


Implementing an efficient URL routing resolution solution in PHP
Efficient URL route resolution solution in PHP
When developing web applications, URL route resolution is a very important link. It can help us implement a friendly URL structure and map requests to the corresponding handlers or controllers. This article will introduce an efficient URL routing resolution solution and provide specific code examples.
1. The basic principle of URL routing parsing
The basic principle of URL routing parsing is to split the URL into different parts and match and map them based on the contents of these parts. Common URL structures include domain name, path and query parameters. In PHP, we can use the $_SERVER global variable to obtain the URL information of the current request.
2. Efficient URL routing resolution solution
In order to achieve efficient URL routing resolution, we can adopt the following solution:
- Use regular expressions to match URL paths
We can use regular expressions to match URL paths. For example, for the URL "/blog/article/123", we can use the regular expression "/^/blog/article/(d )$/" to match. This ensures that the structure of the URL path meets our requirements and extracts the required parameters from it. - Use mapping table for route mapping
We can use a mapping table to map the URL path to the corresponding handler or controller. The mapping table can be an array or a database table. For example, we can map the URL path "/blog/article/123" to the "article" method of the "BlogController" controller and pass the parameter "123". This makes it easy to manage and maintain routing rules. - Use regular expressions and variables to extract parameters
In the process of URL path matching, we can use regular expressions to extract the parameters in the path and pass them as variables to the corresponding handler or controller. For example, for the URL path "/blog/article/123", we can use the regular expression "/^/blog/article/(d )$/" to extract the parameter "123" and pass it to the "BlogController" controller. "article" method for processing.
3. Specific code examples
The following is a specific example code that implements a simple routing parser:
<?php // 定义路由映射表 $routes = [ '/^/blog/article/(d+)$/' => ['controller' => 'BlogController', 'method' => 'article'], // 添加更多的路由映射规则... ]; // 获取当前请求的URL路径 $requestPath = $_SERVER['REQUEST_URI']; // 遍历路由映射表进行匹配 foreach ($routes as $pattern => $route) { if (preg_match($pattern, $requestPath, $matches)) { // 提取参数 $params = array_slice($matches, 1); // 实例化控制器对象 $controller = new $route['controller'](); // 调用指定方法 call_user_func_array([$controller, $route['method']], $params); return; // 结束匹配 } } // 如果没有匹配到路由规则,则显示404页面 http_response_code(404); echo "404 Not Found"; ?>
In the above example code, we first A route mapping table $routes
is defined, which contains some common routing rules. Then, we get the URL path of the current request and traverse the route map table for matching. If the corresponding routing rule is matched, the corresponding controller object is instantiated according to the information in the mapping table and the specified method is called for processing.
It should be noted that in order to simplify the sample code, we did not involve the passing and processing of path parameters. In actual applications, more complex route parsing operations may be required.
Summary
URL route resolution plays an important role in the development of web applications. By using regular expression matching and mapping table route mapping, we can implement an efficient and flexible URL route parsing solution. I hope the introduction and code examples in this article are helpful to you.
The above is the detailed content of Implementing an efficient URL routing resolution solution in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


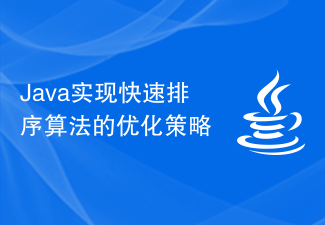
Title: Efficient method and code example to implement quick sort algorithm in Java Introduction: Quick sort is an efficient sorting algorithm, which is based on the idea of divide and conquer and has better performance under average circumstances. This article will introduce the implementation process of the quick sort algorithm in detail through Java code examples, along with performance optimization tips to improve its efficiency. 1. Algorithm principle: The core idea of quick sort is to select a benchmark element and divide the sequence to be sorted into two subsequences through one sorting. The elements of one subsequence are smaller than the benchmark element, and the elements of the other subsequence are smaller than the benchmark element.
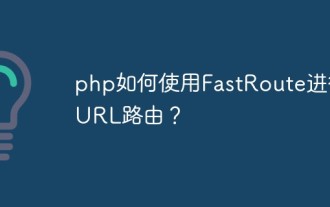
Routing is an inevitable part of modern web development. Routing serves as a bridge between web pages and applications, connecting HTTP requests and specific operations in the application. The purpose of routing is to map incoming URL requests to appropriate handlers so that we can initialize a page or perform other operations based on the URL request. In PHP, the routing system is usually implemented by using a third-party library called "FastRoute". FastRoute is a high-performance PHP routing library. it can
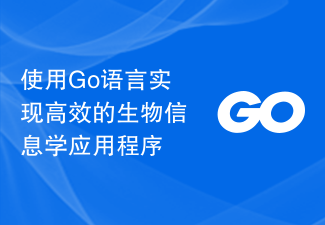
In the ever-evolving field of bioinformatics, developing efficient applications is crucial. Go is an option worth considering as a fast, concurrent, memory-safe language with the ability to manage large-scale data and networks. In this article, we will discuss how to implement efficient bioinformatics applications using Go language. Go language is an open source programming language developed by Google. It is easy to learn and efficient in execution. The concurrency model of Go language uses goroutine and channel, which can easily

Implementing an efficient URL route resolution solution in PHP When developing web applications, URL route resolution is a very important link. It can help us implement a friendly URL structure and map requests to the corresponding handlers or controllers. This article will introduce an efficient URL routing resolution solution and provide specific code examples. 1. The basic principle of URL route parsing The basic principle of URL route parsing is to split the URL into different parts and match and map them based on the contents of these parts. Common UR
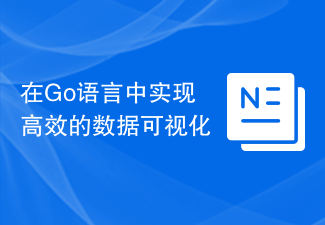
As the scale of data continues to expand, data visualization has become an increasingly popular topic. For data analysts, data scientists, programmers, product managers, etc. in different fields, being able to quickly visualize data has become increasingly important. When implementing data visualization, how to choose a suitable programming language is crucial. This article will introduce how to use Go language to achieve efficient data visualization. 1. Why choose Go language? Go language is an open source programming language developed by Google. it is a static type
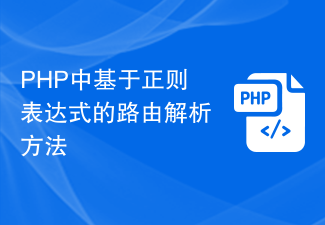
Route parsing method based on regular expressions in PHP In web-based applications, routing (Routing) is a very important concept. It is responsible for mapping user requests to corresponding handlers or controllers to achieve page rendering and processing. In PHP, we can use regular expressions to parse routes. Regular expressions are a powerful tool that can be used to match and extract specific patterns of strings. First, we need to define a routing table (RoutingTable), which is used to
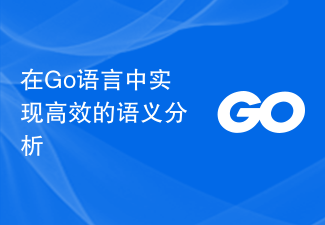
With the development of artificial intelligence and natural language processing, semantic analysis has become an increasingly important research field. In computer science, semantic analysis refers to converting natural language into machine-processable representations, which requires understanding the intent, emotion, context, etc. of the text. In this area, the efficiency and concurrency performance of the Go language have given us strong support. This article will introduce some technologies and methods to achieve efficient semantic analysis in Go language. To implement efficient semantic analysis in Go language using natural language processing library, we
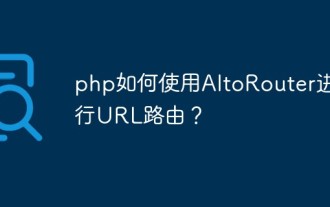
With the development of Internet technology, the development of Web applications increasingly requires the use of routing technology to better manage URL addresses and page jumps. In PHP development, AltoRouter can be used to implement routing functions very conveniently. 1. Introduction to AltoRouter AltoRouter is a simple, fast and flexible PHP router. It is a zero-dependency library that allows routing rules to be defined in the application and mapping URLs to corresponding controller actions. AltoRout
