How to implement audio player function using JavaScript?
How to use JavaScript to implement the audio player function?
In web development, the audio player is a very common feature. By using JavaScript, we can easily implement a simple audio player. This article will introduce how to use JavaScript to implement audio player functions and provide specific code examples.
1. HTML structure
First, we need to create a basic HTML structure for the audio player. Typically, a simple audio player contains a play/pause button, a progress bar, and a volume adjuster. We can do this using the following HTML structure:
<div id="audioPlayer"> <audio id="audio" src="audio.mp3"></audio> <button id="playPauseButton">Play</button> <div id="progressBar"> <div id="progress"></div> </div> <input type="range" id="volumeSlider" min="0" max="1" step="0.1" value="1"> </div>
In this example, we use the <audio>
element to load the audio file. The src
attribute specifies the path to the audio file. <button>
element is used to play/pause audio. <div>
element is used to display the progress bar. <input type="range">
element is used to adjust the volume.
2. JavaScript function
Next, we need to use JavaScript to implement the audio player function. First, we need to define a global variable to reference the <audio>
element and add event listeners for the play/pause button and volume adjuster. The following is an implementation code example:
// 获取音频元素 var audio = document.getElementById("audio"); // 获取播放/暂停按钮 var playPauseButton = document.getElementById("playPauseButton"); // 获取音量调节器 var volumeSlider = document.getElementById("volumeSlider"); // 为播放/暂停按钮添加点击事件监听器 playPauseButton.addEventListener("click", function() { if (audio.paused) { audio.play(); playPauseButton.textContent = "Pause"; } else { audio.pause(); playPauseButton.textContent = "Play"; } }); // 为音量调节器添加事件监听器 volumeSlider.addEventListener("input", function() { audio.volume = volumeSlider.value; });
In this example, we use the getElementById
method to get the HTML element and add event listeners for buttons and volume adjusters. When the play/pause button is clicked, we determine whether it is currently playing or paused by checking the paused
property of the audio, and call the play
or pause
method accordingly to control the audio playback status. We also update the button's text content to reflect the current playback state. When the volume adjuster's value changes, we adjust the volume by setting the audio's volume
property.
3. Progress bar function
At the same time, we can also implement a progress bar to display the progress of audio playback. In order to achieve this function, we need to use a timer in JavaScript to regularly update the position of the progress bar. The following is an implementation code example:
// 获取进度条元素 var progressBar = document.getElementById("progress"); // 使用定时器定期更新进度条的位置 setInterval(function() { var progress = (audio.currentTime / audio.duration) * 100; progressBar.style.width = progress + "%"; }, 100);
In this example, we use the setInterval
function to update the position of the progress bar every 100 milliseconds. We determine the position of the progress bar by calculating the ratio of the current playing time of the audio to the total playing time, and set the width of the progress bar to reflect this ratio.
4. Summary
Through the above steps, we successfully implemented a simple audio player function using JavaScript. We control the play and pause of the audio, adjust the volume, and update the position of the progress bar by getting the HTML element and adding event listeners. I hope this article can help you understand how to use JavaScript to implement audio player functions, and provide some inspiration for your web development.
The above is the detailed content of How to implement audio player function using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


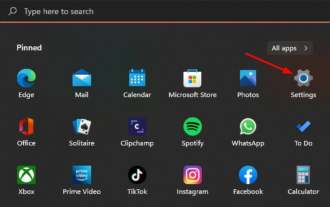
When listening to music or watching movies on a Win11 computer, if the speakers or headphones sound unbalanced, users can manually adjust the balance level according to their needs. So how do we adjust? In response to this problem, the editor has brought a detailed operation tutorial, hoping to help everyone. How to balance left and right audio channels in Windows 11? Method 1: Use the Settings app to tap the key and click Settings. Windows click System and select Sound. Choose more sound settings. Click on your speakers/headphones and select Properties. Navigate to the Levels tab and click Balance. Make sure "left" and
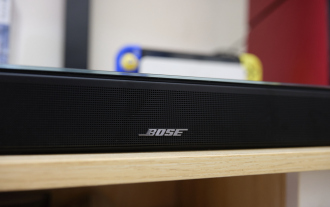
For as long as I can remember, I have had a pair of large floor-standing speakers at home. I have always believed that a TV can only be called a TV if it is equipped with a complete sound system. But when I first started working, I couldn’t afford professional home audio. After inquiring and understanding the product positioning, I found that the sound bar category is very suitable for me. It meets my needs in terms of sound quality, size and price. Therefore, I decided to go with the soundbar. After careful selection, I selected this panoramic soundbar product launched by Bose in early 2024: Bose home entertainment speaker Ultra. (Photo source: Photographed by Lei Technology) Generally speaking, if we want to experience the "original" Dolby Atmos effect, we need to install a measured and calibrated surround sound + ceiling at home.
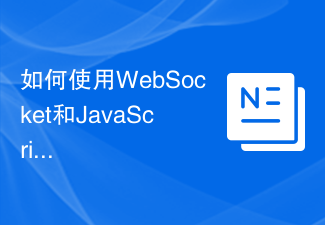
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
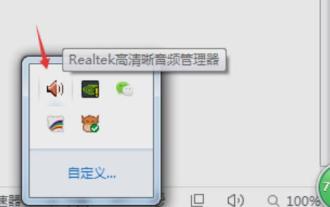
The win10 system is a system that can carry out various settings and adjustments. Today, the editor brings you the solution on how to set the microphone in realtek high-definition audio manager! If you are interested, come and take a look. How to set the microphone in realtek high-definition audio manager: 1. Find the "realtek high-definition audio manager" icon in the show hidden icons in the lower left corner of the desktop. 2. Click to enter the interface. The first thing you see is the "Speaker Page". In this interface, you can adjust the speaker sound through speaker configuration. 3. Next is the sound effect. You can choose the sound effect environment you want as well as "equalizer, pop, rock, club" and so on. 4. Next is the indoor sound quality correction. Indoor space correction can only correct the "
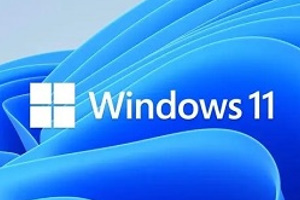
Some friends have reported that even if the sound in their computer is turned up to the maximum, the volume is still too low. At this time, the enhanced audio function in the system can be turned on. So how should it be done? Next, the editor will give you a detailed introduction. Friends who need it can take a look at how to turn on enhanced audio in Win11. How to open: 1. Right-click Start in the taskbar in the lower left corner and select "Settings" in the option list. 2. After entering the new interface, click the "Sound" option in "System". 3. Then click "All Sound Devices" in "Advanced". 4. Then select "Headphones" or "Speakers" in "Output Devices". 5. Finally, find "Enhanced Audio" and turn on the switch button on the right side of it.
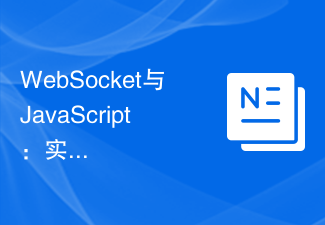
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
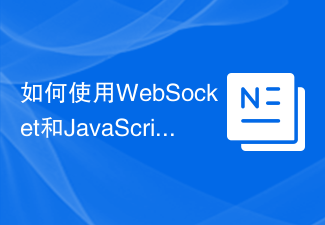
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.

If you encounter RealtekHD audio driver failure error code 0x00005b3 on Windows 11/10 PC, please refer to the following steps to resolve the issue. We'll guide you through troubleshooting and resolving the error. Error code 0x00005b3 may be caused by audio driver installation issues. It may be that the current driver is corrupted or partially uninstalled, affecting the installation of the new driver. This issue can also be caused by insufficient disk space or an audio driver that is incompatible with your Windows version. Installation of RealtekHD audio driver failed! ! [Error Code: 0x00005B3] If there is a problem with the Realtek Audio Driver Installation Wizard, continue reading
