How to use JavaScript to achieve image lightbox effect?
How to use JavaScript to achieve the image lightbox effect?
With the development of social media and web design, the picture lightbox effect has become one of the common interactive special effects in many websites. Image lightbox is an effect that displays an enlarged image in the center of the screen by clicking on it. It not only improves the user experience, but also better displays the details of the image. In this article, we will learn how to use JavaScript to implement a simple image lightbox effect.
First, we need an HTML page to display images. Below is a basic HTML structure.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
|
In the above code, we created a container containing three pictures and added a class of "lightbox" to each picture. We also create a hidden div to display the enlarged image, with the id "lightbox". In this div, we added an empty image and a close button.
Next, we need to create some CSS styles to achieve the effect of the image lightbox. Create a file named style.css and add the following styles:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
|
In the above CSS style, we set the style of the container and image so that they can be displayed in the form of a grid on the page . We also define a style called "lightbox" that is used to adjust the layout and background color when the image is enlarged. Finally, we set the style for the enlarged image and the style for the close button.
Now, we can start writing JavaScript code. Create a file named script.js in the same directory as the HTML file and add the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
In the above JavaScript code, we first get all the files through the getElementsByClassName
method Image element with "lightbox" class. Next, we get the elements for the zoom image and the close button. Then, we use a loop to bind a click event to each image. When the user clicks on the image, the source address of the image is set to the address of the enlarged image, and the hidden style of the enlarged image is removed. Finally, we bind the click event of the close button and add a hidden style to enlarge the image when the user clicks the close button.
Now, we can open the HTML file in the browser, click on the image, and see the effect of the image being enlarged and displayed in the center of the page. When the close button is clicked, the enlarged image will be hidden.
The above is the sample code for using JavaScript to achieve the image lightbox effect. You can modify and expand it according to your needs to create more complex and diverse picture lightbox effects. I wish you success in achieving this!
The above is the detailed content of How to use JavaScript to achieve image lightbox effect?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




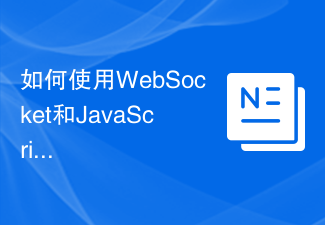
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
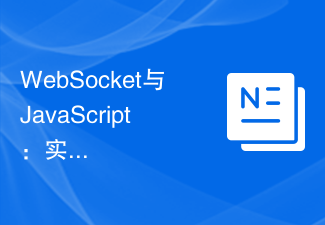
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
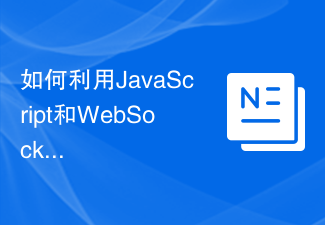
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
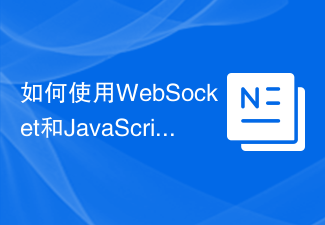
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
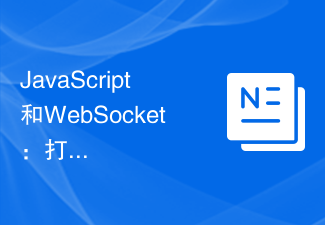
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
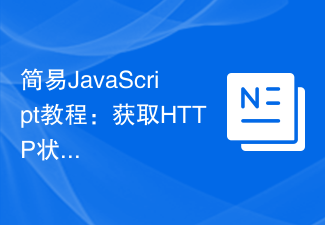
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
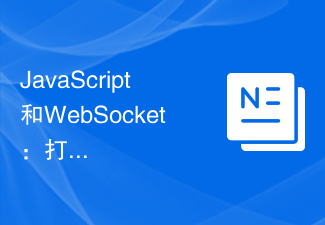
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
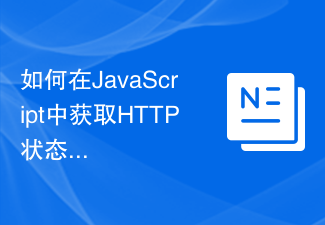
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
