How to implement message push and notification in uniapp application
Uniapp is a cross-platform development framework based on Vue.js that can be used to develop applications that run on multiple platforms at the same time. When implementing message push and notification functions, Uniapp provides some corresponding plug-ins and APIs. The following will introduce how to use these plug-ins and APIs to implement message push and notification functions.
1. Message push
To implement the message push function, we can use the uni-push plug-in provided by Uniapp. This plug-in is based on Tencent Cloud Push Service and can push messages on multiple platforms. The following are the specific steps:
- Register an account on the Tencent Cloud Developer Platform and create an application.
- Install the uni-push plug-in in the Uniapp project. You can install it through the following command:
npm install @dcloudio/uni-push
- In
main.js
of the Uniapp project Introduce the uni-push plug-in and initialize it:
import UniPush from '@dcloudio/uni-push' Vue.use(UniPush, { // 在腾讯云开发者平台上创建应用时生成的 Secret ID secretid: 'your_sceretid', // 在腾讯云开发者平台上创建应用时生成的 Secret Key secretkey: 'your_secretkey', // 在腾讯云开发者平台上创建应用时生成的 SDK App ID appid: 'your_appid', // 推送通知的图标路径(可选) icon: '/static/logo.png', // 推送通知的声音路径(可选) sound: '/static/sound.mp3', // 推送通知点击后要打开的页面路径(可选) page: '/pages/index' })
- Where push messages are required, call the
UniPush.pushMessage
method to send push messages:
UniPush.pushMessage({ title: '消息标题', content: '消息内容', tokens: ['token1', 'token2'], // 推送目标设备的token列表,可以是一个或多个token // 其他可选参数,如自定义字段等 })
- When the device receives a push message, you can listen to
getui in
onLaunchor
onShowin
App.vue. message
event to handle push messages:
export default { onLaunch(options) { uni.$on('getui.message', message => { // 处理推送消息 }) }, onShow(options) { uni.$on('getui.message', message => { // 处理推送消息 }) } }
2. Notification
To implement the notification function, we can use the uni-notify plug-in provided by Uniapp. This plug-in is based on the Notification API of HTML5 browsers and can display notifications in the browser. The following are the specific steps:
- Where notifications need to be displayed, call the
uni.$notify
method to display the notification. You can call it in a method in the component, or in Vue Called in the event callback function in the instance:
uni.$notify({ title: '通知标题', image: '/static/icon.png', content: '通知内容', onClick() { // 点击通知的回调函数 }, onClose() { // 关闭通知的回调函数 } })
- In the browser, when the user requests notification permission for the first time, the user needs to be asked whether to allow notifications. We can request notification permissions during the
created
life cycle of the Vue instance:
export default { created() { if (Notification.permission === 'default') { Notification.requestPermission() } } }
In this way, the user will be asked whether to allow notifications when opening the application.
The above are the specific steps to use Uniapp to implement message push and notification. By using the uni-push plug-in and uni-notify plug-in, we can easily implement these two functions. Of course, in actual development, it can also be customized and expanded according to specific needs. Hope this article is helpful to you.
The above is the detailed content of How to implement message push and notification in uniapp application. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
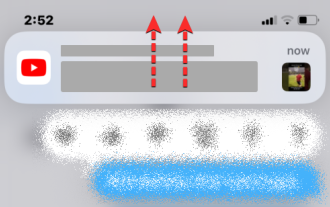
While notifications aren't the strongest suite on the iPhone, in recent iOS updates, Apple has fine-tuned how they appear. The new iOS version minimizes the visibility of alerts through Notification Center to ensure users have a better experience. In this article, we will help you clear notifications on iPhone in various different ways. How to turn off incoming notification banners on iPhone When you are on the Home screen or actively using an app, all notifications will appear as banners at the top unless you disable this feature. If you want to check the notification later without interrupting your current task, simply swipe the banner up to dismiss it. This will move the notifications you receive to Notification Center so you can
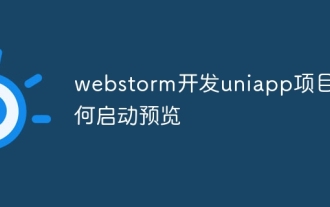
Steps to launch UniApp project preview in WebStorm: Install UniApp Development Tools plugin Connect to device settings WebSocket launch preview
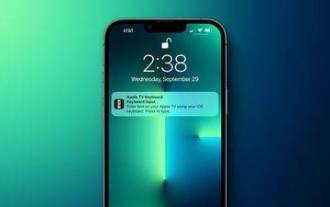
On AppleTV, if you don't want to use AppleTV Remote to enter text, you can type using a nearby iPhone or iPad. Whenever a text field appears on AppleTV, a notification will appear on your iPhone or iPad. After tapping the notification, you can use the on-screen keyboard on your iOS device to enter text on AppleTV. If you find these notifications annoying, you can disable them on your iPhone or iPad (if you have a few AppleTVs and kids at home, you'll know what we mean). If you're running iOS/iPadOS 15.1 or later, here's how to disable them. Launch on iPhone or iPad
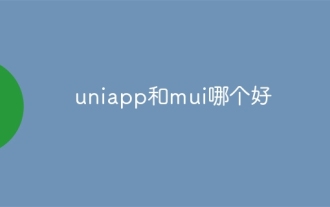
Generally speaking, uni-app is better when complex native functions are needed; MUI is better when simple or highly customized interfaces are needed. In addition, uni-app has: 1. Vue.js/JavaScript support; 2. Rich native components/API; 3. Good ecosystem. The disadvantages are: 1. Performance issues; 2. Difficulty in customizing the interface. MUI has: 1. Material Design support; 2. High flexibility; 3. Extensive component/theme library. The disadvantages are: 1. CSS dependency; 2. Does not provide native components; 3. Small ecosystem.
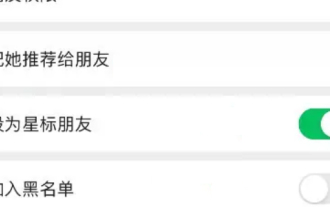
Business cards are a method that can be used to push friends in the software WeChat. Some users don’t know how to push friends’ business cards in WeChat. Just click on the friend’s personal page, select More to recommend them to friends and send them. This article is about WeChat push. The introduction of the friend’s business card method can tell you the specific content. The following is a detailed introduction, take a look! WeChat usage tutorial: How to push a friend’s business card on WeChat? Answer: Click on the friend’s personal page, select More to recommend them to friends and send them. Details: 1. Click on the friend you want to push a business card to. 2. Click the [More] option in the upper right corner. 3. Then click [Recommend TA to friends]. 4. Select the friend you want to send a business card to. 5. Click [Send].
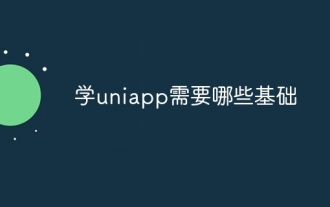
uniapp development requires the following foundations: front-end technology (HTML, CSS, JavaScript) mobile development knowledge (iOS and Android platforms) Node.js other foundations (version control tools, IDE, mobile development simulator or real machine debugging experience)
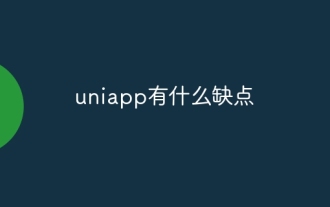
UniApp has many conveniences as a cross-platform development framework, but its shortcomings are also obvious: performance is limited by the hybrid development mode, resulting in poor opening speed, page rendering, and interactive response. The ecosystem is imperfect and there are few components and libraries in specific fields, which limits creativity and the realization of complex functions. Compatibility issues on different platforms are prone to style differences and inconsistent API support. The security mechanism of WebView is different from native applications, which may reduce application security. Application releases and updates that support multiple platforms at the same time require multiple compilations and packages, increasing development and maintenance costs.
