


How to implement queue message filtering and message routing in PHP and MySQL
How to implement queue message filtering and message routing in PHP and MySQL
With the rapid development of the Internet, Message Queue (Message Queue) has become an important The communication mechanism plays a vital role in web development. Message queues can be used to implement functions such as decoupling, peak shaving, and asynchronous processing. This article will introduce how to implement message filtering and message routing in PHP and MySQL, and provide specific code examples.
- Message Queue
Message Queue is a typical "producer-consumer" model that can achieve asynchronous communication between different components. In PHP, you can use third-party extension libraries such as RabbitMQ, Kafka, etc. to implement message queues. In MySQL, database tables and triggers can be used to simulate message queues.
- Message filtering
Message filtering refers to filtering out qualified messages from the message queue based on specific conditions. In PHP, you can use conditional statements to filter messages. For example, the following code example demonstrates how to use conditional statements to filter messages:
// 消息队列中的消息 $messages = [ ['id' => 1, 'content' => '消息1', 'type' => 'A'], ['id' => 2, 'content' => '消息2', 'type' => 'B'], ['id' => 3, 'content' => '消息3', 'type' => 'A'], // ... ]; // 过滤条件 $type = 'A'; // 过滤消息 $filteredMessages = array_filter($messages, function($message) use ($type) { return $message['type'] === $type; }); // 输出结果 foreach ($filteredMessages as $message) { echo $message['content'] . PHP_EOL; }
In MySQL, you can use the WHERE clause to implement message filtering. For example, the following code example demonstrates how to use the WHERE clause to filter out eligible messages from the message queue list:
-- 消息队列表 CREATE TABLE messages ( id INT PRIMARY KEY AUTO_INCREMENT, content TEXT, type CHAR(1) ); -- 过滤条件 SET @type = 'A'; -- 过滤消息 SELECT * FROM messages WHERE type = @type;
- Message routing
Message routing refers to the The type or other properties of the message send the message to different destinations. In PHP, message routing can be implemented using a Switch statement or multiple if-else statements. The following code example demonstrates how to use the Switch statement to implement message routing:
// 消息 $message = [ 'type' => 'A', // ... ]; // 消息路由 switch ($message['type']) { case 'A': // 将消息发送到目的地A // ... break; case 'B': // 将消息发送到目的地B // ... break; default: // 将消息发送到默认目的地 // ... break; }
In MySQL, triggers can be used to implement message routing. The following code example demonstrates how to use triggers to send messages to different destination tables:
-- 目的地表A CREATE TABLE destination_a ( id INT PRIMARY KEY AUTO_INCREMENT, content TEXT ); -- 目的地表B CREATE TABLE destination_b ( id INT PRIMARY KEY AUTO_INCREMENT, content TEXT ); -- 消息队列表 CREATE TABLE messages ( id INT PRIMARY KEY AUTO_INCREMENT, content TEXT, type CHAR(1) ); -- 触发器:将消息发送到目的地A CREATE TRIGGER route_to_a AFTER INSERT ON messages FOR EACH ROW BEGIN IF NEW.type = 'A' THEN INSERT INTO destination_a (content) VALUES (NEW.content); END IF; END; -- 触发器:将消息发送到目的地B CREATE TRIGGER route_to_b AFTER INSERT ON messages FOR EACH ROW BEGIN IF NEW.type = 'B' THEN INSERT INTO destination_b (content) VALUES (NEW.content); END IF; END;
In summary, this article introduces the method of implementing message filtering and message routing in PHP and MySQL, and gives specific code examples. Through the reasonable use of message queues, we can better achieve decoupling and asynchronous communication between systems and improve the scalability and performance of the system. Hope this article is helpful to you!
The above is the detailed content of How to implement queue message filtering and message routing in PHP and MySQL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


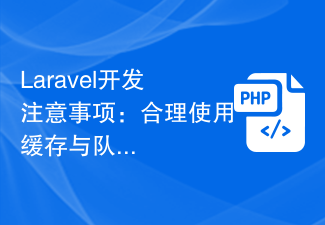
Laravel is a very popular PHP development framework. It provides rich functions and convenient development methods, which can help developers quickly build stable and reliable web applications. During the development process of Laravel, it is very important to use cache and queue properly. This article will introduce some precautions to help developers make better use of cache and queue. 1. Reasonable use of cache The definition and function of cache Cache is a technology that temporarily stores frequently used data in memory, which can greatly improve the response speed of the system.

Introduction to application scenarios of dead letter queues and delay queues in PHP and MySQL As Internet applications become more and more complex, the need to process a large number of messages and tasks is growing day by day. As a solution, queues can effectively implement asynchronous processing of tasks and improve the scalability and stability of the system. In queue applications, two common concepts are dead letter queues and delay queues. This article will introduce the application scenarios of these two concepts in PHP and MySQL, and provide specific code examples. The application scenarios of the dead letter queue are:
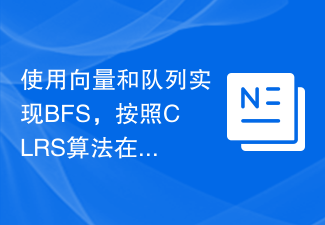
In the CLRS book, the BFS algorithm is described using vectors and queues. We have to use C++STL to implement this algorithm. First let's look at the algorithm. Algorithm BFS(G,s)−begin foreachvertexuinG.V-{s},do u.color:=white u.d:=infinity u.p:=NI
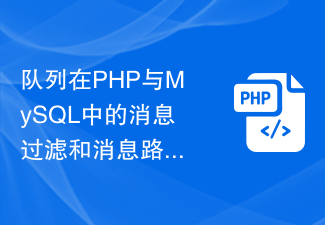
Queue's implementation of message filtering and message routing in PHP and MySQL With the rapid development of the Internet, message queue (MessageQueue), as an important communication mechanism, plays a crucial role in Web development. Message queues can be used to implement functions such as decoupling, peak shaving, and asynchronous processing. This article will introduce how to implement message filtering and message routing in PHP and MySQL, and provide specific code examples. Message queue Message queue is a typical "producer-consumer" model
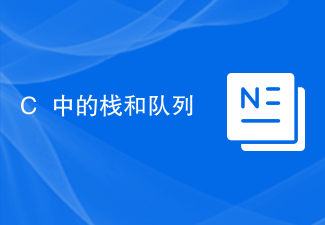
Introduction to stacks and queues in C++ Stacks and queues are commonly used data structures in C++, and they are widely used in programs. This article will introduce the concepts, usage and application scenarios of stacks and queues in detail. 1. The concept of stack Stack (Stack) is a linear data structure, which has the characteristics of "first in, last out". In the stack, the data pushed into the stack is closer to the bottom of the stack; the data pushed into the stack later is closer to the top of the stack. The main operations of the stack are push and pop. Pushing is to add data to the stack, and popping
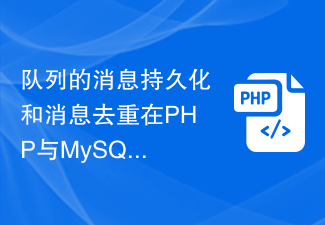
Application scenarios of queue message persistence and message deduplication in PHP and MySQL Queue is a common data structure and is widely used in asynchronous message processing, task scheduling, log collection and other scenarios in software development. Among them, message persistence and message deduplication are two important features of the queue, which can ensure message reliability and data consistency. In PHP and MySQL, queue applications can use Redis as the message middleware and MySQL to store and manage queue metadata. Specific examples are as follows. first
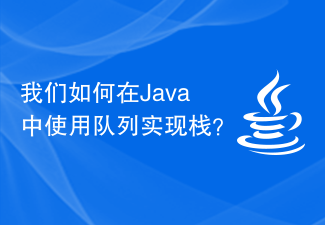
A stack is a subclass of the Vector class and represents a last-in-first-out (LIFO) stack of objects. The last element added to the top of the stack (In) can be the first element removed from the stack (Out). The Queue class extends the Collection interface and supports insertion and deletion operations using first-in-first-out (FIFO). We can also use queues to implement stacks in the following program. Example importjava.util.*;publicclassStackFromQueueTest{ Queuequeue=newLinkedList();
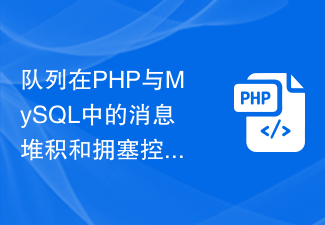
How to handle message accumulation and congestion control in queues in PHP and MySQL. With the rapid development of the Internet, the number of users of various websites and applications continues to increase, which puts higher requirements on the load capacity of the server. In this context, message queues have become a commonly used solution to solve the problem of message accumulation and congestion under high concurrent access. This article will introduce how to handle message accumulation and congestion control of queues in PHP and MySQL, and give specific code examples. In PHP we can use Re
