle for inserting and deleting its elements. In this tutorial, we will learn about two important functions of queues in Java, which are add() and Offer(). What is a queue? Queue in Java is an interface that extends the util and collection packages. Elements are inserted in the backend and removed from the frontend. Queues in Java can be implemented using classes such as linked lists, DeQueue, and priority queues. A priority queue is an extended form of a normal queue, where each element has a priority. The add() method of the queue is used to insert elements into the queue. It will define the element (as
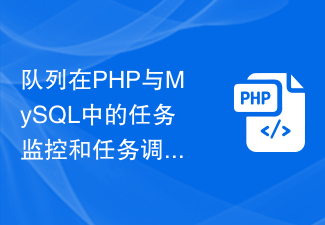
Implementation of queue task monitoring and task scheduling in PHP and MySQL Introduction In modern web application development, task queue is a very important technology. Through queues, we can queue some tasks that need to be executed in the background, and control the execution time and order of tasks through task scheduling. This article will introduce how to implement task monitoring and scheduling in PHP and MySQL, and provide specific code examples. 1. Working principle of queue Queue is a first-in-first-out (FIFO) data structure that can be used to
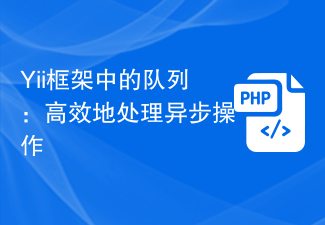
With the rapid development of the Internet, applications have become increasingly important to handle large numbers of concurrent requests and tasks. In such cases, handling asynchronous tasks is essential as this makes the application more efficient and better responsive to user requests. The Yii framework provides a convenient queue component that makes handling asynchronous operations easier and more efficient. In this article, we will explore the use and advantages of queues in the Yii framework. What is a Queue A queue is a data structure used to handle data in a first-in-first-out (FIFO) order. Team
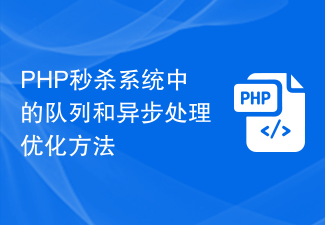
Queue and asynchronous processing optimization methods in the PHP flash sale system With the rapid development of the Internet, various preferential activities on e-commerce platforms, such as flash sales and rush sales, have also become the focus of users. However, this high concurrent user request is a huge challenge for traditional PHP applications. In order to improve the performance and stability of the system and solve the pressure caused by concurrent requests, developers need to optimize the flash sale system. This article will focus on the optimization methods achieved through queues and asynchronous processing in the PHP flash sale system, and give specific code examples.
