


How to implement queue data persistence and high availability in PHP and MySQL
How to implement queue data persistence and high availability in PHP and MySQL
Introduction:
With the rapid development of the Internet, a large amount of data is generated and processing put forward higher requirements on the performance of the system. Among many data processing strategies, queues are a widely used mechanism that can perform asynchronous communication and task processing between different modules. This article will focus on how to implement queue data persistence and high availability in PHP and MySQL, and provide specific code examples.
1. The concept and principle of queue data persistence
Queue data persistence refers to saving the data in the queue in a persistent storage medium to ensure that even if the system is abnormal or restarted, the data can be Resume and continue processing. In PHP, you can use a database system to achieve persistence of queue data, of which MySQL is one of the most commonly used databases.
- Create MySQL data table
First, we need to create a data table to store the queue data. The following is an example MySQL data table structure:
CREATE TABLE `queue` ( `id` int(11) unsigned NOT NULL AUTO_INCREMENT, `data` text NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
- Enqueuing operation
Enqueuing operation is to insert the data to be processed into the queue. The following is an example PHP code:
<?php function enqueue($data) { $mysqli = new mysqli("localhost", "username", "password", "database"); $stmt = $mysqli->prepare("INSERT INTO queue (data) VALUES (?)"); $stmt->bind_param("s", $data); $stmt->execute(); $stmt->close(); $mysqli->close(); } ?>
- Dequeue operation
The dequeue operation is to take out the data in the queue for processing. The following is an example PHP code:
<?php function dequeue() { $mysqli = new mysqli("localhost", "username", "password", "database"); $stmt = $mysqli->prepare("SELECT id, data FROM queue ORDER BY id ASC LIMIT 1"); $stmt->execute(); $stmt->bind_result($id, $data); $stmt->fetch(); $stmt->close(); // 处理数据 // ... // 删除已处理的数据 $stmt = $mysqli->prepare("DELETE FROM queue WHERE id = ?"); $stmt->bind_param("i", $id); $stmt->execute(); $stmt->close(); $mysqli->close(); } ?>
2. The concept and implementation of queue high availability
The high availability of queue refers to the system's ability to maintain continuous operation in the face of abnormal situations. Availability without losing mission data. In PHP and MySQL, high availability of queues can be achieved by using distributed queues and database transactions.
- The concept of distributed queue
Distributed queue stores the data in the queue distributedly on multiple nodes to ensure that other nodes can take over the task when a node fails. Commonly used distributed queue solutions include Redis, RabbitMQ, etc. - Use database transactions to achieve high availability of queues
MySQL provides transaction support to ensure that data can be rolled back and the consistency of the queue can be maintained in the event of exceptions during dequeuing operations. The following is an example PHP code:
<?php function dequeue() { $mysqli = new mysqli("localhost", "username", "password", "database"); $mysqli->autocommit(FALSE); // 开启事务 $stmt = $mysqli->prepare("SELECT id, data FROM queue ORDER BY id ASC LIMIT 1"); $stmt->execute(); $stmt->bind_result($id, $data); $stmt->fetch(); // 处理数据 // ... // 删除已处理的数据 $stmt = $mysqli->prepare("DELETE FROM queue WHERE id = ?"); $stmt->bind_param("i", $id); $stmt->execute(); // 提交事务 $mysqli->commit(); $stmt->close(); $mysqli->close(); } ?>
Conclusion:
Queue data persistence and high availability can be implemented in PHP and MySQL by storing queue data through the database and using transactions to ensure queue consistency. In addition, you can also consider using distributed queues to improve system availability and scalability. The above is the specific implementation method and sample code, I hope it will be helpful to readers.
The above is the detailed content of How to implement queue data persistence and high availability in PHP and MySQL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
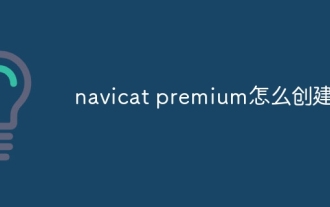
Create a database using Navicat Premium: Connect to the database server and enter the connection parameters. Right-click on the server and select Create Database. Enter the name of the new database and the specified character set and collation. Connect to the new database and create the table in the Object Browser. Right-click on the table and select Insert Data to insert the data.
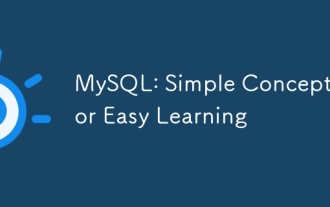
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
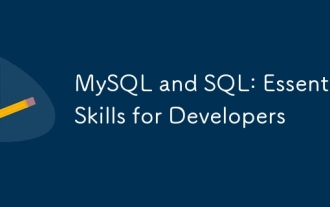
MySQL and SQL are essential skills for developers. 1.MySQL is an open source relational database management system, and SQL is the standard language used to manage and operate databases. 2.MySQL supports multiple storage engines through efficient data storage and retrieval functions, and SQL completes complex data operations through simple statements. 3. Examples of usage include basic queries and advanced queries, such as filtering and sorting by condition. 4. Common errors include syntax errors and performance issues, which can be optimized by checking SQL statements and using EXPLAIN commands. 5. Performance optimization techniques include using indexes, avoiding full table scanning, optimizing JOIN operations and improving code readability.
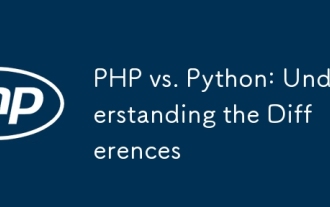
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
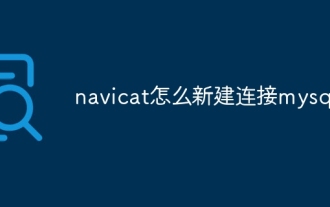
You can create a new MySQL connection in Navicat by following the steps: Open the application and select New Connection (Ctrl N). Select "MySQL" as the connection type. Enter the hostname/IP address, port, username, and password. (Optional) Configure advanced options. Save the connection and enter the connection name.
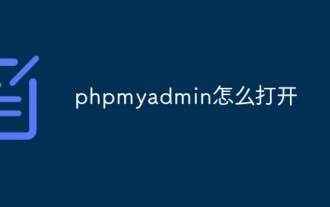
You can open phpMyAdmin through the following steps: 1. Log in to the website control panel; 2. Find and click the phpMyAdmin icon; 3. Enter MySQL credentials; 4. Click "Login".
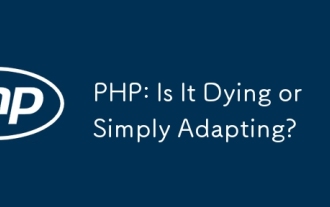
PHP is not dying, but constantly adapting and evolving. 1) PHP has undergone multiple version iterations since 1994 to adapt to new technology trends. 2) It is currently widely used in e-commerce, content management systems and other fields. 3) PHP8 introduces JIT compiler and other functions to improve performance and modernization. 4) Use OPcache and follow PSR-12 standards to optimize performance and code quality.
